Ocaml map add
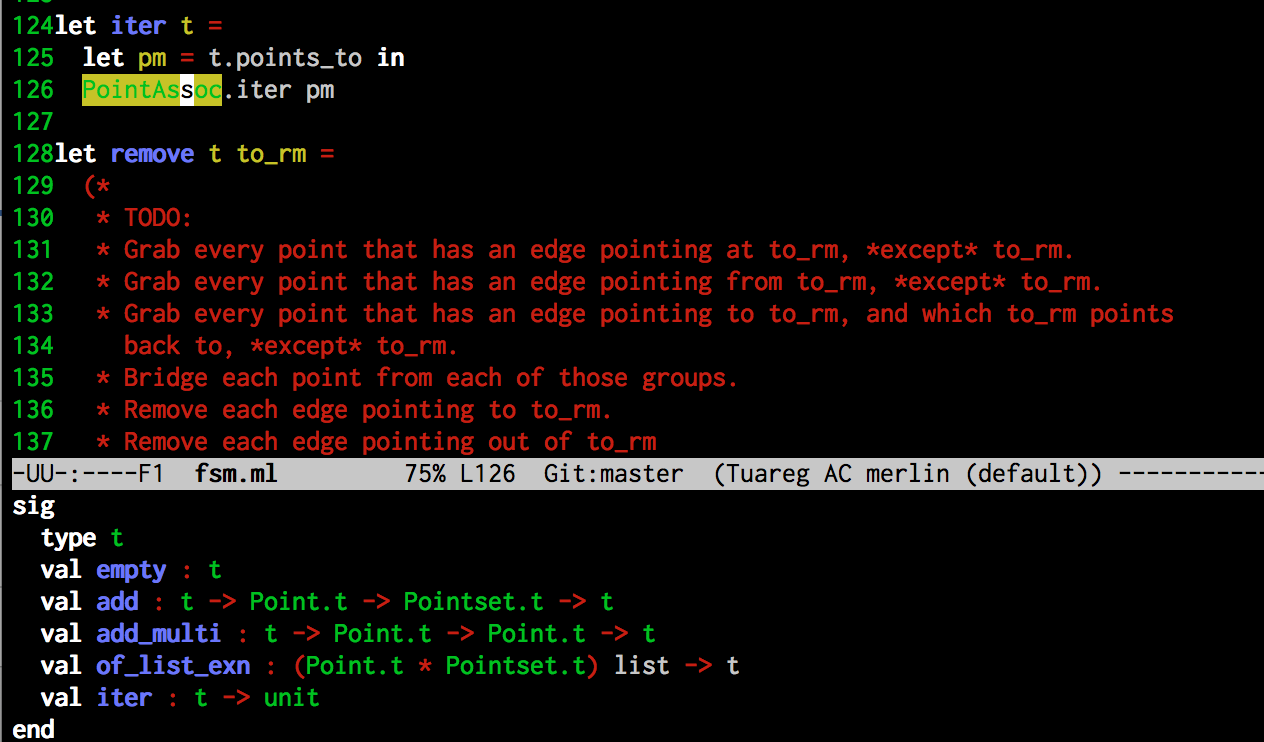
sig module type OrderedType = sig type t val compare : Map. There seem to be a few problems with your code. In particular, maps excel in situations where you need to keep multiple related versions of the data structure in memory at .t val add_to_list : Map.Return the length (number of elements) of the given list. The table grows as needed, so n is just an initial guess. Either None or a value Some v. Here are two functions we might want to .
OCaml library : Map
This chapter describes two program generators: ocamllex, that produces a lexical analyzer from a set of regular expressions with associated semantic actions, and ocamlyacc, that produces a parser from a grammar with associated semantic actions. This allows users to write more compact code. I want to print each value out with a plus sign between them .For best results, n should be on the order of the expected number of elements that will be in the table. # let data = data |> Stock. The first approach uses an intermediate representation using the Seq.getenv_opt : string -> string option does the same, except it returns None if the variable is not defined.t -> StringSet. These program generators are very close to the well-known lex and yacc commands that can be found in .Make functor in OCaml are immutable, so every time you add or remove an element from a set, a new set is created.bindings |> List.t val update : Map. Let's add in some additional data just for kicks.
About maps and NaNs
All elements of a list in OCaml must be the same type.Parameters: Maps. In natural languages, we only had a special symbol, &, for conjunction and not for disjunction, and .map under a changed perspective.OCaml, modules are the basic means of organising software.Make(String);; module MyUsers : sig. OCaml has floating-point values of type float.comRecommandé pour vous en fonction de ce qui est populaire • Avis
Map
This treatment of nan ensures . val empty : 'a t.append (to_alist lower_part) (to_alist upper_part) | .Learn OCaml - List. To make a set of strings: # module SS = Set. compare_lengths l1 l2 is equivalent to .(map hd rows:: transpose (map tl rows)) ;; val transpose: 'a list list-> 'a list list = # let (~:) = transpose;; val (~:): 'a list list-> 'a list list. Top-level modules must be files and are the units of compilation.
Create empty Map with given key and value types?
Understanding operators must be obvious to most readers, otherwise they do more harm . These definitions can be submodules, which allows the creation of hierarchies of modules.compare y0 y1 | c -> c end module PairsMap = Map. functor (Ord : OrderedType) -> sig type key = Ord. #letdata=Stock. 2023How to initialize Map from List in OCaml?30 avr. When I do like this: module StringMap = Map. Ce module permet de créer des « associations ». bind o f is f v if o is Some v and None if o is None. It wraps a function into a variant, and makes it possible to iterate some structure externally. type key = String. 2020In OCaml, is it possible to define Map in terms of Set?28 avr.Make (String) let vector_map = ref StringMap.Pattern matching can be used to deal with both cases, as with any other sum type. Before taking a look at Result.Well, let's create an empty map to begin with: #letdata=Stock. compare x y returns 0 if x is equal to y, a negative integer if x is less than y, and a positive integer if x is greater than y.t -> ' a list Map.addApple10data;;valdata:intStock. It makes little sense to make it look like C in this particular regard only.key -> ' a -> ' a list Map.map, let's think about List. The last element has number length a - 1 .key -> ' a -> ' a Map.key -> (' a option -> ' a option) -> .
Sets · OCaml Documentation
Il y a plusieurs moyens pour installer OCaml : Utiliser OPAM, un gestionnaire de paquet spécialisé pour OCaml.Map — OCaml Programming: Correct + Efficient + Beautiful. In OCaml, functions are treated as values, so you can use functions as arguments to functions and return them from functions.If we want a mapping from fruits to strings, we will have to create a different mapping. The following sections, starting at Function as Values, address functions. I found this bit of documentation which helped me figure out how to define my Map type: type rule =. The O(m) term is due to the need to calculate the length of the output maps. Raises Invalid_argument if o is None. We have now created a new map—again called data, thus masking the previous one—by .One should use Map for functions that access existing maps, like find, mem , add, fold, iter, and to_alist. Raises Invalid_argument if n is outside the range 0 to (length a - 1). This is just pretty printing.I said that because I'm not an OCaml-expert either. John Whitington's OCaml from the Very Beginning is a beginner-friendly guide to learning OCaml, a modern programming language.The function returns a list of the second type where every element is the result of calling the mapping function on an element of the first list. Every module has an interface, which is the list of definitions a .getenv : string -> string from the OCaml standard library queries the value of an environment variable; however, it throws an exception if the variable is not defined. The first element has number 0. I have a list of lists in OCaml returning [[7; 5]; [7; 3; 2]]. get a n returns the element number n of array a . Indeed, low memory usage is directly related to speed here. From the Float documentation:. OCaml是一种快速、简洁、而强大的应用程序开发语言--我想大家也许已经知道这一点了,而且已经安装了OCaml。.Return the length (number of elements) of the given array.
get o is v if o is Some v and raise otherwise. You can also write a.t takes both a string and a string set. It is more desirable to have similar symbols for both conjunction and disjunction.
comHow to initialize Map from List in OCaml? - Stack Overflowstackoverflow. An empty map is kind of boring, so let's add some data. The type of maps from type key to type ' a. Map # Map | OCaml Programming | Chapter 4 Video 2.map string_of_int [ 1; 2; 3; 4 ] #- [ . type account_rules =. some v is Some v. To sum up, a module is a collection of definitions wrapped under a name. Lists are built into the language and have a special syntax. Maps (映射,字典) Sets 集合.Make(String);; module SS : sig.OCaml handles lists internally, as shown in the penultimate expression, but displays them as the last expression.compare treats nan as equal to itself and less than any other float value.To create a Map I can do: # module MyUsers = Map.
Adding to a string map in OCaml
The empty list is written [].map that outperforms stdlib, Base, Batteries, and Containers, and is tail-recursive. But first, here is an example on how to do what you are trying to do: module StringMap . The optional ~random parameter (a boolean) controls whether the internal organization of the hash table is randomized at .Hash tables are not always the faster choice, though.Adding an Element to a Set. The type of the map keys. Here is a list of three integers: # [1; 2; 3];; - : int list = [1; 2; 3] Note semicolons separate the elements, not commas. type key = Ord. To add floats, one must use +.A list is an ordered sequence of elements. Data and Typing Type Conversion and Type-Inference. value o ~default is v if o is Some v and default otherwise.append ~lower_part ~upper_part with | `Ok whole_map -> Map. The first four sections address non-function values. val add_to_list : key -> 'a -> 'a list t -> 'a .The function returns a list of the second type where every element is the result of calling the mapping . No computation takes place between the two last steps. then only the type of the keys are given (String). It uses much less memory than all these implementations, except Batteries. OCaml is in few ways similar to C.martinklepsch November 27, 2017, 12:12pm 1. But now I’m not sure how I can create a map . | Regex of string. Hello, I’m struggling to understand how to use Base’s Maps.Counter-argument 1.
t -> int end module type S = sig . For functions that create maps, like empty , singleton, and of_alist, one .
Counter-argument 2.
OCaml library : Hashtbl
For example: MyMap.split t key returns a map of keys strictly less than key, the mapping of key if any, and a map of keys strictly greater than key.
Operators · OCaml Documentation
val compare_lengths : 'a list -> 'b list -> int.split to convert a list of pairs into a pair of lists (one containing the keys, one the values) and then snd to . Sets created with the Set.to_alist whole_map = List. add Orange 30 |> . The function Sys.OCaml library : Map.(n) instead of get a n.filter (fun key _ -> key = key_searched) map.Exceptions vs Options. Compare the lengths of two lists. On the other hand, the function Sys. type elt = String. val add : key -> 'a -> 'a t -> 'a t.add with type string -> StringSet. 本站为对OCaml 感兴趣者提供一套实用而详尽的教程。.map and Option. functor (Ord : OrderedType) -> sig.
Learn OCaml
Up and Running.
Utiliser le gestionnaire de paquet de votre plateforme ( FreeBSD . You can use bindings to retrieve the key/value pairs of a map and then further process them to extract the values.I offer for discussion a version of List. This tutorial introduces the relationship between expressions, values, and names. ' a t val empty : ' a t val add : key -> ' a -> ' a t -> ' a t val add_to_list .I’m writing my first “real” OCaml program, and I’m looking for a way to create a (global ref) empty Map, and at the same time specify the type of both the keys and the values. val is_empty : 'a t -> . Runtime is O(m + log n), where n is the size of the input map and m is the size of the smaller of the two output maps.