Memory management in c
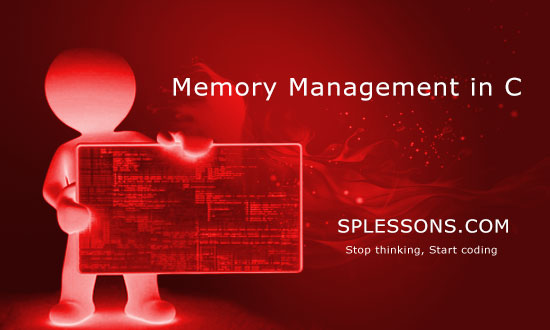
When an instance gets created the constructor of that instance is called, when that instance goes out of scope the destructor is called, this behavior is automated by the compiler, and is transparent to the programmer. The goal is to understand memory . The library also provides functions for controlling paging and allocation of real memory. Takes a pointer to the memory location.C dynamic memory allocation refers to performing manual memory management for dynamic memory allocation in the C programming language via a group of functions in . On the other hand, automatic memory management means the programming language automates this process by performing memory allocation and .
Example: Copy # include # .
Understanding Memory Management in C
Dynamic Arrays : In languages like C++, static arrays have fixed sizes determined at compile time. In the next article, I will discuss Boxing and Unboxing in C#.You have to do memory management when you want to use memory on the heap rather than the stack.In memory management, heaps refer to memory allocations beyond the stack.In this article, I want to mention how memory management is done in .Complete playlist. See examples of array initialization, memory deallocation .
Basic Memory Management in C
Topics: Computer memory layout (heap, stack, call stack), pointers and addresses, arrays, strings, and manual memory allocation/deallocation.If using int x; the allocation occurs on a region of memory called the stack.
C memory management library
See examples of allocating, resizing and releasing memory for .Learn the C runtime memory model, the standard library's memory management functions, and common pitfalls new C programmers can run into.orgWrite A C Program To Implement The Memory .Overview of Memory Management in Programming Definition of Memory Management.Critiques : 1
Guide to Memory Management and Debugging in C
Puis suivez les indications sur votre .
Mastering Dynamic Memory Allocation In C
malloc() calloc() realloc() free() To use these functions, we must include the stdlib. After that get the size of each block and process requests. C provides us with four functions, defined in the stdlib.Learn how to use C language functions to manage memory in your program, such as static and dynamic memory allocations, memory reallocation, and memory leaks. Smart pointers enable automatic, exception-safe, object lifetime management.
Manquant :
memory management Unconstrained Allocation.Memory Management Comparison to C.The GNU C Library has several functions for dynamically allocating virtual memory in various ways.2 Allocating Storage For Program Data.Stack and Heap Memory in C# with Examples
2 The calloc function.Garbage collection ( GC) is a mechanism in C# and other programming languages that automatically frees up memory that is no longer being used by the program. The essential requirement of memory management is to provide ways to dynamically allocate portions of memory to programs at their request, and free it for reuse when no longer needed. It refers to the process of allocating and deallocating memory for variables, objects, and other data structures used in a program. This is in depth video explaining memory management. 1 The malloc function.Dynamic memory management: Strings library: Algorithms: Numerics: Date and time utilities: Input/output support: Localization support: Concurrency support (C11) Technical Specifications: Symbol index Dynamic memory management. DISCLAIMER: This guide is intended to provide a summary of some key concepts . free_sized (C23) free_aligned_sized (C23) aligned_alloc (C11) Functions. Watch and share. Many programmers coming to C++ from C are used to doing manual memory management, particularly for string . S’il existe des virus ou des logiciels malveillants sur votre ordinateur, cette erreur d’écran bleu de Memory Management pourrait également apparaître, donc il est important d’effectuer une analyse antivirus sur votre PC pour détecter les problèmes. The Common Language Runtime's garbage collector manages the allocation and release of memory for an application. A memory manager determines where to put an application’s data. (C++20) (C++11) (C++20) (C++17) (C++11) [edit] Utilities library. Remember, this memory stays allocated until you decide it’s time to release it.
C++ Programming/Memory Management
The web page explains how to .comRecommandé pour vous en fonction de ce qui est populaire • Avis
C Memory Management
Learn how to allocate memory. I will try to keep it simple and short so that people with different levels of knowledge and experience can. Since there’s a finite chunk of memory, like the pages in our book analogy, the manager has to find some free space and provide it to the application.NET with Examples. class IntegerArray { public: int *data; 4. Ah, static memory allocation, the dependable old-timer of the memory allocation world! 🤠. Learn how to release memory.Learn how to use malloc (), calloc (), free () and realloc () functions to allocate memory dynamically in C programming.C Programming: Pointers and Memory Management - 4 | Coursera. Taught in English.C: Memory Management and Usage Instructor: Stephan Kaminsky. a (IntArrayWrapper) data. int * arr = ( int *)malloc(5 * sizeof( int)); // Allocating memory for 5 integers.Memory management is a form of resource management applied to computer memory. 21 languages available. Let’s take a look at the two fascinating realms of memory allocation: Static and Dynamic. Memory management is the process by which applications read and write data. Replacing malloc.
Memory Management in Programming: A Comprehensive Guide
Storage: Value types are stored in stack memory, while reference types are stored in heap memory.In C language, we use the malloc () or calloc () functions to allocate the memory dynamically at run time, and free () function is used to deallocate the dynamically allocated memory.C++ Memory management is a critical aspect of programming.Automatic memory management is one of the services that the Common Language Runtime provides during Managed Execution.Access: Stack memory access is more straightforward and faster, while heap memory requires more complex management.
C++, C) C++ supports dynamic memory management, which means you as the programmer are responsible for allocating and deallocating memory.h file at the top of our document.
[RÉSOLU] Écran bleu Windows 10/11 Memory Management
De-allocates memory that was previously allocated using new.
Type support (basic .Understanding how memory works in C is important. If using new int; the allocation occurs on a region of memory called the heap. Simply don’t use dynamic memory or allocate from the heap as you have alternatives like the Global space (extern/static globals and local static variables go here) or use the Stack space (typically 1MB limit on Windows, 8MB on Linux distros for every thread).Pointers & Memory Management in C Learning Goals: * Motivation * Pointer as an Abstract Data Type - Attributes and value domains - Operators (malloc, free, calloc, realloc) * Visualizing pointers w/ box-pointerdiagrams - More Operators: Assignment, Comparison, Initialization - Yet More Operators (pointer arithmetic) * What are Pointers used for . Memory mapped I/O is not discussed in this chapter.1 Error checking.Here is my list of good memory management tips for C (in my opinion). See examples of how global and static variables are . Whenever you use malloc or calloc, you are essentially utilizing a freed piece of heap memory that you possess. Allocation Debugging. See Memory-mapped I/O .
C Dynamic Memory Management Tutorial
Review •Pointers and arrays are very similar •Strings are just char pointers/arrays with a null terminator at the end •Pointer arithmetic moves the pointer by the size of the thing it’s pointing to •Pointers are the source of many C bugs! Let’s kick things off with a quick glimpse into what memory management is all about.
This leads to better resource utilization.Body: I'm trying to implement a custom memory allocator in C for managing dynamic memory allocation and deallocation. In the case of calloc, every memory slot is ., allocating and freeing the memory.In this series, I put together small, bite sized chunks of information that you don't necessarily need to be at your computer to learn from (which is rare in.Dynamic memory allocation in C refers to the process of allocating memory at runtime, allowing the programmer to manage memory resources as needed .Dynamic memory management (e.096 Introduction to C++: Memory management. Automatic Storage with Variable Size. If you don't know how large to make an array until runtime, then you have to use the heap. Memory Allocation in C Programs.Learn how C programs are organized into different sections in memory, such as text, data, bss, stack and heap.Memory Management: From Hardware to Software.Another Memory management aspect that is present in C++ but not in C, is that C++ have object oriented approach to classes .Resource Management: We can allocate memory when needed and deallocate it when it's no longer required.NET environment.com/l/1ugBm🔥Business Analysis Course for 0-3 Yrs Work Exp: https://l.
C Memory Management.Best Fit Algorithm.The Python memory manager has different components which deal with .🔥Business Analysis Course for 3-8 Yrs Work Exp: https://l. The pointer arr now points to a memory block that can store 5 integers.Learn how to use malloc, calloc, realloc and free functions in C to allocate and release memory dynamically.h file, to use for dynamic memory allocation. This course is part of C Programming with Linux Specialization. This is an important part of memory management in C#, as it allows programmers to focus on the logic of their programs without having to worry about manually freeing up . Then select the best memory block that can be allocated using the above definition.malloc (memory allocation) carves out a chunk of memory from the heap. Distinguish between stack-based and heap-based memory allocation. of Processes and no.Dynamic memory allocation in C is a way of circumventing these problems. They vary in generality and in efficiency.Learn how to manage memory in C with stack and heap variables, malloc and free functions, and examples of common pitfalls and solutions. In C++ memory management can be done explicitly using the new and delete operators that we are going to explore later in thai article.Memory Management¶ Overview¶. int *x = new int; // use memory allocated by new. Great Idea #1: Levels of . This section covers how ordinary programs manage storage for their data, including the famous malloc function and some fancier facilities special to the GNU C Library and GNU Compiler.In the whimsical land of C programming, memory management plays a crucial role in keeping your code running smoothly. C++ also supports these functions, but C++ also defines unary operators such as new and delete to perform the same tasks, i. For example, you might want to store something in a string, but don't know how large its contents will be until the program is run. This is critical to any advanced computer system where more than a .Solution 6 : Effectuer une analyse antivirus. When you create a basic variable, C will automatically reserve space for that variable. Language support.Dynamic memory management: Strings library: Algorithms: Numerics: Date and time utilities: Input/output support: Localization support: Concurrency support (C11) . By Soura Mandal and Jonah Meggs.
C Programming/Memory Management
Automatic Memory Management
Memory Allocation in C Programs.
In the world of programming, memory management refers to the process of controlling and coordinating computer memory, enabling efficient allocation and .
C Memory Management (Memory Allocation)
An int variable for example, will typically .