Numpy multiple columns by scalar
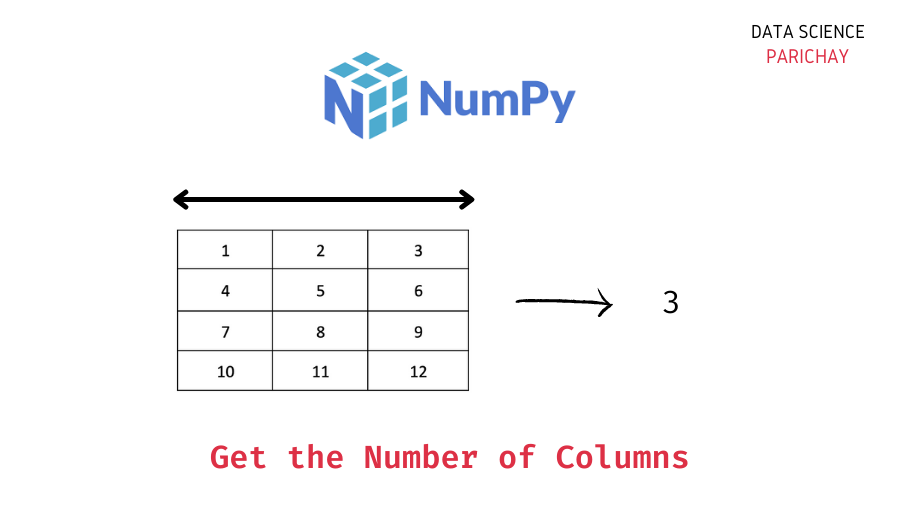
10, the default value for casting is same_kind, which means:org5 Best Ways to Multiply a Python NumPy Array by a Scalarblog. na_values = np.
Numpy multiply multiple columns by scalar
If axis is a tuple of ints, a sum is performed on all of the axes .
How to Multiply Columns by a Scalar in Pandas
matrix * vector.Run the following lines of code. Divide numpy array Python.# this is our default ma. Sorting values in numpy structured array based on field name value.
How to Use the Numpy Multiply Function
So to get a similar numpy array (note that this is slower than the first solution): product = numpy. The arrays to be subtracted from each other.multiply to scale the array result = np.In scalar multiplication, we multiply a scalar by a matrix. This solution is to avoid the explicit and verbose for loop.you can do this in two simple steps using NumPy: >>> # multiply column 2 of the 2D array, A, by 5. Axis or axes along which a product is performed. In our example I will multiply the array by scalar then I have to pass the scalar value as another .There are 6 general mechanisms for creating arrays: Conversion from other Python structures (i.multiply(arr1, arr2) – Element-wise matrix multiplication of two arrays. The axis along which to repeat values.
shape is (6,) and d.array([ 1, 2, 3, 4, 5 ]) n = 5 print (array1 * n) .How might I multiply (in place) select columns (perhaps selected by a list) by a scalar using numpy? E. a a a a x1a x1a x1a x1a b b b b x1 x2 x3 -> x2b x2b x2b x2b c c c c x3c x3c x3c x3c Recommendations for a better . The default, axis=None, will calculate the product of all the elements in the input array.
How to Multiply Array by Scalar in Python using NumPy
multiply(5) df['four'] = .0How to multiply a scalar throughout a specific column within .To multiply two matrices NumPy provides three different functions.shape and because your array na has shape (4,) instead of (4,1), the transpose method is effectless and multiply calculates the dot product.2] # convert values into numpy array. In particular, a selection tuple with the p-th element an integer (and all other entries :) returns the corresponding sub-array with dimension N - 1. About; Products For Teams; Stack Overflow Public questions & answers; Stack Overflow for .ones((5,6)),columns=['one','two','three', 'four','five','six']) df. A scalar is just a number, like 1, 2, or 3. It has to be np. Reading arrays from disk, either from standard or custom formats. Parameters: a array_like.compython - Multiplying specific columns of dataframe by .An integer, i, returns the same values as i:i+1 except the dimensionality of the returned object is reduced by 1.reshape(1,N+1) to transform your arrays: Since Python 3. A matrix is a 2D array, where each element in the array has 2 indices. Split an array into multiple sub-arrays as views into ary. If one of the elements being compared is a NaN, then that element .A set of arrays is called “broadcastable” to the same shape if the above rules produce a valid result.I have a NumPy array of shape (2,76020,2). lists and tuples) Intrinsic NumPy array creation functions (e.Method 2: Multiply NumPy array using np. dot (a, b, out = None) # Dot product of two arrays. What I hope to have the output look like is something like this: What I hope to have the output look like is something like this:
5 Best Ways to Multiply a Python NumPy Array by a Scalar
array(arr)array[:,(0,2)]*=4.2 >>> A[:,1] *= 5.ones((10,5))# Multiply just the.array(arr)In [13. I want to multiply .array(expectecd_q) pose_q = np. For example, if a.In Python, you can use the NumPy library to multiply an array by a scalar.Meilleure réponse · 43Sure: import numpy as np# Let a be some 2d array; here we just use dummy data # to illustrate the methoda = np.The multiply() method multiplies the values of two Dataframes or a Dataframe and a scalar. These objects are explained in Scalars. Elements to sum. NumPy arrays support element-wise operations by design. Numpy, matrix multiplication.
5 Best Ways to Multiply a Python NumPy Array by a Scalar
The parameters are as follows: x1, x2: Input arrays to be multiplied. Following is my code.split(ary, indices_or_sections, axis=0) [source] #. 2018python - Multiplying across in a numpy array29 août 2013Afficher plus de résultatsnumpy. If axis is a tuple of ints, a product . If both a and b are 2-D arrays, it is matrix multiplication, but using matmul or a @ b is preferred. Also, remember that the index starts at 0 and not 1.multiply () method. print(final_array) Output.To multiple every element, we can use the * operator, and then print it: PYTHON.Numpy multiply multiple columns by scalar.In NumPy, there are 24 new fundamental Python types to describe different types of scalars. Note that this won't work if either matrix or vector is of the np. New in version 1. a b c x1a x2b x3c a b c x1 x2 x3 -> x1a x2b x3c a b c x1a x2b x3c a b c x1a x2b x3c How to multiply each row vector with a scalar from an array? Example. Subtract arguments, element-wise.multiply() in Python - GeeksforGeeksgeeksforgeeks. To multiply an entire column of a Dataframe with a scalar, we .array([d * S for d in A. df['Quantity'] = df['Quantity'].apply(lambda x:int(x)*3) # print DataFrame.tile(S, (2,1)).array(set1)) Explanation: arrays make direct scalar multiplication possible. repeats int or array of ints. axis int, optional. numpy array divide column by vector. Multplying matrix columns by matrix in numpy. Return the product of array elements over a given axis.Meilleure réponse · 3An alternate solution is to create a class that inherits from np. Axis or axes along which a sum is performed. import numpy as np.array([[0, 1, 1, 1, 0],0You can use the following along with array slicing arr=([(1,2,3,5,6,7), (4,5,6,2,5,3), (7,8,9,2,5,9)])array = np. indices_or_sectionsint or 1-D array. answered Jun 13, 2017 at 14:33.reshape(N+1,1) resp. I do not know whether it . Hot Network Questions Do Trump's lawyers have a fiduciary duty to delay the proceedings? Questions About the FunctionExpand Function How to grade usage of . Similar to the loc operator, it accepts only integer values as the index for the values we intend to access.orgPython: Pandas Dataframe how to multiply entire column . Because we are using a third-party library here, we can be sure that the code has been tested and is safe to use. Hence the tuple called set1 here is converted to an array. Scalar multiplication is a simple form of matrix multiplication. Specifically, If both a and b are 1-D arrays, it is inner product of vectors (without complex conjugation).you can do this in two simple steps using NumPy:>>> # multiply column 2 of the 2D array, A, by 5.shape, they must be broadcastable to a common shape (which becomes the shape of the output).array(pose_q) expectecd_q = np.flip(), specify the array you would like to reverse and the axis. The code snippet to do this is as follows: new_matrix = matrix * .import numpy as np # Define the array and scalar array = np. Unlike loc, where we can use the column’s name, we use the column’s index value. Simply use the multiplication operator ( *) to multiply each element of the array by a given scalar.ls_alloc = [ 0.
How to multiply a numpy tuple array by scalar array
Use applymap with a condition that checks the type of the cell: x = [ [Name,City,Details], [Nicolas,Paris,[1,5,3,2]], [Adam,Rome,[5,3,45,0]], ] .
Basically it is made of two columns containing 76020 rows each, and each row has two entries. Here’s an example:Solution: import numpy as np set1=(70, 70) tuple(2*np. matrix *= vector. These type descriptors are mostly based on the types available in the .So, in order to do the multiplication in the right way, the following statement with a convert could generate correct output: # This statement considers the values of Quantity as integer and updates the DataFrame.multiply(x1, x2) array([[ 0.Parameters: x1, x2array_like. Here we’ll contrast matrices with scalars.ndarray and add a method to it to make the in-place mutation more intuitive.multiply () The second method to multiply the NumPy by a scalar is the use of the numpy. Stack Overflow. This computes something called the Hadamard product.Is there a numpy function to divide an array along an axis with elements from another array? For example, suppose I have an array . Dividing numpy array by numpy scalar.1 (scalar, type float) The casting argument, however, determines the rules for what kind of data type casting is permitted as a result of an operation. arange, ones, zeros, etc. If your matrix is an MxN numpy array and your vector an N vector then you can simply do.What is a Matrix. A location into which the result is stored.I assume you wish to keep using the tuple, hence we convert the array back to a tuple.It will multiply each element in the Numpy with the scalar and return a new Numpy matrix with updated elements.product = A * numpy. numpy sort a structured array by two fields, ascending and descending orders.2 >>> # assuming by 'cumulative sum' you meant the 'reduced' .What I want to do is subtract a number from only the second column, say 10 for example.
26 Manualnumpy. These type descriptors are mostly based on the types available in the C .Scalar multiplication or dot product with numpy. dividing a row in a matrix using numpy.2>>> A[:,1] *= 5. Creating arrays from raw bytes through .To use in-place assignment, we have to select the Pandas column we want to multiply by the scalar value using either the dot notation or the bracket notation. If indices_or_sections is an integer, N, the array will be divided into N equal arrays along axis.array([2, 4, 6]) scalar = 3 # Use numpy. Multi Dimensional Numpy Array . Multiply columns 0 and 2 by 4 In: arr=([(1,2,3,5,6,7), (4,5,6,2,5,3), .
numpy structured array sorting by multiple columns
There are three main ways to perform NumPy matrix multiplication: np.) Replicating, joining, or mutating existing arrays.5, you can use the .comRecommandé pour vous en fonction de ce qui est populaire • AvisEach element in the matrix is multiplied by the scalar, which makes the output the same shape as the original matrix. Input arrays, scalars not allowed. repeat (a, repeats, axis = None) [source] # Repeat each element of an array after themselves. These can be scalars or N-dimensional arrays. if you want it in-place. sympy element wise division of vector . If provided, it must have a shape that matches the .2>>> # assuming by 'cumulati.You can use array slicing as follows for this - In [10]: arr=([(1,2,3,5,6,7), (4,5,6,2,5,3), (7,8,9,2,5,9)])In [11]: narr = np. For example, [[1, 2], [3, 4]] is a . Reversing a 1D array. minimum (x1, x2, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True [, signature, extobj]) = # Element-wise minimum of array elements.0python - Numpy, multiply array with scalar25 nov.multiply(array, scalar) . In the Hadamard product, the two . Sum of array elements over a given axis. outndarray, optional.If N = 1 then the returned object is an array scalar.
flip() function allows you to flip, or reverse, the contents of an array along an axis.comHow to Multiply Columns by a Scalar in Pandas | Delft Stackdelftstack. If provided, it must have a shape that the inputs broadcast to.multiply to multiply two same-sized arrays together. Numpy - Matrix multiplication.I'm trying to divide numpy array by numpy float64 type scalar.8To multiply a constant with a specific column or row: import numpy as np;X=np.^ where ufunc = multiply, self = b (ndarray, type int64, and other = 2.How to multiply each column vector with a scalar from an array? Example .divide(matrix_c, N*M) Just make sure N*M is a float in case your looking for precision. It accepts two arguments one is the input array and the other is the scalar or another NumPy array.reshape((3, 3)) >>> x2 = np.Use the iloc Operator to Multiply Columns by a Scalar in Pandas. If either a or b is 0-D (scalar), it is equivalent to multiply and using numpy.shape is (5,1), b.
repeats is broadcasted to fit the shape of the given axis.T] though that doesn't get you a numpy array as output, and it's transposed. Numpy sort ndarray on multiple columns. If you don’t specify the axis, NumPy will reverse the contents along all of the axes of your input array. The number of repetitions for each element.squeeze(pose_q) expectecd_q = np.