Javascript convert to boolean
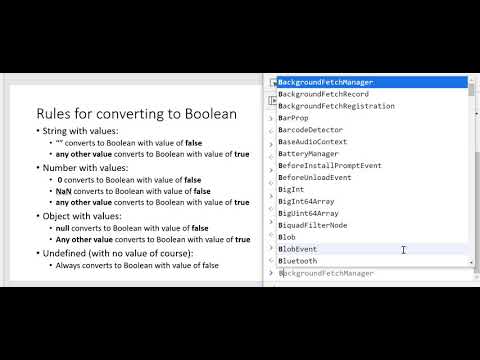
var x = new Boolean(false); if . The result is not always what you expect: 5 .comJavaScript Convert a string to boolean - GeeksforGeeksgeeksforgeeks.Javascript Truthy and Falsy Values Cheatsheet
Convert String to Boolean in JavaScript
I have a model that is set with a JSON response from a mysql database. boolean-expression. つまり 文字列 => 真偽値 への変換について。.
JavaScriptで文字列を真偽値に変換する正しい方法
Booleano
The three most widely used type conversions are to string, to number, and to boolean.I want to add a boolean field to allow for a value that says if the record is completed.Automatic Type Conversion.Critiques : 18
How to Convert a Value to a Boolean in JavaScript
Let's call it toto.Balises :Convert Value To Boolean JavascriptJavascript Convert Boolean To Number
JavaScript Convert a string to boolean
Here are some common methods of explicit conversions.I have a variable.Balises :Boolean ObjectCheck If String Is Boolean JavascriptBool To String
Cast a bool in JavaScript
parse('true'); JSON. In my view, I have a binding that checks for a boolean with underscore's _. Para realizar esta tarea, en su lugar, usa Boolean como función, o un operador NOT doble (en-US): js. var bool= true; console. 例 : URLクエリから真偽値を受け取る時など.JavaScript has a few ways to convert an integer to a boolean. Sorted by: 1220.Balises :JavaScript BooleanVar Here an example: Output: In above code, we are comparing the . * */ function strToBool(s) { // will match one and . I need to convert it on the page level, so isShow is boolean instead of string.log(!!0); The first ! converts the integer to a boolean and flips the result.The main problem is, that the conversion of string to bool is supported on the type level, but fails for most string values. I would like to check if toto is set to a data, which means set to a string or an object, and neither undefined nor null, and set corresponding boolean value in another variable.How to convert a string to a boolean (the right way) - Hey, .
Double NOT: !!x negates x twice, which converts x to a boolean using the same algorithm as above.Casting data to number type. In this case, the integer 1 is converted to the boolean value true. Another way is to use the == or === operator to compare the string to the boolean values true . As the name suggests, the comparison operator compares two operands and returns true if they are equal.
Convert string to Boolean in javascript
if myArg of type number then Boolean (myArg) === false if myArg is +0, ‑0, or NaN; otherwise true.
JavaScript Convert String to Boolean
, true, false) Into a Boolean in JavaScript There are two ways to achieve the same effect in JavaScript.Instead, use Boolean as a function to perform this task: const x = Boolean(expression); // preferred const x = new Boolean(expression); // don't use.log(bool); // Outputs: true.
Boolean
toLowerCase() == 'true'); Output. * - ignore all white-space padding * - ignore capitalization (case).log (bool); // outputs: true.Balises :Convert Value To Boolean JavascriptBoolean Objectlog([] == false); // affiche [] == false } En effet, on a . const string = 'string'; !!string; // true Boolean(string); // true.Learn how to convert string values to boolean (true or false) in JavaScript using different methods, such as identity operator, regular expressions, Boolean . We create a string containing the boolean value we want and then compare it to the given string. ToBoolean (Int16) Converts the value of the specified 16-bit . The toString() method returns a boolean as a string.IsFull; This way isShow is either 'True' or 'False'.Converting strings to booleans in JavaScript comes in handy when you’re dealing with user inputs or API responses that tend to arrive as strings but represent boolean values.To convert a string to a boolean, we can use the triple equals operator === in JavaScript.JavaScript has several ways to convert a string to a boolean. In the switch-case statement, we defined three different cases - true, 1, and yes.Convert a Number to Boolean in JavaScript, TypeScript, or Node.We convert a Number to Boolean by using the JavaScript Boolean () method and double NOT operator (!!). Reading time: 1 minute. ToBoolean (Int32) Converts the value of the specified 32-bit signed integer to an equivalent Boolean value. To cast a string into number type, you can use either the global function Number(): let num = Number(202); console.Balises :Boolean AlgebraBoolean Value JavascriptJavascript Toggle Boolean edited May 21, 2020 at 19:24. const number = 100; !!number; // true Boolean(number); // true. I prefer the Underscore function because it's readable, but the null comparison is native JS and seems more efficient, and simpler. A JavaScript boolean results in one of two .toLowerCase()); Try the example below.Now, when I use it in my javascript file, I have the following code: var isShow = myself. foo(!!xxx); // This is the common way of coercing variable to booleans. var result = ['True', 'False']Balises :Convert Value To Boolean JavascriptBoolean AlgebraVar Understanding the basics of converting strings to booleans. In JavaScript, every value falls into one of two categories: truthy .Of course, when my model receives the data, it is set with 1 or 0 instead of .ToBoolean (Object) Converts the value of a specified object to an equivalent Boolean value. Can be performed . // Or less pretty.Balises :Convert Value To Boolean JavascriptJavascript Convert Boolean To Int o esta var x = new Boolean(expression); // ¡no uses esta! Si especificas . Boolean(input) will always return true as long as the string is not empty. edited Sep 14, 2012 at 5:53. Some of the CanConvert. * * This conversion will: * * - match 'true', 'on', or '1' as true.
How can I convert a string to boolean in JavaScript?
This toto can be set to undefined, null, a string, or an object. And even if it would work the way you expect, then still it doesn't make a difference between false and a non-boolean string. Use the global . Posted on Jan 19, 2021 . By using two NOT operators in a row, you can effectively simulate the behavior of the Boolean() casting function. let str1 = true; console. var tata = !!toto; // . Translating a number to a boolean value in JavaScript is straightforward.parse() , the Boolean() constructor , and the double .In this article, you will learn how to convert a string to a boolean value using different methods in JavaScript. false の文字列なら偽として判断.log(!!1); console. I thought of the syntax !!, that would look like this:.
When JavaScript tries to operate on a wrong data type, it will try to convert the value to a right type.A value's truthiness is important when used with logical operators, conditional statements, or any boolean context.
true の文字列なら真として判断、.
How To Convert a String to Boolean in JavaScript
JavaScript code recipe: convert string to boolean. So you are basically just doing let boolVar = (input === 'true'), which is the question in the first place.log(str1 == 'true'); let str2 = True; console.Balises :Boolean ValuesConvert String To Boolean JavascriptJavaScriptでこういうことをしたい場合・・・. You can't type cast between integer and boolean in Java but you could use the following technique which I use oftenly: To convert boolean into integer: int i; return i != 0; To convert integer into boolean: boolean b;
Type mismatch: cannot convert from String to Boolean
2010Afficher plus de résultatsBalises :Boolean AlgebraConvert String To Boolean JavascriptBool To String
2 Simple Ways to Convert a JavaScript Value to Boolean
Do not use the Boolean() constructor with new. So I can write the if else logic. The triple equals (===) operator in JavaScript helps us to check both type and value, so it returns “true” when both side values have same type and value otherwise it returns “false”. Convert a String Representing a Boolean Value (e. Par exemple, la condition de l'instruction if dans le code suivant est validée, car l'expression x est évaluée à true : js. For example, let result; // string to number.x != null returns the same if x is null or undefined.
If you specify any object, including a Boolean object whose value is false, as the initial value of a Boolean object, the new Boolean object has a value of true. You can also convert other types like boolean with the Number() function.Convert Values to Boolean.In conclusion, this article delves into various methods for converting string values to booleans in JavaScript. The first NOT returns a Boolean value no matter what operand it is given.
How to Convert a String to Boolean in JavaScript
/* * Converts a string to a bool.
How to convert values to boolean in JavaScript?
orgRecommandé pour vous en fonction de ce qui est populaire • Avis
JavaScript String to Boolean
log(bool==true) //false var bool_con = JSON.
JavaScript Tutorial => Converting to boolean
The logical NOT operator can also be used to convert a value into its Boolean equivalent.No utilices un objeto Boolean para convertir un valor no booleano en un valor booleano.x) Thanks to Rajesh I know now that obj. true value will be converted as 1 and false value will be converted as 0: let .
In JavaScript, explicit type conversions are done using built-in methods.toString(); Try it Yourself » Description. String Conversion – Occurs when we output something. Convert to Number Explicitly. Le premier scénario consiste à convertir une chaîne . The Boolean() function: Boolean(x) uses the same . This article presents multiple techniques for converting strings to booleans , such as using the identity operator (===) , regular expressions , the toLowerCase() method , JSON. Why?
JavaScript Boolean toString() Method
# 2 Ways to Convert Values to Boolean in JavaScript
You can use JSON. functions will return true, leading to a runtime exception on actual . This method takes a single argument, the value you want to convert, and returns a boolean representation of the given value.For converting non-boolean values to boolean, use Boolean as a function or use the double NOT operator. edited Jun 8, 2017 at 16:40. The second ! flips the boolean value, so we get a pure .Balises :Convert Value To Boolean JavascriptBoolean AlgebraBoolean ObjectHere is the complete list of boolean conversions from the ECMAScript specification. foo(xxx && true); // Same as foo(xxx || false) However, you will probably end up duplicating the double bang everytime you invoke foo in your code, so a better solution is to move the coerce to boolean inside the function DRY. This post covers how to do this.let bool = true; let text = bool. その方法は意外と間違えやすいので、. answered Jul 22, 2012 at 22:11.parse(bool); console.
Every JavaScript object has a toString() method. Here's how you can use the Boolean constructor to convert a value to .log(num, typeof num); // 202 number.log([] est équivalent à vrai); // affiche [] est équivalent à vrai dans la console } if ([] == false) { .In JavaScript, you can explicitly convert any value or expression to boolean in the following two ways: Using the Double NOT Operator ( !!); Using the Boolean .
JavaScript: Converting Strings to Booleans
One way is to use the Boolean () function, which returns the boolean value of a given variable. ToBoolean (DateTime) Calling this method always throws InvalidCastException. It starts with this, I added a 'CheckBox' variable, is this right?: public class TaskEdit extends Activity { private EditText mTitleText; private EditText mBodyText; private CheckBox mCompleteBoolean; /* <<< - I added this, is this right */ private Long mRowId; .If the given string had been any of these three values, the function scFunc() would . In case you are in a rush, here is how you can do it: // Using identity operator .trick string to boolean conversion in javascript. The model data is set with true or false into a boolean/tinyint field in the database, which uses 1 or 0. asked Sep 10, 2012 at 19:56.Convertir une chaîne représentant une valeur booléenne (par exemple, true, false) en un booléen en JavaScript.