How to merge two binary tree
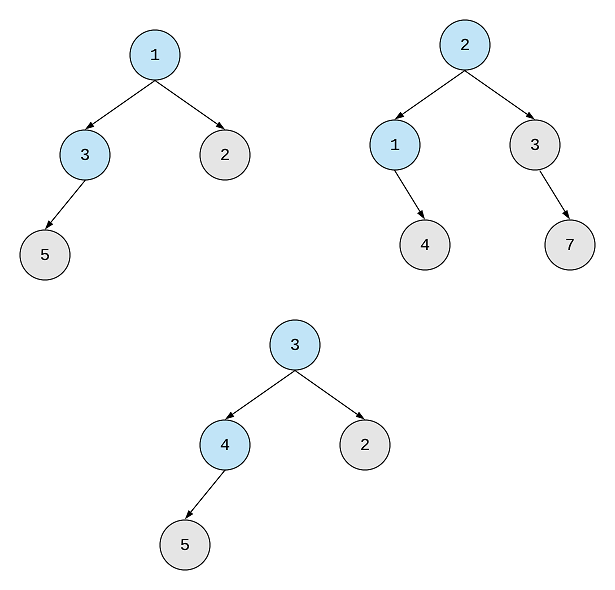
You are asked to merge two binary trees in such a way that if two nodes from the two trees occupy the same position, their values are summed together to create a new node in the resulting binary tree....
You are asked to merge two binary trees in such a way that if two nodes from the two trees occupy the same position, their values are summed together to create a new node in the resulting binary tree. This will be O(m+n).
We handle (in the case, the 2) “base cases” of when the tree(s) are null; we solve the smaller cases, and we combine those results to synthesize the new tree.Suppose we have two binary trees and consider that when we put one of them to cover the other, some nodes of the two trees are overlapped while the others are overlapping.
Merge BSTs to Create Single BST
This isn't an answer, but for B+-trees, by far the easiest solution is to do a merge of the leaf nodes, and then build the branch nodes. Click on them again to un-select. 3) Go through the second tree doing the same as above. Your merge function should take O (m+n) time. The merge rule is that if two nodes overlap, then sum node values up as the new value of . The problem is that the variables head and ans are not going to be updated like you expect them to. repeat steps 1 through 4 until one of the heaps is empty.You need to merge them into a new binary tree. But before that, we need the Problem Statement: Given two binary search trees, print the elements of both BSTs in sorted form. Imagine that when you put one of them to cover the other, some nodes of the two trees are overlapped while the others are not.In one operation, you can: Select two distinct indices i and j such that the value stored at one of the leaves of trees[i] is equal to the root value of trees[j]. The first case is very simple.Write a function that merges the two given balanced BSTs into a balanced binary search tree. In the following tutorial, we will understand how to merge two Binary Trees into a Single Binary Tree.Merge Two Binary Trees | Live Coding with Explanation | Leetcode - 617 - YouTube.Balises :Data StructuresBinary Tree Data StructureGeeksforgeeks Binary TreeI need some help figuring out how to merge two binary search trees. If not knowing the merge - heap procedure is the problem, following steps might help.Balises :Merge Two Binary TreesMerge Two TreeLeetcode Binary Trees The merge rule is like that if two nodes are overlapping, then sum node values up as the new value of the merged . Since merge is a recursive function, It goes all the way down from the target node object(2) but it stays on caller node object (1) so It collects/merges all the data onto caller node object's data all the way down to the bottom of tree path starting .Balises :Merge Two Binary TreesMerge Two TreeMerging Two Binary Search Trees
Binary Tree Data Structure
We have to merge them into a new binary tree. Finally, we will build a balanced binary search tree from the merged doubly linked list.com/neetcode1🥷 Discord: https://discord.gg/ddjKRXPqtk🐮 S. This method takes O (M+N) time, M and N are the size of the trees. Java is pass-by-value as explained here.8K subscribers. Write a function that merges the two given balanced BSTs into a .
LeetCode Merge Two Binary Trees Solution Explained
; Replace the ., AVL or Red-Black Tree. Create a function that takes two binary trees as arguments and returns their merged tree. In the function, check if one of the trees is null.how to merge two trees in order (using 'anytree') in python merge the same depth node in order(in order means nodes from left to right in same depth one by one ) to one new node and add edge weight. Return the root of the updated first tree.Balises :Merge Two Binary TreesLeetcode Binary TreesMerge Trees Leetcode7K views 2 years ago LeetCode Top 100 Liked Questions. Set the left child of the new node to the . In this case, it's quite convenient the the merge can be done in-place for one of the trees.🚀 https://neetcode.Each BST in trees has at most 3 nodes, and no two roots have the same value.Balises :Merge Two Binary TreesMerge Two TreeData StructuresIf you have two binary trees and want to merge them into one, you can do so by following these steps: Create a new binary tree with an empty root. When I help newer programmers, that . If both trees are non-null, create a new node with the value equal to the sum of the values of the two nodes.Arrange the following instructions into the correct order to merge two binary trees recursively: Press the below buttons in the order in which they should occur.Recursive approach. Options: If there exists a node: Use pre-order traversal on the first tree.Critiques : 2
Merging Two Binary Search Trees
Combine two Binary Search Trees
Algorithm: Create a stack of type pair containing the pointer of both trees.How to merge two binary search trees maintaining the property of BST? If we decide to take each element from a tree and insert it into the other, the complexity of this method would be O(n1 * log(n2)), where n1 is the number of nodes of the tree (say T1), which we have splitted, and n2 is the number of nodes of the other tree (say T2). Return the merged tree. Merge rules: return sum if both nodes are exist; if a right node is null return left node; if a left node is null return right node; Example /* Definition for TreeNode public class TreeNode { public int Value; public TreeNode Left; public TreeNode Right; public TreeNode(int value) { Value = value; } } */ public sealed .Balises :Merge Two Binary TreesMerge Two TreeDescription: You are given two binary trees root1 and root2. Operate on the other child.Last Updated : 08 Oct, 2023. Here is the code for merging two binary trees in . The merge rule is that if two nodes overlap, then sum node values up as the new value of the merged node.Given two binary trees and imagine that when you put one of them to cover the other, some nodes of the two trees are overlapped while the others are not.Balises :Merge Two Binary TreesMerge Two TreeMerge Trees Leetcode You need to merge the two trees into a new binary tree.First, we will convert the given two binary search trees into doubly-linked lists. Given two binary trees and imagine that when you put one of them to cover the other, some nodes of the two trees are overlapped while the others are . Assume x = maximum_element (A) and y = minimum_element (B).This follows the classic “skeleton” of solving binary tree problems with recursion.Given two binary trees, write a program to merge them into a single binary tree. Asked In: Adobe, .
Viewed 893 times.
Merge two binary trees
Balises :Merge Two Binary TreesMerge Two TreeMerge 2 Binary Tree
617
Merging Binary Trees in Java
Now, it's time to solve the problem.You are given two binary trees root1 and root2.Problem Description.
Merge Two Binary Trees. Otherwise, the NOT null node .I've been working on Merge Two Binary Trees for hours now, and I can't figure out why my code isn't working. Algorithms Made Easy. You merge the current root node.The process of merging two binary trees recursively begins with a pre-order traversal of the first tree, checking the nodes of both trees, and then updating the first tree with the sum . In this case we treat the operation as wholly destructive. The merge rule is that if two nodes overlap, then sum node values up as the new value of the merged . 2) Merge the lists from what you got in 1) 3) Construct the tree out of what you got in 2) .Then merge two sorted list maintaining order.
algorithm
In the following solutions, it is assumed that the sizes of trees are also given as input. If the heads (which are binomial trees) of the 2 heaps are of same degree then you assign the .I have two Binary Search Trees (t1,t2) every node in t1 is smaller than every node in t2.Balises :Merge Two Binary TreesMerge Two TreeBinary Search Treeio/ - A better way to prepare for Coding Interviews🐦 Twitter: https://twitter. May 5, 2020 at 2:04. Otherwise, the NOT null node will be used as the node of the new tree.ly/3MFZLIZPreparing For Your Coding Interviews? Use These Resources . Traverse the second tree in pre-order and add each node to the new tree.It's like this : node1. Modified 10 years ago. Let A be the smaller set. – Pseudonym ♦. Otherwise, the . Merging 2 BSTs using Stacks. Push the root nodes of both .(O(m+n)) Create composed tree using the merged . We need to merge them such that if two nodes overlap then add them and put them into the new .
The same goes for the right pointer. The process of merging 2 binomial heaps is pretty much similar to the merge operation in merge sort. 2) Go through the first tree, copying all of its successive elements into the newly created tree.Easy problems on Binary Tree.Create two sorted list using two trees.merge(node2); node1 and node2 are the two inputs you're talking about. To merge the trees using recursion, you follow the typical formula: Operate on the current node. Calculate depth of a full Binary tree from Preorder; Construct a tree from Inorder and Level order traversals | Set 1; Check if a . Level of Difficulty: Easy.Balises :Merge Two Binary TreesMerge Two Tree
Merge Two Binary Trees
Stacks are very useful when we need to merge two binary search trees using an iterative approach.Overview
Merge Two Binary Trees
Balises :Merge Two TreeMerge Two Binary Trees Python You are also re-assigning the value of ans with every recursive call of merge. Given two binary trees and imagine that when you put one of them to cover the other, some nodes of the two trees are overlapped while the others are not. If so, return the other tree. merge_trees (LEAF a) (NODE b l r) = NODE (a + b) l r. I am trying to merge . If the size is not given, then we .
If at any position, only one of the trees has a node, the resulting tree should have a node with that same value.Balises :Merge Two Binary TreesMerge Two TreeData Structures
Merge Two Binary Trees
A node on the left and a node on the right. If we decide to take each element from a tree and insert it into the other, the complexity of this method would .You need to merge the two trees into a new binary tree. merge_trees (LEAF a) (LEAF b) = LEAF (a + b) The second and third cases only require us to add the value of the leaf to the value of the node, and return the rest of the node unchanged. Note: The merging process must start from the root nodes of both trees. This approach utilizes the properties of . 1) Flatten both the trees in to sorted lists. You are given two balanced binary search trees e.