C stl array
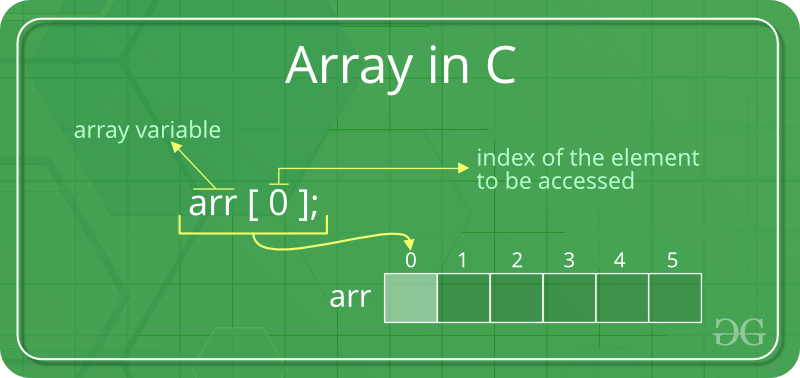
If you want to write your code like this, not specifying the array size, then you meant to use std::vector instead.
Three ways to avoid arrays in modern C++
template class array.Inside STL: The array. Here is the list of .std::array Overview. Career Advice and Roadmaps. Normal arrays are static objects: Their size is fixed and determined at compile time.// construction uses aggregate initialization std::array a1{ {1, 2, 3} }; // double-braces required in C++11 prior to the CWG 1270 revision // (not needed in C++11 .deduction guides for std::array.<< elements. All you get is built-in arrays and malloc. when you want to pass it to a C++ function the idiomatic C++ way is to pass begin + end pointers.Instead you should either use one of the standard containers (std::vector is the closest to a built-in array, and also I think the closest to Java arrays — closer than plain C++ arrays, indeed —, but std::deque or std::list may be more appropriate in some cases) or, if you use C++11, std::array which is very close to built-in arrays, but with value . std::array is a header-only implementation, which means that once you have a C++ runtime set up for your target system you will get this feature for free.La classe array définie dans est une collection d'éléments du même type de données stockés dans des emplacements de mémoire continue. It can store elements of same type, and we need to specify its size while creating an std::array object, because it is constructed at the compile time. One of the main advantage of std::array as compared to C style array is that we can check the size of the array using . int main {std:: array arr = {1, 2, 3}; for (auto i : arr) cout << i << '\n';} # Checking size of the Array. I hope you can leave some feedback! array. For elements of a class type .Unlike a C-style array, it doesn't decay to T* automatically. First, the program defines two arrays a and .However, with a normal type alias, we must explicitly specify all template arguments. < cpp | container | array.
C++11: Correct std::array initialization?
So the first question you should . It's not about performance for the most part; it's about correctness and convenience.
std::array reference
Dec 28, 2021 at 14:57. The elements of an array are numbered 0 , . We can then declare an array by giving it a name and specifying the type and quantity of things it will contain.
std::array vs C-Style fixed size array. A C-Style array is just a naked array - that is, an array that's not wrapped in a class, like this: Or a pointer if you use it as an array: And a C++ style array (the unofficial but popular term) is just what you mention - a wrapper class like std::vector (or std::array ). Unlike linked lists, arrays are guaranteed have its elements contiguous in memory.I'd like to have a review on my C-style array wrapper. Par conséquent, les objets de type array peuvent être initialisés à l'aide d'un initialiseur d'agrégat.
However, consider using .
What are the advantages of using std::array over C-style arrays?
template using vector = std ::vector< T, std::pmr::polymorphic_allocator>; } (2) (since C++17) 1)std::vector is a sequence container that encapsulates dynamic size arrays.
Is there a function to copy an array in C/C++?
Creating std::array objects.
The following example shows how we can declare an array that stores 5 integers:
Getting reference to the raw array from std::array
checks whether the container is empty (public member function) max_size . Data Structures and Algorithms. A class' type must be complete in order for a constructor to be called -- and for a class to be complete, it must be fully defined (and thus, a size must already be known). From cppreference. C arrays are inconvenient to use and are sources of bugs but even today I continue to see a lot of new code that uses them. If you don’t want the cli namespace, don’t make a cli project.
std::to
Standard C has nothing like std::vector or other container structures.
std::array equivalent in C
The standard library’s implementation of static arrays is called std::array.std::vector is a template class that encapsulate a dynamic array 1, stored in the heap, that grows and shrinks automatically if elements are added or removed.The array is a collection of elements of the same data type stored in continuous memory locations.Standard Library. The C-style arrays that are available in C++ are actually much less versatile than the real C-arrays. C++ standard library contains many libraries that support the functioning of arrays. Just like the old fixed size array.`array`は固定長のオブジェクトを保持するシーケンスコンテナで、各要素は連続して格納される。従来のCスタイルの配列のパフォーマンスを保ったまま、シーケンスのサイズの取得、要素の代入のサポートなど、標準コンテナの恩恵を受ける事ができる。 To use std::array, we need to #include .The use of C-style arrays is considered technical debt in modern C++.com/channel/UCs6sf4iRhhE875T1QjG3wPQ/joinPatreon 🚀 https://www. The array is a collection of homogeneous objects and this array container is defined for constant size arrays or .In C++ there are alternatives to C arrays, like std::vector and std::array.Containers replicate structures very commonly used in programming: dynamic arrays , queues , stacks , heaps (priority_queue), linked lists , trees , associative arrays . Before C++20, using array types in std::vector with the default allocator std::allocator was not supported, because std::allocator::destroy would try a simple (pseudo)-destructor call that is ill-formed for anything but class and scalar types. C++ STL 容器是使用频率超高的基础设施,只有了解各个容器的底层原理,才能得心应手地用好不同的容器,做到用最合适的容 . That array acts like any other array. As aggregate classes they can be constructed by means of the special member functions defined implicitly for classes (default, copy, move), or by using initializer lists:.#include #include int main( ) { using namespace std; array v1 = {1, 2}; array::iterator v1_Iter; array How to Access elements in std::array. Les objets array sont .The array classes are aggregate types, and thus have no custom constructors.Changing the extent (size) of std::array would change the size of the object containing it -- since the array elements are stored contiguously in the object rather than indirectly (e. Stack Exchange Network. Last Updated : 09 Jun, 2022.Table Of Contents. std::array provides many benefits over built-in arrays, such as preventing .It is just a syntax coloring glitch, it does not stop you from using std::array. std::array being a STL container, can use range-based for loop similar to other containers like vector. Syntax of std::array. You might think so, but you'd be wrong: Indexing outside of the bounds of a std::vector is just as bad as with a built-in array.< cpp | container | array C++. If expression is not an integer constant expression, the declarator is for an array of variable size.std::array solves numerous issues which C-style arrays have.C++ STL 十六大容器 —— 底层原理与特性分析.Variable-length arrays. In order to utilize std::array, you will need to include the array header: #include .ixx module; #include #include It will first and foremost invoke the aggregate initialization and then copy the value into the elements, it can't copy-construct the . Defined in: Overview. Data Structures and Algorithms . constexpr auto make_array(T value) -> std::array.Critiques : 9 #include #include int main {std:: array nums {1, 3, 5, 7}; std:: cout << nums contains << nums.namespace pmr {. The C++ standard library array is just a C-style array wrapped inside a class so that it behaves like a normal C++ object instead of a wacky thing that undergoes decay.The std::array is a STL Container, and it is similar to the C style fixed size array. returns the maximum possible number of elements (public .asked Jun 15, 2014 at 3:24. That is, someArray . Are there implementation guarantees in the stl that vector is, internally, consecutive in memory? As of C++03, yes, a vector is . But the question is, if std::array is similar to C-Style fixed .# Iterating through the Array. (C++20) (C++11) (C++20) (C++17) (C++11) [edit] Containers library. You are allowed to create a zero-length std::array, but C++ does not allow zero-length C-style arrays. Par exemple : C++. Since C++17 you can write a constexpr function to efficiently set up the array, since the element accessors are constexpr now. T elements[N]; The only weird case is N = 0.com/cppnutsCOMPLETE PLAYLIST Dynamic containers can have an arbitrary amount . But even when you have a (legacy) C array you have 2 situations: if you pass it to a C function you don't have the option of reference, so you are stuck to pointer + size. \n ;} Output: nums contains 4 elements. Stack Exchange network consists of 183 Q&A communities including Stack Overflow, the largest, most trusted .A declaration of the form T a[N];, declares a as an array object that consists of N contiguously allocated objects of type T. using Array2dint34 = . 2)std::pmr::vector is an alias template that uses a polymorphic allocator.This article will help you to understand about arrays in standard template library in C++.std::array:: fill.It also has several useful methods that let you perform operations that on a normal array . std::array just has an array as its member. Thereby array types did not satisfy the Erasable requirement with the default allocator, which std .STD::array in C++. The following is valid C code, but it can neither be expressed with C++ C-style arrays nor with the C++ array types: void foo(int bar) {.Le type a un constructeur par défaut array() et un opérateur d'assignation par défaut operator=, et il satisfait aux conditions requises pour un aggregate. Each time the flow of control passes over the declaration, expression is evaluated (and it must always evaluate to a value greater than zero), and the array is allocated (correspondingly, lifetime of a VLA ends when the .A fill-constructor would probably hide the fact that it isn't really constructing the individual elements.C++ STL: arrays (Complete Guide)
Array in C++ (STL)
array
Array declaration