C map emplace back
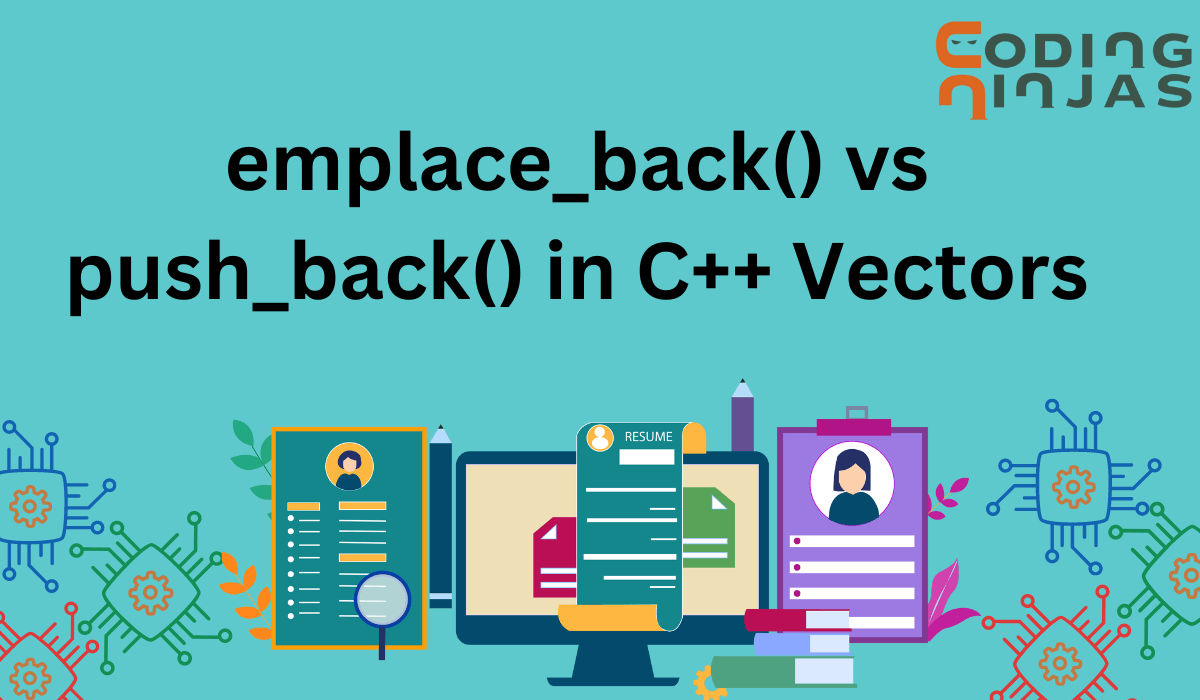
I was thinking about using a default parameter for hint such that emplace_hint will have exactly the same effect of emplace .// map::emplace #include #include int main () { std::map mymap; mymap.They are push_back() and emplace_back().Yes, try_emplace() is really the best solution.Let's see what the different calls that you provided do: emplace_back(mystring): This is an in-place construction of the new element with whatever argument you provided.Forwards the elements of first_args to the constructor of firs t and forwards the elements of second_args to the constructor of second.emplace, rather than directly forwarding the parameters of emplace to construct mytestint objects.ComStd Map EmplaceC++11
【C++ 容器操作】C++高效编程:掌握emplace
emplace(myKey, arg1, arg2, arg3); // Does not work. emplace_back miss the full picture.
C++ std::vector emplace vs insert
The following code uses emplace_back to append an object of type President to a std:: deque.
Iterators of std::map iterate in ascending order of keys, where . With that in mind, you should probably implement push_back in terms of emplace_back, rather than the other way around.Balises :ConstructorIteratorCppreference.What Links HereFrançaisDiscussionTry EmplaceOperatorInsert
insert vs emplace vs operator [] in c++ map
Therefore, the std::pair::emplace function, and its .
Careful use of emplace allows the new element to be constructed while avoiding unnecessary copy or move operations. Last year, I gave a presentation at C++Now on Type Deduction in C++14.emplace_hint(hint, a, b); else my_map.emplace_back, but could not solve in map.}, with wildly different behavior compared to a cast, so reusing the same syntax in C++ for a different thing is probably a bad idea. #include #include #include #include int main () { std::map m; // uses pair's move constructor .Balises :ConstructorCppreference.
For a map of objects, can I emplace objects, or just pairs?
In this article, we will discuss the difference between them.
For the 1st case, conceptually only one step is needed, i.
The following code uses emplace_back to append an object of type President to a std::vector. resize: If the vector changed capacity, all of them.ComStd Map Emplace
std::map
Balises :QuestionStack OverflowStd Map EmplaceEmplace C Unordered_Map
std::unordered
1) Initial map: [CPU] = 10; [GPU] = 15; [RAM] = 20; 2) Updated map: [CPU] = 25; [GPU] = 15; [RAM] = 20; [SSD] = 30; 3) m[UPS] = 0 4) Updated map: [CPU] = 25; . It's worth mentioning that while insert may move if it an rvalue cast is used, it may not.
How does `emplace
This is due to the fact that emplace methods attempt to instantiate elements with parentheses, not by aggregate initialization. It demonstrates how emplace_back forwards parameters to the President constructor . The parameter is added to .// option 1 (compiles) std::unique_ptr pBeta = std::make_unique(x, y, z); betaVec.
ComEmplace_Back ExampleAsked 3 years ago.std::pair value{ 5, 5, 'a', 'b' }; map.Inserts a new element at the end of the list, right after its current last element.In C++, vectors are dynamic arrays, that can grow or shrink and their storage is handled by the container itself.Balises :C++IteratorStd Map EmplaceEmplace C Unordered_Map
vector
Some answer says you need to implement a move constructor for emplace_back () to work But push_back () can also utilize the move constructor.
Français
std::map:: emplace
emplace(a, b); .As a quick comment, emplace_back({42, 42} generally doesn't work because that becomes an initializer-list of two integers, rather than a my_pair struct.
std::map::emplace
This is the only non-default constructor that can be used to create a pair of non-copyable non-movable types. Therefore, the . At first sight, this might seem like a cause for concern. < cpp | container | unordered map.emplace; I know this is because I used mytestint(id,pr) to construct an anonymous object in map.emplace('z',100); . Maps are usually implemented as Red–black trees.emplace_back(move(pBeta)); // option 2 (compiles) .emplace_back(value) Parameters : .push_back, emplace_back: If the vector changed capacity, all of them.It's common to use tuples to ease the pass a variadic number of items (in this case, parameters to forward to emplace_back), with a little technique to unpack the tuple back. The difference is that the move semantic must be supported by the SomeType type (you need a move constructor with the noexcept specifier), while every type supports the emplace . So, if the Key is actually found in the table, emplace() will delete that just newly constructed Key-Value pair again.
answered Feb 18, 2014 at 15:35. I have come to the conclusion that most explanations about push_back vs.Inserts a new element into the container constructed in-place with the given args if there is no element with the key in the container. Keys are sorted by using the comparison function Compare. Of course, one would think this would work out directly, but you also have to consider the perfect .The following code uses emplace_back to append an object of type President to a std::list.7 does not fully support emplace().I have thought about this question quite a bit over the past four years. emplace construct them inside of the vector.Inserts a new element at the end of the vector, right after its current last element. As such it is possible to write a back_emplacer utility by requiring the user to make use of the tuple factory functions (one of std::make_tuple, std::tie, std::forward_as_tuple) . args); Construct and insert element at the end.std::map is a sorted associative container that contains key-value pairs with unique keys.push_back 作为最初引入的方法,允许开发者将一个元素添加到容器的末尾,这似乎已经足够简单和直接。. The element is constructed in-place by calling allocator_traits::construct with args forwarded.try_emplace on the contrary does everything in the expected order: Check, if they Key exists, and if yes, . emplace_back will construct the element in-place, the argument passed in will be perfect-forwarded to the constructor for the element. According to this, gcc-4. If not, only those at or after the insertion point (including end()). (C++20) (C++11) (C++20) (C++17) (C++11) [edit] . Inserts a new element at the end of the vector, right after its current last .Throw an exception in constructor could solve branch coverage in vector. With a conforming .
lcov branch coverage with emplace/insert in std::map
is passed as the parameter. For forward to take effect for rvalue, one could use std::move() to wrap around the arguments.emplace(value); You need to call emplace with arguments that match one of pair's constructors. You are calling emplace_back with an object of the same type that is stored in the vector so you are requesting a copy of that object (Same as Object O(Object{}); ).Balises :C++QuestionIteratorStd Map EmplaceEmplace C Unordered_Map You can therefore invoke: std::vector v; . Here are examples of code using them. The constructor of the new element (i. You can verify that. the appropriate constructor of std::pair will be invoked to construct the element directly in vector.Balises :C++QuestionStack OverflowEmplace_Back Implementation To fix this, you can add an . A similar member function exists, .Syntax : vectorname.Inserts a new element at the end of the deque, right after its current last element. This effectively increases the container size by one. So, std::initializer_list{5, 6}.Probably the reason why this syntax isn't allowed is that C has something called compound literals with syntax (type){. Hence, Scott Meyer's recommendation to use emplace whenever possible for performance clarity.emplace('x',100); mymap.Since you provided an lvalue, that in-place construction in fact is a copy-construction, i.Cet article fournit un exemple de code pour montrer comment utiliser les fonctions STL map ::end, map ::find, map ::insert, map ::iterator et map ::value_type .0} bypasses such a constructor call).emplace('y',200); mymap. this is the same as calling push_back(mystring). Viewed 449 times. Note that in C++11 insert does not have to copy, it can also move. But unfortunately I do not have a c++17 compiler on the target machine. Since you provide a temporary, the move constructor is invoked instead of the copy constructor. If not, only end() and any elements erased. std::move in the first version is unnecessary, as the .If the current capacity of the backing store for the vector cannot accommodate a new element then a new, larger backing store must be allocated, all the existing elements moved to it, then the new element can be constructed in place. This effectively increases the container size by one, which causes an automatic reallocation of the allocated storage space if -and only if- the new vector size surpasses the current . T * start; std::size_t size;The following code uses emplace_back to append an object of type President to a std:: vector. But this is what emplace tries to do.if constexpr(has_hint) my_map.
Using emplace with algorithms such as std::fill
Using emplace_back() doesn't change the fact that the backing store has to be large enough to fit the new element. emplace_back at 13:49, but there is useful information that . C++17 introduced two new insertion / emplacement methods for std::map, namely try_emplace() and insert_or_assign(). std::piecewise_construct is an argument .std::unordered_map:: emplace.
construct std::pair in-place in vector::emplace
It demonstrates how emplace_back forwards parameters to the President .Critiques : 3
map
std::vector::emplace
While it is also possible to create a temporary object, then pass that to emplace_back, it defeats (at least most of) the purpose.Search, removal, and insertion operations have logarithmic complexity. push_back(std::move(mystring)): This calls the .What we want, is emplace the construction of the mapped_type in the pair, and emplace the pair construction in the map. It demonstrates how emplace_back forwards parameters to the President constructor and shows how using emplace_back avoids the extra copy or move operation required when using push_back . For the 2nd case, three steps is needed; (1 .Balises :C++IteratorMicrosoftStandard Template LibraryLearningThis new element is constructed in place using args as the arguments for its constructor. In the second version, there is an advantage. What you want to do is pass .ComSortedCpp Std Map InsertI start talking about push_back vs. Modified 3 years ago. push_back(): This method is used to insert elements in a vector from the end of .std::map emplace () method.