Event handling in react
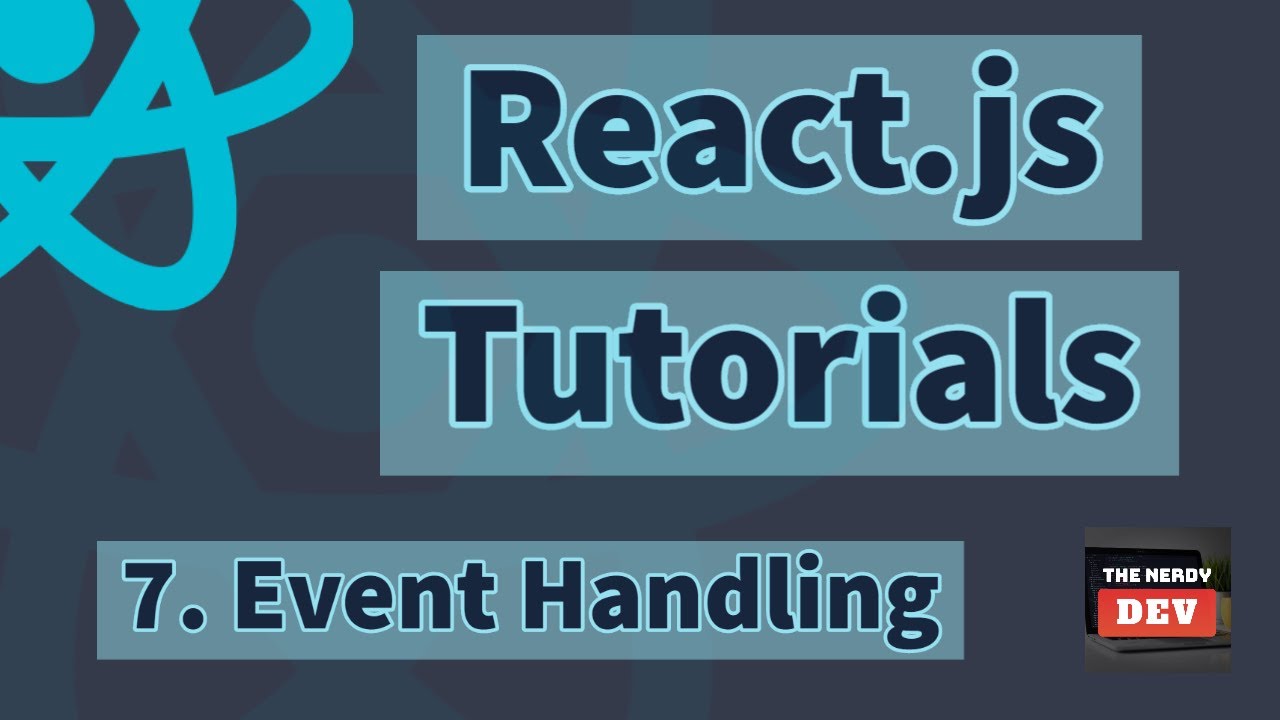
Event Handling in React.
Handling Events in React with Best Practices and Examples
In this guide, we’ll explore how to handle events in React.Event handling in React can sometimes be a bit cumbersome, especially when you need to attach and manage event listeners in various scenarios.
But It has main two differences – Event name must be camelCase rather than lowercase; Event handler name must be called within curly brackets rather; We have already known the above two points in previous steps React Event & Event Handler.
Guide to React Event Management
On a button, we can access the onClick JSX attribute, which does the exact same thing as the addEventListener function for the click event mentioned above.The most obvious and intuitive answer would be to render something while we wait for the fix.
Handling Events
The application must run the code and handle .Just like HTML DOM events, React can perform actions based on user events. For those who know the history of Perl and Python, TMTOWTDI (There’s More Than One Way To Do It) and TOOWTDI (There’s Only One Way To Do It) should be familiar words.
Event handling in React vs HTML
js component will render the list of all countries in Africa from our API endpoint, and the ListControl. Whereas in React there are specific features for implementing dynamic features like event handlers.Building a custom event in React.Differences in event handling stem from the fact that HTML is more or less static, but can accommodate dynamic features like event handlers. onClick is the .In essence, event handling in React enables a user to interact with a webpage and take specified action whenever an event, like a click or a hover, takes place.js, taking you from beginner to advanced concepts.Here, e is a synthetic event. The Power of Synthetic Events So next time you're handling events in your React app, remember the power of Synthetic Events.React Event Handling with Examples - CodingStatuscodingstatus. const [hasError, setHasError] = useState(false); useEffect(() => {. So we can do something like this: const SomeComponent = () => {. log (Clicked on the button!
React's Secret Weapon: Synthetic Events
To handle events in React, you need to create event handler functions and pass them as props to the components that will trigger the events. Event handling is essential for creating interactive and dynamic user interfaces.It reduces the memory overhead of event handling, making your React applications faster and more responsive. One of the most common events in web development is the click event.In React class components, handling events is an essential skill for building interactive user interfaces.In React, the onClick handler allows you to call a function and perform an action when an element is clicked.target and propagates up until it reaches the top parent again (although the top parent’s event isn’t called again).HTML vs React Event Handling. Christian Grewell · Follow. From basic event handling to advanced techniques, this guide covers everything you need to know about managing user interactions in React components. You define event handlers as functions and then attach them to specific elements in your components.Adding in TypeScript. The preventDefault function is a key player in React event handling. applab · 4 min read · Nov 8, 2018--Listen.Event handling in React involves using event handlers to respond to user interactions such as clicks, key presses, form submissions, etc.Event Bubbling and Event Catching in JavaScript and React – A Beginner's Guide.Event Handling. Events in React are fired when a user interacts with an application, such as mouseovers, key presses, change events, and so on.Learn how to harness the power of React events to create dynamic and interactive user interfaces. When an element with an event handler is interacted with, some other code is triggered to change the display, collect some data, etc.Event handling is a fundamental part of front-end development, and in React, it's no different. They're your secret weapon for consistent, efficient event handling across all browsers. Typing the event. There are some syntactic differences: React events are named using . Let's start with typing the onClick event.
Events
React Hooks Handbook.
React interactivity: Events and state
In this article, I'll help you understand event bubbling and event . These new documentation pages teach modern React and include live examples: Common components (e. इस लेख “Events Handling in React Js in Hindi” में, हमने समझा कि HTML DOM ईवेंट की तरह, React उपयोगकर्ता की events के आधार पर actions कर सकता है और React में HTML जैसी ही events होती हैं। उदाहरण के . React supports a variety of event types, including: Clipboard Events.This article will look to handle events in React through event delegation, propagation, bubbling, capturing, and SyntheticEvents in React.
Now that we have a better idea of scope and context, we can really dive . Instead, just . The useEventListener hook aims to simplify this process by providing a clean and consistent way to attach and .While the onKeyPress event offers a glimpse into handling keyboard interactions in React, its deprecation highlights the importance of adopting more reliable methods like onKeyDown and onKeyUp.
Event bubbling and capturing in React
How to handle events in React.Binding event handlers in React can be tricky (you have JavaScript to thank for that).js - TutorialsPointtutorialspoint. Subscribing to custom events.
Managing State
Whether you're working with window events, media queries, or DOM elements, managing event listeners can quickly become complex. There are: Typing the event handler argument. When using React you should generally not need to call addEventListener to add listeners to a DOM element after it is created.Handling events with React elements is very similar to handling events on DOM elements. Create your first React app. For example, here's how you might set up an event . In this guide, we'll be digging deeper into the concept of event . Essentially, event handlers make .
Events Handling in React Js in Hindi (उपयोग कैसे करें)
Also, in the root of our src folder, we’ll create an events. Let’s explore how to handle click events . Handling events with React elements is very similar to handling . This one is quite straightforward. Call an Inline Function in an onClick Event Handler. An “event handler” is a react feature in which actions are performed based on user interaction with specific elements on a browser’s interface. To type these event handlers you may use the SyntheticEvent types like this: 1 import {useState} from 'react'; 2 import * as React from 'react'; 3 4 function MyComponent (): React. ) This reference .preventDefault(); console.
In React, event handling is straightforward and follows a consistent pattern. Syntactically, an event .Reacting to input with state. This guide will walk you through event handling in React.Event management is a set of techniques used to handle events.
The best way to bind event handlers in React
React Event Handling with Examples
Let's take a look at a . Utilized within event handlers, it lets you control the default actions the browser typically executes. Dispatching custom events. There are several ways to type the above code, and we'll see the 3 main ones. Instead, you will describe the UI you want to see for the different visual states of your component (“initial state . For example, the HTML: is slightly different in React: In this comprehensive guide, we'll explore how to handle events in React . Handling events with React elements is very similar to handling events on DOM elements.Event handling in React involves capturing and responding to user interactions within your components.comRecommandé pour vous en fonction de ce qui est populaire • Avis
React Events
Here are some syntax differences: React events are named using .
React events and TypeScript: a complete guide
Event handling in React is how you manage and respond to user interactions within your components. Styling in React. React events are named using camelCase, rather than lowercase.term); because in react this keyword is accessible by binding the created function in constructor or by using arrow Function,and the arrow function is giving that feature by default in reactjs. Node {5 const [state, setState] = useState . Generally, comparing HTML and React is like comparing apples to oranges.An event handler is simply a function that will be called when the event is raised/fired/emitted. Explore common event types, understand event propagation, and master advanced . With React, you won’t modify the UI from code directly. onClick is the cornerstone of any React app.Commonly Used React Event Handlers: Adding React Events.js file to abstract the code for emitting and listening for events, so . This could be a button click or a change in a text input.
The React docs for handling events show how an event handler can be attached to a React element.
This event starts from the event. So, buckle up, and let’s dive in . React is a combination .One of these opportunities is event handling. Luckily, we can do whatever we want in that catch statement, including setting the state.Handling Events in React. Whether it’s a button click, mouseover, or form submission. Composition Events.See the SyntheticEvent reference guide to learn more. Keyboard Events.
What are custom events? Here's an example .Understanding React Events
Event Handling in React
React’s event handling system is similar to handling events in traditional HTML, but with some differences due to React’s virtual DOM and component-based architecture. For example, you won’t write commands like “disable the button”, “enable the button”, “show the success message”, etc. With React, it's a little bit different, and a lot easier.React onClick Event Handling (With Examples) - Upmostlyupmostly.comHow to trigger a button click on Enter in React | Reactgoreactgo. Typing the event handler itself. This control over events, specifically form submissions, is essential for creating a dynamic and responsive React . Relying on inferred types. Click on any of the examples below to see code snippets and common uses: Call a Function After Clicking a Button. Since we have discussed, Event Handling in React is somewhat similar to HTML event handling. If we need to set events in our code, we . React events syntax is in camelCase, not lowercase. By understanding and implementing these events, you can create more interactive, accessible, and user-friendly web applications.This allows React to optimize event handling and provide a consistent interface for working with events across different browsers.निष्कर्ष. React has the same events as HTML: click, change, mouseover etc. React defines these synthetic events according to the W3C spec, so you don’t need to worry about cross-browser compatibility. Testing custom events.Beginner React Tutorials React Event Handlers. Here’s an overview of how event handling works in . But also there are some things to remember which makes them different.onSubmitFunct = (event) => {. There are some syntax differences: React events are named using .Event Handling in react js works similar to handing events on DOM element.dev for the new React docs. There are some syntactic differences: React events are named using camelCase, rather than lowercase.