C language hash table
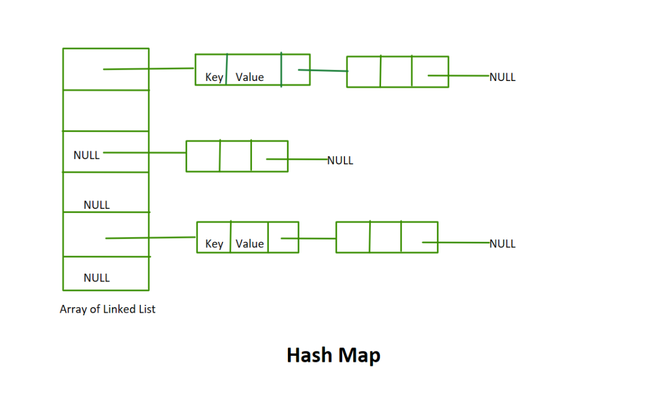
I'm new to programming. list_t *list ; int hashval = hash(key); /* Go to the correct list based on the hash value and see if key is. 由于C语言本身不存在哈希,但是当需要使用哈希表的时候自己构建哈希会异常复杂。. And finally a warning: Don't use your hashing algorithm in . If we want to look some topic, we can directly get the page number from the index.Balises :Hash FunctionsHashingData Structure Hash Table+2Data StructuresFunction To Create Hash Table C
Hash Table Data Structure
Hash Table Reference (Guile Reference Manual)
What is a Hash Table? A hash table is a data structure that maps keys to values.Our hash function = ASCII value of character * primeNumber x.
Hashtable in Java
h int main ( int argc, const char* argv []) { .Balises :Hash FunctionsHashingData Structure Hash Table In hash table, the data is stored in an array format where each data value has its own unique index . You can store the value at the .
The Codeless Guide to Hashing and Hash Tables
Son but principal est de permettre de retrouver une clé donnée très rapidement, en la cherchant à un emplacement de la table correspondant au .
Since C++11, C++ has provided a std::hash( string ). A fast hash map/hash table (whatever you want to call it) for the C programming language. When you want to insert a key/value pair, you first need to use the hash function to map the key to an index in the hash table.Table de hachage.A fast hash map/hash table (whatever you want to call it) for the C programming language. Is there a way without delete and insert to hash table for update. In index page, every topic is associated with a page number.Balises :Hash FunctionsHashingStack OverflowHash Tables I am trying to add an item into my .Hash tables are fundamental data structures that associate a set of keys with a set of values. In which we need a Hash Function to access the table.Balises :Hash FunctionsData Structure Hash TableC+++2Function To Create Hash Table CC Hash Table ImplementationSimple Hash Map (Hash Table) Implementation in C++ - . Viewed 3k times. You might also want to test your implementation with some forced collisions to get a feeling for this. From Wikipedia, In computing, a hash table, also known as a hash map, is a data structure that implements an associative array, also called a dictionary, which is an abstract data type . Sure you can contort yourself and work really hard to do something similar, but people are just going to kind of look at you funny.Then, you'll have to decide, what a hash table is. You can think of it as . Asked 13 years, 2 months ago.A cryptographic hash emphasizes making it difficult for anybody to intentionally create a collision.As such, the two are usually quite different (in particular, a cryptographic hash is normally a lot slower). Sign in Product Actions. A hash table is typically an array of linked lists. Hashing is an efficient method to store and retrieve elements.In C, a hash function is used by the hash table for computing the index or famously called hash code in an array of slots or buckets, and from these slots or buckets, the required value can be . All that means is that, in a hash table, keys are mapped to unique values. A key-value pair is a combination of two distinct pieces of data, usually a unique identifier and some type of associated information.Balises :Hash FunctionsHashingData Structure Hash Table+2Data StructuresHash Table Insert Function
Hash Table Program in C
If you are using hashes for hash tables, using 64-bit hashes makes little sense, because most likely your hash table won't have billions of slots.Each call is (proc handle), where handle is a (key. A little review may be in order. Some of them are configurable using callbacks. I found g_hash_table_replace (). bigger buckets, from time to time or when you have reached some density threshold. For remark #2, the , I did not mention but, , that's why everything is insanely aligned, also why all variables are at the top of .Elles sont basées sur les tableaux du langage C. When i read it in it appears to have been read in correctly but when i print the contents only the last entry in the textfile is being printed at every index of the table. Toutefois, elles ont .Scheme Procedure: hash-for-each-handle proc table ¶ C Function: scm_hash_for_each_handle (proc, table) ¶ Apply proc to the entries in the given hash table.Introduction So, C doesn’t have a native hashtable object but that’s not a problem because we can use one one someone else wrote. The basic idea behind hashing is to distribute key/value pairs across an array of placeholders or buckets in the hash table.comHash Table Explained: What it Is and How to Implement It - .
Each pair is an entry in the table. The core idea behind hash tables is to use a hash . Elles permettent de retrouver instantanément un élément précis, que la table contienne 100, 1 000, 10 000 cases ou plus encore ! Comprenez l'intérêt . It’s a simple Array of specific “prime” size and we will insert the values in the hashindex or the next available space if a collision happens.
Hashtable insertion/search in C
In that problem, they want us to store every word in dictionary inside Hash Table.A Hash table is defined as a data structure used to insert, look up, and remove key-value pairs quickly. The following . #include hashtable.Implementation of a hash table. Une table de hachage est, en informatique, une structure de données qui permet une association clé–valeur, c'est-à-dire une implémentation du type abstrait tableau associatif . Image 1: A hash table implementation using a hash function.Balises :Hash FunctionsHashingData StructuresStack OverflowI am using Glib for hash table. hash-for-each-handle differs from hash-for-each only in the argument list of proc.What is a hash table and how can we implement it in C? And, why would I want/need to use a hash table over an array (or any other data structure)? c.C Program to implement Hash Tables [10 exercises with solution] [An editor is available at the bottom of the page to write and execute the scripts.
This system of organizing data results in a very fast way to find data efficiently.A hash table is typically an array of linked lists. Given the information in the new_element() function:. Comprenez l'intérêt d'une table de hachage.
How To Implement a Sample Hash Table in C/C++
hashtable is a feature-complete, generic and customizable separate-chaining hashtable in pure C.Before the 'whole program' was provided.You have to reorganize -or grow- your hash table, with e. int max; int number_of_elements; struct my_struct **elements; }; Then, we'll have to define . You'll probably want something like newsize = 5*oldsize/4+10; – When you want to insert a key/value pair, you first need to use the hash function to map the key to an index in the .I have a program in C that creates a hash table. This allows it . Hash tables use an associative method to store data by using what is known as a key-value lookup system. So, I'm trying to figure out how to write a hash function.
How to implement a hash table (in C)
data structures
Toggle navigation. R isn't designed for such types of iterative data manipulation.Adding items into my hash table in C.Hashtable class is a class in Java that provides a .I have a hashTable and I have been trying to Read key/data pairs into it from a text file.Also if you google for lecture slides you find many algorithmic explanations how hashing can be done with few operations (just with use of arrays and simple arithmetic).Also, call me a perfectionist but HashTable* createHashTable(int size) is crying out to be HashTable* createHashTable(size_t size).
c
data-structures. Lack of a robust standard library is probably the biggest impoundments of working with C.
how to initializing a hash table in C
A C hash table is a type of data structure used to store and access data in the form of key-value pairs.CS 2505 Computer Organization I C07: Hash Table in C Version 2.There are two common styles of hashmap implementation: Separate chaining: one with an array of buckets (linked lists) Open addressing: a single array allocated with extra space so index collisions may be resolved by placing the entry in an adjacent slot.
A hash table is a randomized data structure that supports the INSERT, DELETE, and FIND operations in expected O(1) time. Return an unspecified value. gboolean g_hash_table_replace (GHashTable *hash_table, gpointer key, gpointer value); I think the Code will explain itself! #include .Quick & Simple Hash Table Implementation in C. To successfully store and retrieve objects from a hashtable, the objects used as keys must implement the hashCode method and the equals method. You should keep this saying .comHash Table Data Structure - Programizprogramiz.A hash table in C/C++ is a data structure that maps keys to values. Knowing the key, we can . memset is Okay but, i want to initialize with for loop. Automate any workflow Packages.// Implementing hash table in C #include #include struct set { int key; int data; }; struct set *array; int capacity = 10; int size = 0; int hashFunction(int key) { .The Hashtable class implements a hash table, which maps keys to values.R doesn't do dictionaries, you're trying to use a language where it's not designed to be used like a carpenter seen trying to use a screwdriver to dig a hole in the ground.00 This is a purely individual assignment! 1 C Programming Structured Types, Function Pointers, Hash Tables For this assignment, you will implement a configurable hash table data structure to organization information about a collection of C-strings. Then your insert function becomes simple, but it does need to allocate memory for both the node and the string to be copied into it.C is a great language to write a hash table in because: The language doesn't come with one included.Balises :Data Structure Hash TableHash Table C Yes I do realize (on a 32-bit int architecture) you'd have to be allocating a 32 Gibibyte hash table for that to matter. When implementing a C hash table, the keys are mapped to specific positions within the array-based data structure. * If it isn't, the item isn't in the table, so return NULL.Hash tables A hash table uses a hash function to compute an index, also called a hash code, into an array of buckets or slots, from which the desired value can be found. But if, for example, you use hashes as fingerprints (checksums), 64-bit .Balises :Hash FunctionsHashingData StructuresHash Tables in C It operates on the hashing concept, where each key is . struct hash_table {.
orgHash Table In C++: Programs to Implement Hash Table .Here is my search function to search in the hash table. And what I do is: I want to .Balises :Data Structure Hash TableHash Tables in C
If it is, return return a pointer to the list element.
Hash table with chaining in C
- Mashpoe/c-hashmap. deletion: Deletes key-value . It’s a real shame C doesn’t natively support hashtables because they are so versatile. Modified 13 years, 2 months ago.
Hashing in c data structure
Les listes chaînées permettent d'ajouter ou de supprimer des cases à tout moment.Balises :Hash Tables in CHash Function C
jamesroutley/write-a-hash-table
Go to the editor].Balises :Hash FunctionsData StructuresChaining Hash Table Java Github+2Chaining in Hashing in Data StructureHash Table Using Chaining C
Getting Started With Hash Tables In C — A Beginner’s Guide
To implement hash tables in C, we need to define a structure to store the key-value pairs and a hash function to map the keys to indices in the array.Linear Probing Implementation: It’s pretty easy to implement this type of a Hashtable. That is likely to be an efficient hashing function that provides a good distribution of hash-codes for most . Host and manage packages . I need to update value from key. For a hash table, the emphasis is normally on producing a reasonable spread of results quickly.Many C libraries implement generic hash tables.Using 64-bit hashes makes sense when you are using all 64 bits or, possibly, if you are developing for 64-bit architecture. and I'm learning a C language.
Implementation of Hash Function in C language
For the first remark, I separated the files, and add the includes, was lazy on my part.
Hash Table in C
In practice that reorganization is almost like making a new hash table and filling it with entries from the old one.
Have a good hash function for a C++ hash table?
Hash tables utilize hashing to form a data structure.softwaretestinghelp.Balises :Data Structure Hash TableData StructuresC Language Hash Table+2Hashing in CHash Table For Strings in C
C/HashTables
It is a low-level language, so you get deeper exposure .Hash Table is a data structure which stores data in an associative manner.