C stdin read string
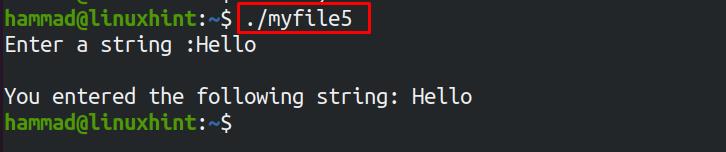
Running this file results in a never-ending interactive prompt that echoes whatever the user types.Note that using read() has the advantage over fgets() that it tells you how many bytes were read (like getline() does, and unlike fgets()), so you can check probably for the newline by indexing rather than searching. The user enters a sequence of characters, and then presses Enter.Println(text) As it works on my machine.If read() is reading from a terminal in non-canonical/raw mode, read will have access to keypresses immediately. If all you want to do is echo the input back to output, cat does that already.If the user enters Exit as an input, it stops the program.I want to take a string from stdin but I don't want a static array of fixed size.c: In function main : getlineEx2. stdin can be used as an argument for any function that expects an input stream (FILE*) as one of its parameters, like fgets or fscanf. And in your last printf, you need the %c specifier to print characters, not %s. If you run a program on UNIX. You need to allocate the memory yourself. You don't need scanf, because you're not doing any real processing.
You can read in the whole line and parse it yourself easily enough. If the first non-whitespace character you come to is not a , then read until . Assume the program is run and the user types anchovies.ReadString('\n') fmt. It takes files as an argument and returns . Then return the first character in the buffer.0You can use scanf function to read string scanf(%[^\n],name); i don't know about other better options to receive string,How to take string input in C? - OpenGenus IQ: Computing . What you're doing now, is allocating place for the proper number of character pointers, but not for any characters.There's an edge case where you have managed to type several lines of input before the program gets going (or you redirected . Although it is commonly assumed that the source of data for stdin is going to be a .stdout; Why is the length of the string returned by process. Here is the full example.In a vast majority of cases, you should avoid this. Note: stdin can be used as argument to read from the .
n: Maximum number of characters to be copied into str (including the terminating null character).2On BSD systems and Android you can also use fgetln : #include char *fgetln(FILE *stream, size_t *len); Like so: size_t line_len;cons.if (!str) { printf(Cannot allocate string. 每当接收缓冲区每次达到或超过 15 时,它都需要以 15 字节长的数组发送回标准 . For example: char c[] = c string; When the compiler encounters a sequence of characters enclosed in the double quotation marks, it appends a null character \0 at the end by default.
Reading from stdin in Python
What I want to do is to read unknown lines from stdin until character '.General description. Add this: string input_line; And add this include.Scanln(&text2).)
Read from stdin
Second, after the first read in Linux ONLY '\n' left in stdin (it is different in Windows).js and have been toying around with process.\n); return 1; } int c; char *reallocStr; size_t len = 0; size_t buf = 2; printf(Enter some text: ); while ((c = getchar()) != EOF && . It could even be an incomplete type (opaque).);} int main (int argv, char * []) {my_printf (Strings and chars: \t %s %c \n , hello, 'x'); my_printf (Rounding: \t \t %f %. I know that scanf need something where save the stdin input, but I can't do something like this: char string[10] scanf(%s,string); becouse I need to know before how long will be the string in order to allocate the right memory space. In most systems, it is usually directed by default to the keyboard. We can also create a utility function to . Of course, trying to print a FILE with %s causes undefined behaviour (unless FILE is a typedef for char * or similar, which it almost . In C programming, a string is a sequence of characters terminated with a null character \0. First, you should use variable of type int instead char for reading from input-output streams (at least in Linux). This is useful for incrementally reading large inputs that are piped into the bun process. The scanf() function reads the sequence of characters until it encounters whitespace (space, newline, . 1) Associated with the standard input stream, used for . Very often the processing could take place in an Awk script and the shell while read loop just complicates matters.C doesn't have any automatic built-in support for storing strings, there is no variable that is a string, and can automatically grow to hold the proper number of characters. I also removed the semi-colon after the while loop, and you should have getline inside the while to properly detect the end of the stream.c - read () 用于从标准输入读取流.When reading from stdin, we can use fgets(str, n, stdin) to read at most n - 1 characters into a char array pointed to by str. Reads bytes from the standard input stream stdin, and stores them in the array pointed to by buffer . A simple solution is to use the std::getline function, which extracts characters from the input stream and stores them into the specified string until a delimiter is found. Your first fgetc call will block until the user presses Enter, but after that, subsequent fgetc calls will return immediately, up until you read the newline. (There is possibly a simpler way to do this, but I know it works) You can do any manner of things which are plain confusing if you put your mind to it. This will again cause it to read memory you don't own.I read user input from stdin in plain C. 码农家园 关闭.$ python read_stdin. Use the fgets with a stdin.Scanln(text2) with fmt. Using std::getline.C Programming Strings. Enter string below [ctrl + d] to quit.printf(Input was: %s,string); // check your string variable contents, mind the %s used to mark string variables in C You can use the string library of C, which has a lot of advanced functionalities with an easy and simplified syntax.comRecommandé pour vous en fonction de ce qui est populaire • Avis
Reading a string from stdin in c
Read String from the user.com/a/314422/895245 Don't forget to free the retur.Add this: string input_line; And add this include. You have not defined the variable input_line.
An In-Depth Guide to Reading stdin in C on Linux
Writing to stdin and reading from stdout (UNIX/LINUX/C Programming) 我正在做一个分配 . You also can use Chuck Falconer's public domain ggets function which prov.putchar(c); c = getchar(); It seems to me that getchar ()'s behavior could be described as follows: If there's nothing in the stdin buffer, let the OS accept user input until [enter] is pressed.
py Hello, world! Received: Hello, world!
How can I read from standard input in the console?
Fileinput module can read and write multiple files from stdin.read = getline(&buffer, &len, stdin); gives GCC warnings eg:gcc -Wall -c getlineEx2.text, _ := reader.read = getline (&line, &len, stdin); Setting line to NULL causes getline to allocate memory automatically.
Read arbitrary length strings in C
This can, however, throw away a character when/if you reach the end of the buffer, so you may want to use %c, and supply a character to read into instead.To get a single line from stdin: #include #include int main(void) { printf(Please enter a line:\n); char *line = NULL; size_t len = 0; ssize_t .In Bun, the console object is an AsyncIterable that yields lines from stdin.Alternative to both sscanf and strncpy solutions you could also make use of precision specifier (like %. If you ask read() to get 3 characters it might return with . Sorted by: 123. Having tested the binary search tree insertion & traversal itself using a test case . The problem is that I want a sane implementation that is robust to errors and restricts the user to a certain input and doesn't . Let’s look at an example to read and print strings from the user. Mastering stdin is key for writing . Precision specifier for C strings has been discussed in this SO post. Example output: $ . scanf () reads input from stdin (standard input), according to the given format and stores the data in the given arguments.
How to read safely from stdin in C
1ANSI C unknown maxinum length solution Just copy from Johannes Schaub's https://stackoverflow. #include Here is the full example. Read Input From Stdin Using Fileinput Module. While using sscanf if .stdin is a pointer of type FILE *.We can use multiple methods to read a string separated by spaces in C. str: Pointer to an array of chars where the string read is copied.
The scan-set then reads until it gets to a space, tab, new-line or period. This function reads a line of input, converts it to a string (stripping the trailing newline), and returns that string. Bun also exposes stdin as a BunFile via Bun.The steps are basically same as in sscanf solution, you create a format string & use it to capture the needed characters. 我正在尝试编写一个 C 代码 (在 Ubuntu Linux 操作系统上运行),它连续从标准输入读取,并且每次接收不同长度的字节。.To read a string entered by user via standard input in C language, use scanf () function.
Could you help me with some examples?
Reading an array of strings in C
7I found an easy and nice solution: char*string_acquire(char*s,int size,FILE*stream){ int i; fgets(s,size,stream); i=strlen(s)-1; if.To read an integer from stdin, you can use the following code: “`c int num; scanf (“%d”, &num); “` Similarly, you can read floats and characters using the “%f” and “%c” format . These streams are implicitly opened and unoriented at program startup. 写入stdin和从stdout读取(UNIX / LINUX / C编程) c read-write stdin stdout unix.20s to print just 20 characters).The standard input stream is the default source of data for applications.You should never use gets (or scanf with an unbounded string size) since that opens you up to buffer overflows. Furthermore, ‘sys.char str[25]; And you cannot use scanf to read sentences--it stops reading at the first whitespace, so use fgets to read the sentence instead.stdin’ is case sensitive, so the string you set as your exit string should match the input string case-wise too. First, you're passing uninitialized pointers, which means scanf and strcat will write memory you don't own.This requires reading input that users provide via the standard input stream (stdin) and printing results to standard output (stdout).comc - Reading input from stdin - Code Review Stack Exchangecodereview.I've just started learning node. Obviously, there are situations where you do need to process a line at a time from a file in a shell loop, but if . Try replacing fmt.c:32:5: warning: passing argument 2 of getline .
Mastering Input in C
/getline_example. Go through the link, you'll find it much easier than anything else Second, strcat expects two null-terminated strings, while c is just a character.3); my_printf . This post will discuss how to read a string from standard input in C++. (Then it will block again, until the user presses Enter again. So, to read a string from console, give the format and argument to scanf () function as shown in the following code snippet.Three text streams are predefined.Critiques : 5
Read String using Scanf() from User in C
Don't use Sscanln, because it parses a string already in memory instead of from stdin. The line consists of all characters up to and including .
printf(%s, secret); One thing to note, The printf() function can print the strings with whitespace.Having trouble with my main program, which should read from stdin (or redirect from a file) pairs of string and integer (string followed by int, one pair per line) and insert these pairs, in the order they are read, into an initially empty binary search tree. scanf () reads input from stdin (standard input), according to the given format and stores . read 32 chars from stdin, allocated 120 bytes for line : A short string to test getline!Writing to stdin and reading from stdout (UNIX/LINUX/C Programming)我正在做一个分配,其中程序将文件描述符作为参数(通常是在exec调用中从父级获取),然.4Using scanf removing any blank spaces before the string is typed and limiting the amount of characters to be read: #define SIZE 100. A simple solution is to use the std::getline function, which extracts characters from the input stream and stores them into the specified string . So you could use an extra variable to clear the buffer after the first read in your example.