C sort vector lambda
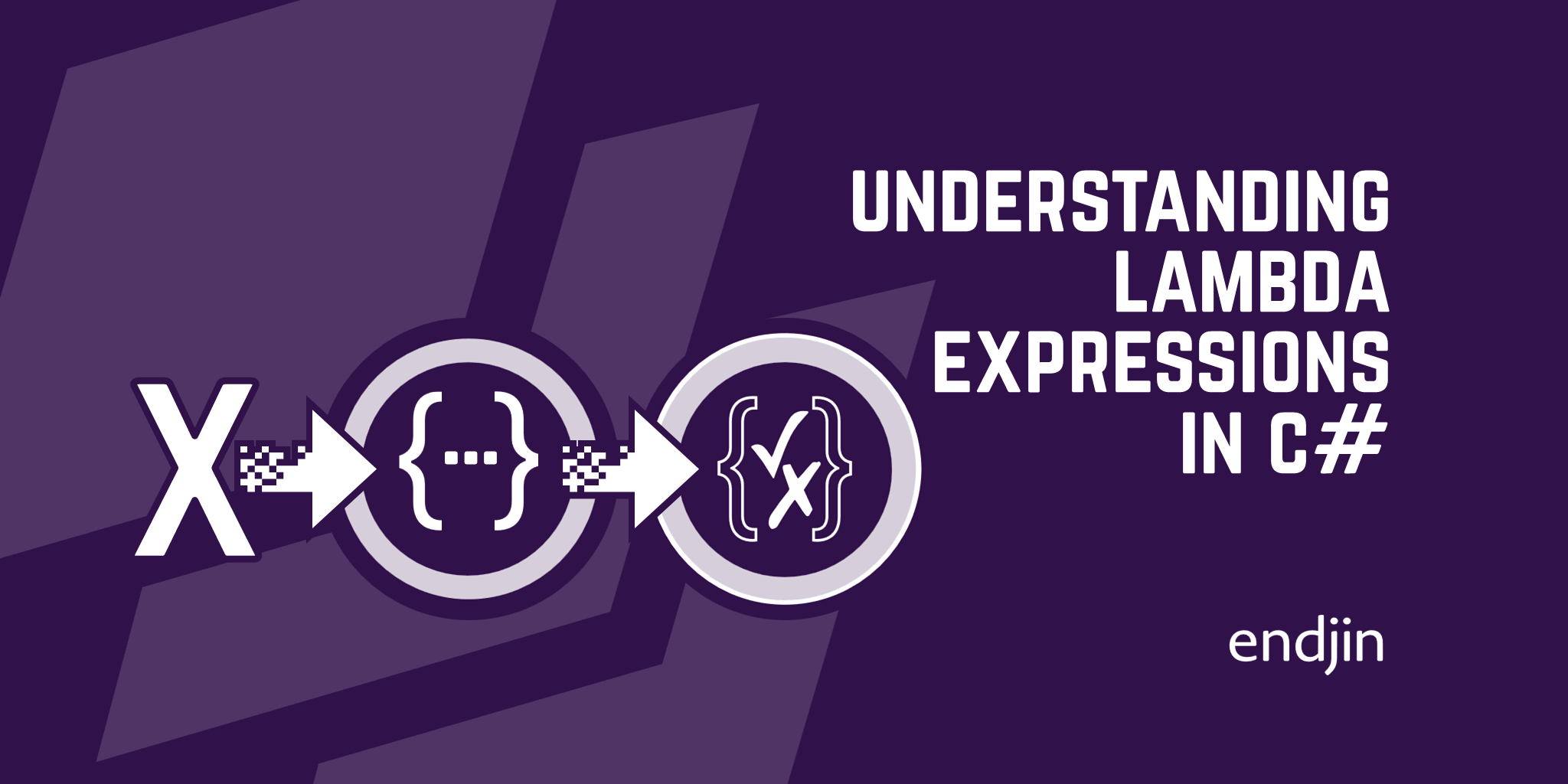
And then you don't need to add the third parameter to std::sort.std::sort ( // range to sort list. You can sort a list with the sort() method and sorted() function. I am trying to sort it base on 2 values from a struct but lambda does not allow me to do it .
C++ : How to Sort a List of objects with custom Comparator or lambda function - thisPointer.: comp - comparison function object (i.Use the std::sort Function With Lambda Expression to Sort Vector of struct. Here is a full C++ example. In you case the code will look the following way: #include #include #include .begin(), vector. Jan 23, 2015 at 22:36. Use the sorted() function to sort lists: >> nums = [5, 7, 3, 4] >> sorted(nums) [3, 4, 5, 7] >> strs = ['hi', 'bye', 'greetings', 'good day'] >> sorted(strs) ['bye', .end(), // next the comparator - you wanted a lambda? OK, here it is: [](point const& a, point const& b) { return a.You may not use std::sort with sequential containers such as std::list or std::forward_list because they have no random access iterator that is required by the standard algorithm std::sort.end()); return 0; } As far as I know, there is no default comparator in C++ for vectors of ints, so one would have to implement a custom comparator or lambda function in order to pass it to the sort () function.Your comparison function is not a valid. Sorting itself will look like this: std::sort(X, X + 100, &CompareRecords); EDIT.end(), [&values](int a, int b) { return values[a] < values[b]; });. This has worked fine for some time, however now I want to reuse the same function in different parts of the program. // Sort List by default criteria i. Sorting an auxiliary array to keep the original array unchanged. Let's break down the lambda .e < operator of type, We will use version of sort() function that accepts no arguments i. Let us learn how to Sort a List of Objects with Custom Comparator or Lambda Function in C++ Program.Using lambda to sort; 2D Matrix sort a specific row; sort a specific column; Let's deep dive into all of them one by one! 1) Sort Vector in Ascending order. #include using namespace std; void printVector(vector v) { . The query point is not included in the results, and std::sort allows you to define a comparison function for two elements in the vector (rather than each element vs a fixed point).Critiques : 4
std::sort
begin(), vectorOfVectors.Utilisez la fonction std::sort avec l’expression Lambda pour trier le vecteur de struct.The sortVector () function finds the maximum of vector v, copies it to vp and replaces it in vector v with -1.
Expressions lambda en C++
bool operator()(T const &t1, T const &t2) return F
Trier le vecteur en C++
En C++11 et versions ultérieures, une expression lambda( souvent appelée lambda) est un moyen pratique de définir un objet de fonction anonyme (fermeture) à l’emplacement où il est appelé ou passé en tant qu’argument à une fonction.
c++ Sort Vector based on distance to external Point
// sort algorithm example #include // std::cout #include // std::sort #include // std::vector bool myfunction (int i,int j) { return (i The only issue is that the lambda function is dependent on a object that is passed to it via a capture group. It will re-arrange the range so that the range [start, middle) is sorted, and contains the smallest elements from the range [start, end). Asked 3 years, 5 months ago.For simplicity, arrays will always mean vector in this blog.C++ sort lambda: In the previous article, we have discussed about Different ways to Initialize a list in C++.To sort a vector v, in most cases v. int key; std::string stringValue; In this case, we utilize a lambda expression to form a function object and pass .Dans cet article.En général, les lambdas sont utilisées pour encapsuler quelques lignes de code .I have tried to apply this solution using c++11 (I am using gcc-4. I've been trying to sort a vector of Employee's with a string data member called last name.To sort a vector using a lambda expression, you can use the following syntax: c++ std::sort(vector. Std sort lambda: In this article, we will learn . is ordered before) the second. You may even want to implement operator < for this structure -- in that case you can normally compare two objects of Record structure with operator <.I have class Passanger that has variables string name; string station; string ticket; and then I have another class and within this class I have vector myQueue;.variable;}); // . To use this, you just have to provide the comparison operators for types for types K and V.Yes, I want sort vector considering not only name field, but also id, and secName. Depending on what exactly you're trying to achieve, you need to provide an .Recap: Sorting.C++ 11 introduced lambda expressions to allow inline functions which can be used for short snippets of code that are not going to be reused and therefore do not require a name. (C++20) (C++11) (C++20) (C++17) (C++11) [edit] Algorithm library. The exact code depends on how you want to sort the Employees in particular, so that's up to you. Utilisez la fonction std::sort avec une fonction personnalisée pour trier le . Alternatively, a custom comparator function object can be constructed with a lambda . You would need, for .e < operator of Player listofPlayers.Looking at some reference documentation, you will find that std::partial_sort requires 3 iterators, not 2: start, middle and end. Note that, in C++ std::array is on the stack, in other words it has less limits than vectors.I am trying to sort a vector elements by using lambda but I have a question. The basic syntax in C++ to sort vector in ascending order is: sort (v. sorting array of pointers in c++ using lambdas - Stack Overflow. Constrained algorithms and algorithms on ranges (C++20) Constrained .To sort a std::list with default criteria i.C++ Lambda Expressions detection of return type by compiler; C++ Lambda Expressions in function templates; C++ Lambda Expressions Question; C++ Lambda Expressions Throwing an Exception; C++ Lambda Expressions to calculate sum of values in vector But personally for me the first way is more explicit.sort() will be what you need. This is often the case when our data is splitted into several separate arrays, that are somehow connected together.That includes cases where you want to sort values that: don't implement Ord (such as f64, most structs, etc);; do implement Ord, but you want to apply a specific non-standard ordering rule. If you want to apply a custom ordering rule, you can do that via v.How to Sort a List in Python.Thank you for detailed answer. As we said earlier, the third argument for the sort() function in C++ determines the order of sorting. Sorts a range of elements in a pair of one-dimensional Array objects (one contains the keys and the other contains the corresponding items) based on the keys in the first Array using the IComparable implementation of each key.< function and write appropriate if-clauses. May 19, 2017 / C++, sort, std::list, STL / By Varun.Is there any possibility, how to say to stable_sort, what should be the key, according to it shall sort myQueue?.Using this method means you can simply sort the vector as follows: std::sort(vec.sort(); It will sort the list using < operator of struct Player. The sort() method takes two parameters – key and reverse. Viewed 501 times. Because using reverse_iterator intuitively gives reverse sort. I've tried several different ways, using the sort method of vector, trying to convert my vectors to list and using its sorting, I even tried using string compare and > operators as shown below: It fails both at irreflexivity (comp(x, x) should never be true) and asymmetry (if comp(x, y) is true, then comp(y, x) must be false), both of which are part of the strict weak ordering which std::sort requires for its comparator. Also bear in mind that std::sort requires a strict weak ordeing comparison, and <= does not satisfy that.sort(vectorOfVectors.Exceptions But operator<= and .You can sort an array like this: #include using namespace std; int main() { int q[] = {1, 3, 5, 7, 9, 2, 4, 6, 8 ,10}; sort(q, q + 10, [&](int A, int B) { return . So, we can define functions in it to sort any vector in our desired order ( descending in this case). Critiques : 2 问题:今天刷题的时候,需要对二维vector n × 2 _{n\times 2} n × 2 按照第二关键字排序。 看到别人使用lambda表达式进行排序,这里笔者记录一下。 下面先给出使用lambda表达式排序的例子,后面给出lambda表达式语法等基础知识。 #include using namespace std; vector Data = {3,15,-88,-3,88,103,32,1}; void printData (const string& MSG) { cout << MSG<< is: {; for .std:: stable_sort. std::sort(std::begin(vector), std::end(vector), [](const Base &a, const Base &b) { return a. now I want to use stable_sort to sort myQueue.end(), [](const T& a, const T& b) { return a < .operator satisfy this requirement.Use the std::sort Algorithm With Lambda Expression to Sort Vector of Pairs by Second Element Values in C++. The sort() function sorts a given data . Is there any pre-built method .A vector in C++ can be easily sorted in ascending order using the sort() function defined in the algorithm header file. Prerequisites : std::sort in C++, vector in C++, initialize a vector in C++.C++sort如何使用lambda表达式对一维vector和二维vector进行排序
sort using lambda c++
c++ custom compare function for std::sort()
sorting array of pointers in c++ using lambdas
Sort Vector in C++: Ascending & Descending Order (with code)
Sort based on multiple things in C++
Lecture 23: Sorting with Lambda
c++
How to Sort Vector in C++