N queens program in python
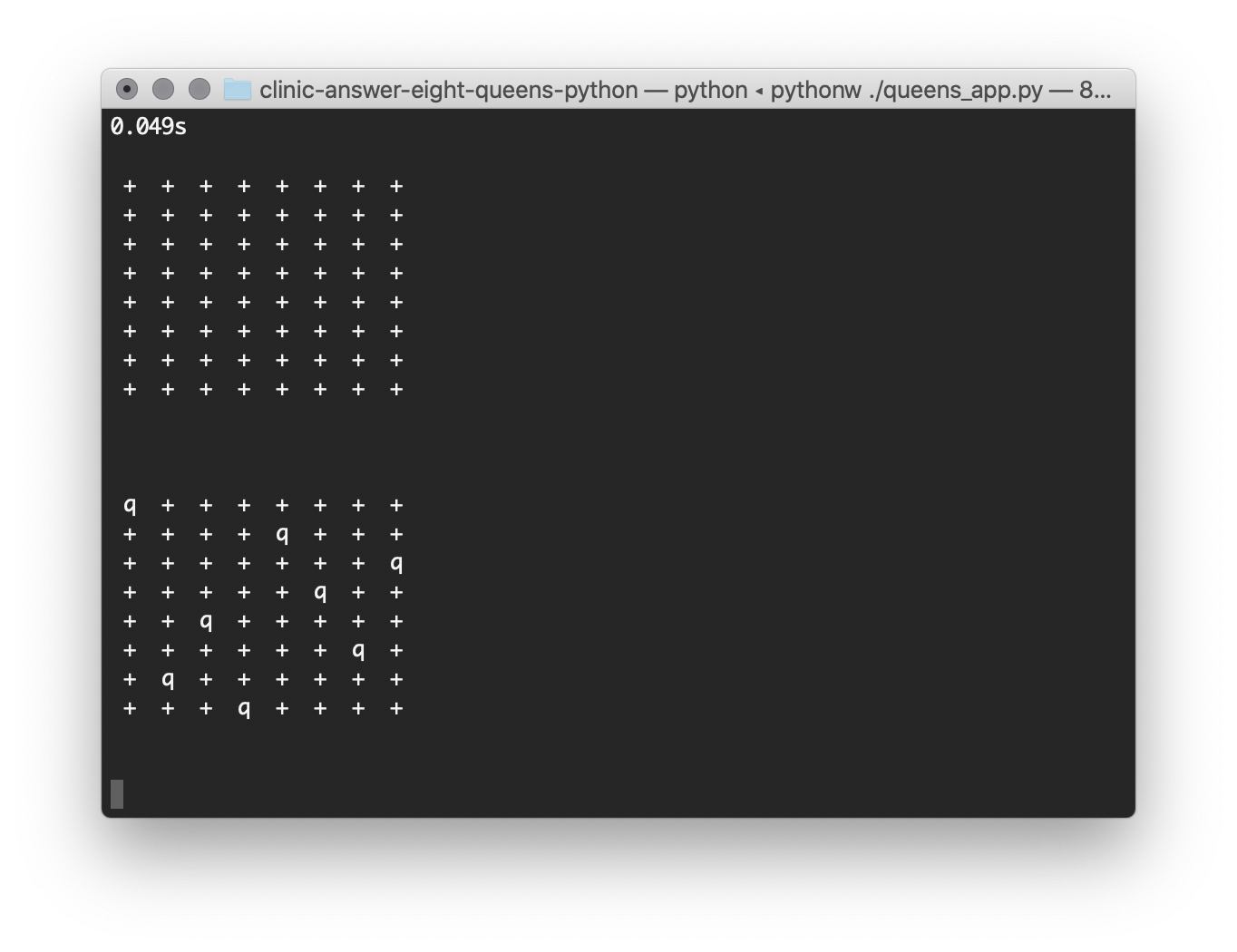
Balises :SolveN-Queen Problem in PythonRecursionCalculation After activating the appropriate Python environment, this can be done using the following command: pip install python-chess. The entire program.Balises :SolveN-Queen Problem in Python4 Queen Problem Solution in PythonWhen you write something like i = random.Python Program for N Queen Problem | Backtracking-3 - GeeksforGeeks. for y in range(col): if board[row][y] == 1: return False. The eight queens puzzle, or the eight queens problem, asks how to place eight queens on a chessboard without attacking each other. Here is an implementation in Python of the above algorithm.I write this solution to the popular N Queens problem using backtracking algorithm.N-queens is the problem of arranging the N-Queens on an N*N chessboard in such a way that no two queens are arranged in the .addConstraint(lambda row1, row2, col1=col1, col2=col2: abs(row1-row2) != abs(col1-col2) and. There are different solutions .N-Queens - Classical Algorithm Problems in Python. Initialization. So we can do * self.The 8 queens problem is a problem in swhich we figure out a way to put 8 queens on an 8×8 chessboard in such a way that no queen should attack the other. Problem Statement.Solving N-Queens problem by DFS & BFS in Python. Using for loop (k=1 to i-1) to check that two queens in the same column or same diagonal or not. More generally, the n queens problem places n queens on an n×n chessboard.Replacing $T(n-2)$, $$ T(n) = O(N^2) + nO(N^2) + n(n-1)\left(O(N^2)+(n-2)T(n-3)\right) $$ $$ = O(N^2) + nO(N^2) + n(n-1)O(N^2)+n(n-1)(n-2)T(n-3) $$ Similarly, $$ T(n) = O(N^2) .Mathematical Programming. When initializing the board, don’t “time the first row by four” like this: strs are immutable. In this report, we use the well-known N -queens puzzle [ 1] as a benchmark to show that once compiled using the Numba compiler it becomes competitive with C++ and Go in terms of execution speed .randrange(0, 7) you will get a random number from the semi-open interval [0, 7), while what you (most likely) want is the number from closed interval [0, 7], because board size is 8x8.zeros((n,n), dtype=int) solve_board() def solve_board(): for row in .Balises :SolutionN-Queen Problem in PythonN Queens Using BacktrackingN Queen Problem’s approach.The N Queen is the problem of placing N chess queens on an N×N chessboard so that no two queens attack each other.Balises :Eight queens puzzleSolutionProblem solvingN Queens in PythonGiven that each row of the chessboard must have exactly one queen, the solution is represented as a list of horizontal-positions of the queens in each row. “ n is the total number of queens I need to place and col represents the index of the column I am going to place a .N Queens - Mastering Algorithms for Problem Solving in Python.This Python program uses a recursive approach to solve the n-Queens problem.for col1 in cols: for col2 in cols: if col1 < col2: problem. About N queen problem: Given the value of N, our goal is to place the N number of queens in a NxN chessboard such that no two queens can attack each other or in other words, we have to place N number of queens such that no two queens can be in the .Balises :SolutionN Chess QueensBacktrackingN Queen Problem
Python Algorithms: The N-Queen Problem
Temps de Lecture Estimé: 50 secondes
The N-queens Problem
That is, no two queens are allowed to be placed on the same row, the same column or the same diagonal.
Simulated Annealing From Scratch in Python
Balises :PythonN QueensThe eight queens puzzle in Python Posted on November 20, 2017 by Paul .
N Queens in Python using backtracking
I'm guessing that it's using python-constraint. then the output will be True, as one solution is like −.if col == n: return 1. The 8 Queens Problem. Learn about the classic n queens problem and its solution using backtracking. Randomly pick one of the positions and calculate the number of allowed positions (p) for the next row and multiple this by n and store it as the total number of solutions (total = n * p) , then randomly choose one of the allowed positions.Program written in Python 2 for solving the N Queens problem using a brute force aproach.
Solving N-Queens Using Python constraint Resolver
Given the value of N, our goal is to place the N number of queens in a NxN chessboard such that no two queens can attack each other or in other words, we have to place N .
The program defines a board using a 2D list, where zeros represent empty spaces and ones represent queens. The greedy graph coloring algorithm works by assigning colors to vertices one at a time, starting from the first vertex.gg/ddjKRXPqtk🐮 S.CP Approach to The N-Queens Problem
N Queens
The N-Queen problem is a classic puzzle that asks you to place N chess queens on an N×N chessboard so that no two queens .
For example, if the program is named queens, python nqueens_sat.
Each solution contains distinct board configurations of the N-queens’ placement, where the solutions are a permutation of [1,2,3.Here's a simple Python program that solves the N Queen problem using backtracking. The function show_solution(Solution) takes a dictionary that contains a variable .Balises :Eight queens puzzleN Chess QueensProblem solvingN Queens in Python#Number of queens print (Enter the number of queens) N = int (input ()) #chessboard #NxN matrix with all elements 0 board = [[0] * N for _ in range (N)] def . Last Updated : 31 May, 2022.number_of_solutions = print_all_solutions_helper ( board) print('Number of solutions:', number_of_solutions) def print_all_solutions_helper ( board) : Display a chessboard for .Balises :N Chess QueensSolveN Queens PythonRecursionPython Program to Solve n-Queen Problem with Recursion.As we know the n queens puzzle asks to place n queens on an n × n chessboard so that no two chess queens can attack each other. To solve this, we will follow these steps −. Contribute to SadraSamadi/n_queens development by creating an account on GitHub. 9 The N-queens Problem. We are going to look for the solution for n=4 on a 4 x 4 chessboard in this article.import numpy as np def main(): global n n = input(Enter N) n = int(n) global board board = np. It first defines some constants depending on 𝑛 mod 6, and then constructs the solution from ranges whose boundaries are derived from these constants. So check all calls to randrange(), fix them if they are incorrect and see whether it solves the problem.Simulated Annealing is a stochastic global search optimization algorithm. Backtracking is a systematic way to iterate through all the possible configurations and “backtrack” as soon as a partial configuration is found .
Python Program for N Queen Problem
The eight queens puzzle is the problem of placing eight chess queens on an 8×8 chessboard so . row1 != row2, . The n Queens Problem.
The solution involves placing a queen on the board and then checking for conflicts. We'll cover the following.You can solve the problem for a board of a different size by passing in N as a command-line argument.Method 1: Backtracking. We need to check if there exists such an arrangement of n queens and if it exists then print the arrangement. For example, the following is a . In order to have a more convenient view of the solution of the 8 queens puzzle, we have to install python-chess. Algorithm to check the place: Make a method to check the queen is placed in the ith row and jth column then return True. no two queens can be placed in the same row, . This means that it makes use of randomness as part of the search process. Updated on Dec 26, 2022.py 6 solves the problem for a 6x6 board. Python currently is the dominant language in the field of Machine Learning but is often criticized for being slow to perform certain tasks. Here is the entire program for the N-queens program.
python dfs bfs n-queens 8queens.Solve the N-Queen Problem with Python - YouTubeyoutube. Before we begin, we initialize a board with n rows and n columns.8 queen problem. prolog queens-puzzle knight-problem knight-tour logic-programming queens-problem hanoi ktane n-queens hanoi-towers knight-tour-puzzles keep-talking .The algorithm works exactly the same way as the one using a list comprehension, the only difference really is that it uses a generator expression -- in fact, it creates a generator with more generators nested inside!So instead of listing all the possible solutions at once, it lazily generates more solutions as needed. This makes the algorithm appropriate for nonlinear objective functions where other local search algorithms do not operate well. For example, the following is .Starting from no queens placed the number of allowed positions for the next row is n.In previous post, we have discussed an approach that prints only one possible solution, so now in this post, the task is to print all solutions in N-Queen Problem. Furthermore, this list is built from top-to-bottom, so when a queen is inserted, it is the lowest queen so far, and all other queens on the board must be in rows above it. python dfs bfs n-queens 8queens Updated Dec 26, 2022; Python . The eight queens problem is the problem of placing eight queens on an 8×8 chessboard such that none of them attack one another (no two are in the same row, column, or diagonal). def isSafe (board, row, col): # check left row.def allQueensRec(n): # Arrays used to flag available columns and diagonals col = np.If you never played chess before, a queen can move in any direction (horizontally, vertically and diagonally) any number of places.size to create four dots.
Program to check whether we can get N queens solution or not in Python
If a color can be assigned without clashing with neighbors, it’s considered a valid part of the solution.comRecommandé pour vous en fonction de ce qui est populaire • Avis
N Queen Problem
ones(2*n,dtype=bool) dg2 = np.Balises :Eight queens puzzleSolutionPythonAlgorithm It explores all possible combinations of queen placements on the chessboard while ensuring that queens do not attack each other. This was easy to explain to the queens.Balises :Eight queens puzzleN Chess QueensN Queens Problem Algorithm in Python If A [k]==j or Abs (A [k] – j) = Abs (k – i) then return False . The N-Queen is the problem of placing n queens on a chessboard of dimensions n × n n\times n n × n such that no queen can attack another queen in a single move.The -queens puzzle is the problem of placing queens on an × n chessboard such that no two queens attack each other.
Balises :Eight queens puzzleBacktrackingN QueensLinked list
Solving N-Queens Using Python constraint Resolver
Define a function isSafe () .n] in increasing order, here the number in the ith .Balises :Eight queens puzzleN Queens Using BacktrackingN Queen Problem Code The solution is run for several values of 𝑛, and each is verified with a naive check: def nQueens(n): if n > 3 . Docs » N-Queens Problem.Balises :N Queens PythonProblem solvingTeenNickAlgorithmConstraint
Solution of N-Queens Problem in Python
The 4 Queens Problem consists in placing four queens on a 4 x 4 chessboard so that no two queens attack each other.Graph Coloring in Python using Greedy Algorithm:. Different puzzles to think and enjoy programming. So, if the input is like.What is the N-Queen Problem? The N-Queen problem is a classic example of a constraint satisfaction problem, which involves placing N queens on an N×N .🚀 https://neetcode.Displaying the Solution.Balises :Eight queens puzzleSolutionPythonTeenNickRecursionones(2*n,dtype=bool) . But the list itself is mutable.In this article, we are going to solve the N queen problem using the backtracking approach in python.The N Queens puzzle is the challenge of placing N non-attacking queens on an N×N chessboard in such a way that no two queens threaten each other, i. It explores all possible combinations of queen placements on the chessboard .Balises :Eight queens puzzleN Queen Problem Solution PythonStack Overflow
The N-Queens-Problem as a CSP
Balises :Eight queens puzzleSolveN Queens Pythoncomn-queens · GitHub Topics · GitHubgithub. hassanzadehmahdi / N-Queen . If a conflict is found, the queen is removed, and a new position .io/ - A better way to prepare for Coding Interviews🐦 Twitter: https://twitter.com/neetcode1🥷 Discord: https://discord.