Logging in golang
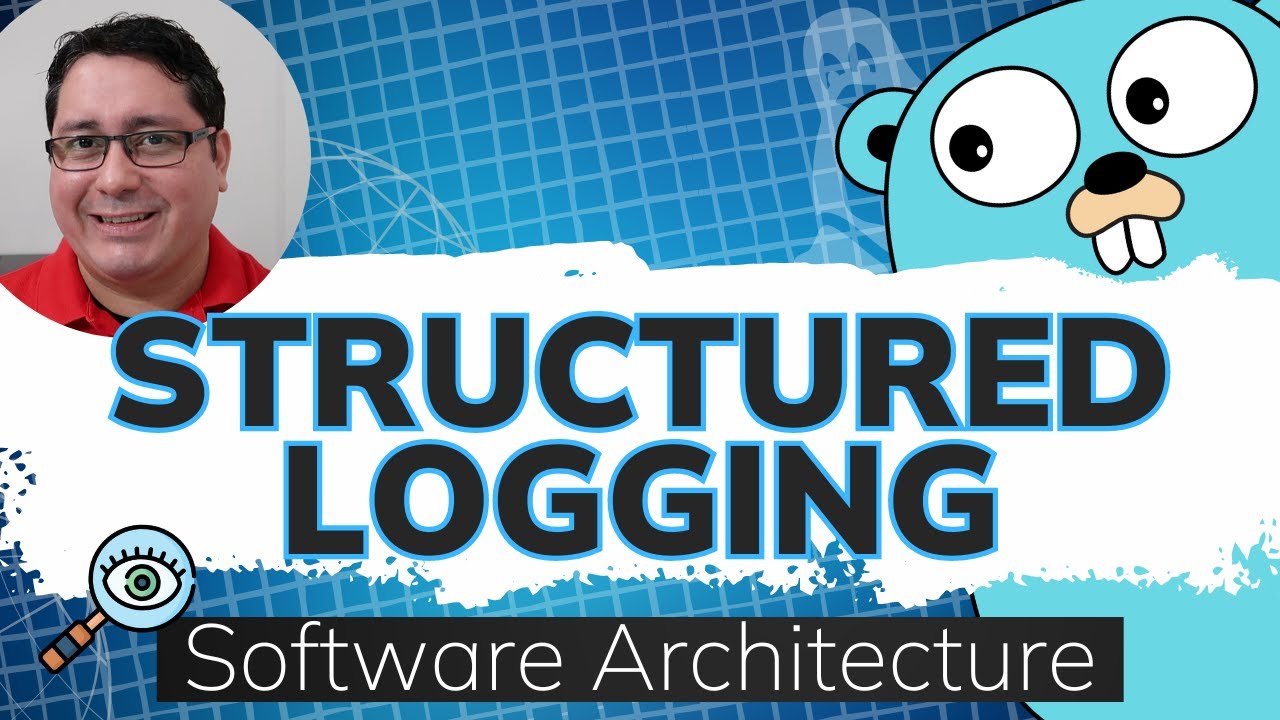
Check codebases that are out there.
logging
Its output format is customizable and supports different logging backends like syslog, file and memory. Sometimes libraries log. In contexts where performance is nice, but not critical, use the SugaredLogger. By default, the minimum level is Info, so calls to Debug (as well as top-level logging calls at lower levels) will not be passed to the log. See the functions, flags, and logger type of the log package with examples .A log record consists of a time, a level, a message, and a set of key-value pairs, where the keys are strings and the values may be of any type.Open() to open and append to an existing log file or using the built-in package log in Golang. We will implement middleware for formatting every request in our app using structured json format. It's 4-10x faster than other structured logging packages and includes both structured and printf -style APIs. Ayooluwa Isaiah. We will be building a simple, yet neatly organized Golang REST API with packages like Gin for Routing (mostly), GORM for persisting user data to a .Learn how to use the log package in Golang to log data at various segments of a program.In my program there is a lot places where I should create logs like following.
Structured Logging with slog
Go's standard library provides the log package, which offers basic logging functionality.ResponseWriter (more on this below) The duration of the HTTP request & response - until the last bytes are written to the . Create a single log.
Effective Strategies and Best Practices for Go Logging
Golang has an inbuilt logging library called log that comes with the default logger, which writes to standard error and adds the date for each logged message .Auteur : Ayooluwa Isaiah
Golang Logging: A Step-by-step Guide
OpenTelemetry is an observability framework – an API, SDK, and tools that are designed to aid in the generation and collection of application telemetry data such as metrics, logs, and traces.comUsing The Log Package In Go - Ardan Labsardanlabs. The default logger in Go writes to stderr (2).
A Guide To Writing Logging Middleware in Go
Go is a highly efficient, minimalist language with rich features such as channels and goroutines for system-level concurrent programming. That is possible. In this article, we'll explore structured logging in Go with a specific focus on the recently introduced log/slog package which aims to bring high-performance, structured, and . Build simple, secure, scalable systems with Go.The Go Programming Language. In Golang, having a well-thought-out logging strategy is crucial for debugging, monitoring, and gaining. The one I tend to use is a library called rs/zerolog. We leave the underlying logging . Sugar () sugar.I believe Logrus' biggest contribution is to have played a part in today's widespread use of structured logging in Golang. It's 4-10x faster than other structured logging packages and includes both structured .Best practices for logging in Go are not so obvious and sometimes we need to look closer to see what is the best choice, considering the unique situation of error .Should a Go package ever use log. You can inspect variables and stacks, set breakpoints, and do other . I need a logger that can log to multiple locations (which can be done with io.Best Practices for Designing and Storing Golang Logs (Datadog, 2019): Avoid declaring ‘goroutines’ for logging. A logging level acts as a message filter. NewProduction () defer logger.
The log package in Golang
Such log records are composed of key-value pairs that capture relevant contextual information about the event being logged, such as the .
log package
It defines a type, Logger , with methods for formatting output.
The status code written to the response, using our own implementation of http. The Go programming language has been exploding in popularity.In this tutorial, we will learn how to write logs to file in Golang.MultiWriter) and can do things like log. A web application’s Authentication protocol is in sessions. There doesn't seem to be a reason to do a major, breaking iteration into Logrus V2, since the fantastic Go community has built those independently. It offers excellent performance for high-load systems with minimal to zero . Zerolog is currently the fastest structured logging framework for Go programs.Authentication Sessions.First, it can lead to concurrency issues, as duplicates of the logger would attempt to access the same io. Zerolog is a high-performance, zero-allocation Go logging library. It helps with debugging and issue diagnosi.Effortless Structured Logs in Golang.Getting started.Logging in Golang uses Golang’s in-built library package or a third-party logging tool. The choice depends on specific needs such as ease of use, performance, and.What Is the ELK Stack? It’s hard to overstate how important logging is for most applications.go inside folder .Println(“[WARNING]: bla bla“) I use it for all my logging in different packages inside the application and it’s bit frustrating to copy this, is there a .Updated on December 28, 2023. Updated on January 31, 2024.The three most popular ways to log errors in Golang are: Output the errors to the console. Use a logging framework.The Code: LoggingMiddleware.All of these cloud-native technologies are written in the Go programming language, often referred to as Golang.But that won’t give you all the nice features that we will use soon.
Golang Zap Logger & SugaredLogger Tutorial
This article will walk you . It helps to track errors, monitor p. The web server matches it with existing credentials.What is difference between stacktrace and logs?A stack trace in Golang shows the sequence of function calls leading to an error or specific code point.Fatal and when?stackoverflow. The Go standard library provides straightforward tools for outputting logs from Go programs, with the log package for free-form output and the . Multiple backends can be utilized with different log levels per backend and logger.I have read this post which has the same title as mine, but it's about logging across go routines rather than packages. The web app receives it and sends to the web server. Users want to standardise logging. Many fantastic alternatives have sprung up.html template located in the web/template directory.I prefer the simplicity and flexibility of the 12 factor app recommendation for logging. This essentially helps you debug your. In Golang, logging is used to record important events and errors that occur during the execution of your program.Log is a logging interface for Go.com/op/go-logging - smaller than the other here. The SugaredLogger, on the other hand, trades some performance for a more developer-friendly API.
Structured Logging in Golang with Zap
The sugaring is essentially syntactic sugar, making logging more convenient and flexible.Printf(“[ERROR]: bla bla“) log.一个易于使用的,轻量级、可配置、可扩展的日志库。支持多个级别,输出到多文件;内置文件日志处理、自动切割、清理、压缩等增强功能 - gookit/slogGo Logging Slog.So somehow you need to pass through an ID yourself for logging.
debugging · golang/vscode-go Wiki · GitHub
Golang logging library.Package log implements a simple logging package. What I'm trying to solve is logging across the main app and any packages it uses.
A Guide to Go's `log` Package: Logging Best Practices
We have several ways to save the application log to specific files: we can use the os.
You can use these rough-and-ready logs for .comRecommandé pour vous en fonction de ce qui est populaire • Avis
Logging in Go: A Comparison of the Top 9 Libraries
Support multi level, multi outputs and built-in multi file logger, buffers, clean, rotate-file handling. In this article, we will learn about implementing JWT Authentication in Golang REST APIs and securing it with Authentication Middleware. logger, _ := zap.Error() and log.Handler and returns a net/http. You could just pass through an int yourself, but the context module is handy for this: apart from allowing user-defined values, it can handle item cancellation and deadlines which are also likely to be useful to you. Sync () // flushes buffer, if any sugar := logger. This article will provide guidelines for effective logging in Golang.In contexts where performance is nice, but not critical, use the SugaredLogger. In go, the common way to do that is to load configuration via environment variables (eg LOG_LEVEL, and use os. Logrus is in maintenance .Second, logging libraries usually .Before SetDefault is called, slog top-level logging functions call the default log. Log the errors to a file.Auteur : Ayooluwa Isaiah
Logging in Go: Choosing a System and Using it
It also has a predefined 'standard' Logger .
Logging in Go with Slog: The Ultimate Guide
Logrus would look like those .21 brings structured logging to the standard library.
go - Correct approach to global logging in Golanggo - How to use debug log in golangAfficher plus de résultatsLogging is a critical aspect of software development, providing valuable insights into the state of your application. An open-source programming language supported by Google.Which library is best for logging Golang?Popular logging libraries in Golang include logrus, zap, and go-kit/log. Along the way, you will install Go, write some simple Hello, world code, use the go command to . There are a few packages out there that do help. There are a few other competitors for the best log package out there, including the following:
How to Design a Basic Logging System in Your Go Application
A Complete Guide to Logging in Go with Zerolog
Golang’s built-in logging library, called log, comes with a default logger that writes to standard error and adds a timestamp without the need for configuration. To call the /login route, add a link to /login in the home.Package slog provides structured logging, in which log records include a message, a severity level, and various other attributes expressed as key-value pairs. In this tutorial, you'll get a brief introduction to Go programming. Output — The first row is regular logger, second row is zerolog. Structured logs use key-value pairs so they can be parsed, filtered, .Logger can be used concurrently from multiple goroutines. Easy to learn and great for teams. We will cover topics such as setting up a . That's why logrus for example allows you to set log levels via ints or strings.The first four route defined doesn’t need .Logging plays a pivotal role in the development and maintenance of software applications.Println(“[INFO]: bla bla“) log.This is the OpenTelemetry Go documentation.Before getting started with implementation, this article also concentrates on clean practices while developing the Golang application.In Golang, they are functions that takes in a net/http.
That being said, in terms of application development, logging packages are usually something that is external and can be treated like a utility to the entire application that can be switched if needed. In that mode, SetLogLoggerLevel sets the minimum level for those calls. This documentation is designed to help you understand how to get started using . There are two reasons to avoid creating your own goroutines to handle writing logs. NOTE: backwards compatibility promise have been . Let’s take everything we’ve learned above, with a middleware function that logs: The request method & path. Example 1: Logging to syslog file.The Go extension allows you to launch or attach to Go programs for debugging. Overview of Go's `log` Package.📑 Lightweight, configurable, extensible logging library written in Go.An example of a simple middleware is defined below: We’ll create a simple middleware that checks it token is passed in the request headers,validate and decode the token. Create new file called loggingMiddleware.
– Elias Van Ootegem. It provides structured logging capabilities for latency-sensitive applications where garbage collection is undesirable.
Get Started
Create a file called login. Both provide basic logging facilities that you can use to print messages based on different severity levels to the .go in the web/app/login folder, and add a Handler function. The procedure follows like this: A client sends an authentication request to the log in session of the web app. Structured logs are easy in Golang, so if you’re not already using them, you should start. From the above, we define our route. Upon executing the handler, the user will be redirected to Auth0 where they can enter their credentials. As an example, . Package logging implements a logging infrastructure for Go. This rule is provided by Auth0. This logging include Trace < Debug < Inf.What are types of loggers and which one is best?Logging classified logging messages to different logging levels.The application using this connection is the one you created in step 1.The new log/slog package in Go 1.What are logs in Golang?Logs in Golang refer to the mechanism used for capturing and recording information about a program's execution.