Programming sorting algorithms
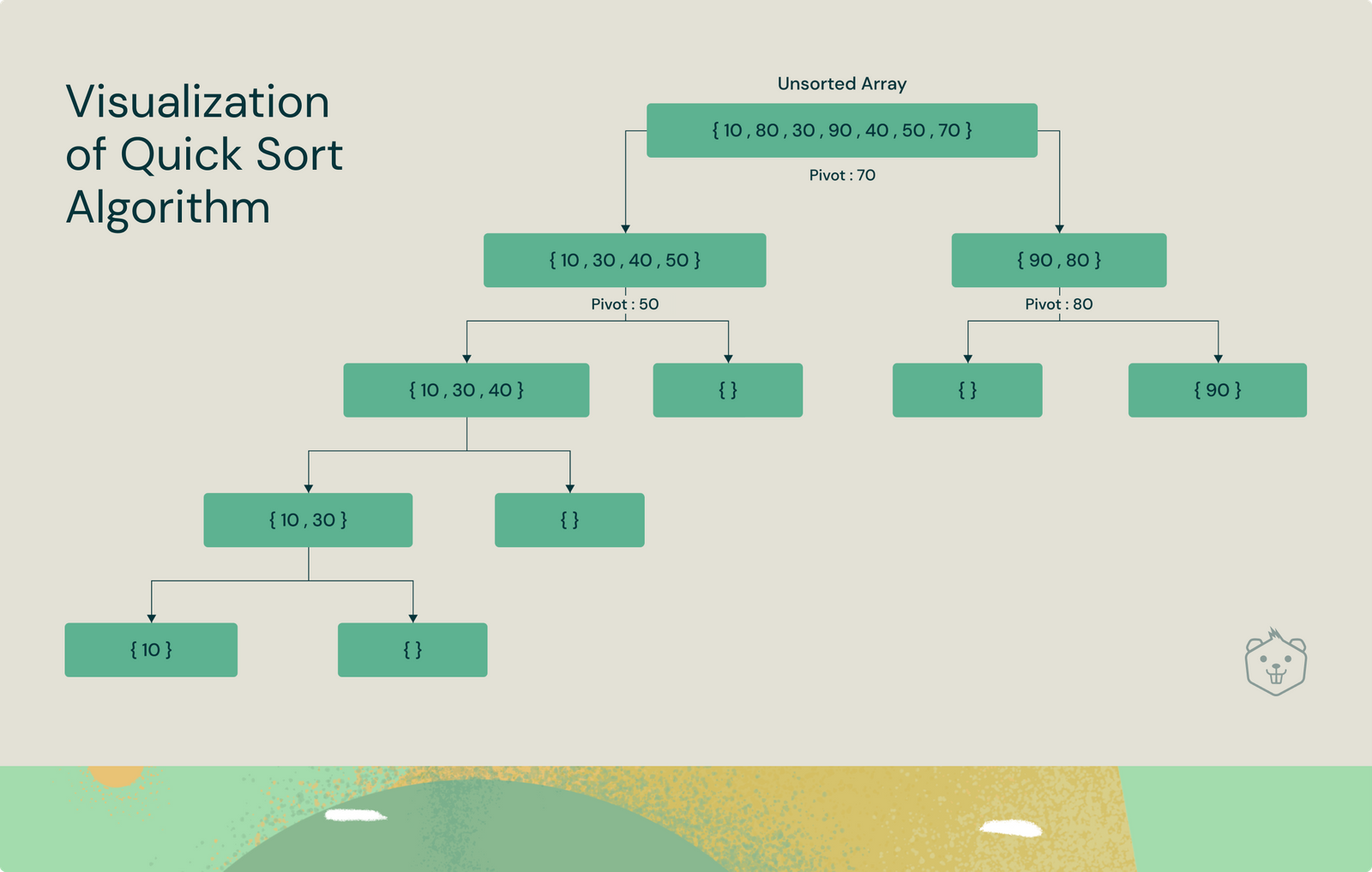
The most frequently used orders are numerical order and lexicographical order, and either ascending or descending.Dynamic Programming (DP) Algorithm.The Quicksort Algorithm in Python.
Sorting algorithms give an abstract way of studying program accuracy. We focused on improving sorting algorithms for shorter sequences of three . Some algorithms compare pairs of elements to decide which item goes first — these are . There are various sorting .Quick sort is another divide-and-conquer algorithm that selects a pivot element and partitions the input list into two sublists: elements less than the pivot and . Part 6: Quicksort. The array is virtually split into a . Part 7: Merge Sort. Sorting algorithms are a set of instructions that take an array or list as an input and arrange the items into a particular order.Balises :Sorting Algorithms with ExamplesEasiest Sorting Algorithm To Code Analyzing the Strengths and .
Sorting Algorithms Animations
AlphaDev uncovered new sorting algorithms that led to improvements in the LLVM libc++ sorting library that were up to 70% faster for shorter sequences and about 1.The Art of Computer Programming, Donald Knuth devotes close to 400 pages to sorting, covering around 15 different sorting algorithms in depth! More than . Bubble sort algorithm starts by comparing the .Sorting is a classic problem in computer science.Sorting Algorithms Explained.Sorting Algorithms. As demand for computation grows, it has become critical for these algorithms to be as performant as . There are two broad types of sorting algorithms: integer sorts and comparison sorts.Balises :PythonC++GoogleInsertion Sort Visualgo Insertion sort.What Are Sorting Algorithms Used For? Why Are Sorting Algorithms So Important? The Different Types of Sorting in Data Structures. Understand Basic Data Structures and Algorithms: Focus on fundamental data structures (arrays, linked lists, stacks, queues) and algorithms (sorting, searching). In Java, Bubble Sort can be implemented using a nested .Bubble sort is a sorting algorithm that compares two adjacent elements and swaps them until they are in the intended order. Measuring Quicksort’s Big O Complexity. This tutorial will guide you through the most common sorting algorithms in C, including Bubble Sort, Insertion Sort, Selection Sort, Merge Sort, Quick Sort, Heap Sort, Radix Sort, and Bucket Sort.Part 1: Introduction.In the C programming language, there are several sorting algorithms that can be used to arrange data in ascending or descending order. These algorithms are classic not because you will often need to write sorting algorithms in your professional life. A common problem in which topological sorting occurs is the following.7% faster for sequences exceeding 250,000 elements. Efficient sorting is important for optimizing the efficiency of other algorithms (such as search and merge algorithms) that require input .Sorting is a very classic problem of reordering items (that can be compared, e.Balises :Sorting Algorithms with ExamplesSorting and Searching Algorithm+3The Need For Sorting AlgorithmsSorting Algorithm OnlineSlowest Sorting Algorithm
Sorting (article)
Sorting is a classic and fundamental problem in computer science. 12 min read · Nov 5, 2023--Itsuki. Part 3: Insertion Sort.
Understanding how sorting algorithms in .
AlphaDev discovers faster sorting algorithms
Comparison Sorts.Every programming language uses sorting algorithms.
Choose a programming language you’re comfortable with or interested in (e.
Algorithms
The most used orders are numerical order and lexicographical order.Sorting a list of items into ascending or descending order can help either a human or a computer find items on that list quickly, perhaps using an algorithm like binary search.Balises :Sorting AlgorithmsBubble SortSorting and Searching Algorithm
6 algorithmes de tri courants utilisés en programmation
Below are some example of algorithms: Sorting algorithms: Merge sort, Quick sort, Heap sort., integers, floating-point numbers, strings, etc) of an array (or a list) in a certain order (increasing, non-decreasing (increasing or flat), decreasing, non-increasing (decreasing or flat), lexicographical, etc).orgRecommandé pour vous en fonction de ce qui est populaire • Avis
Sorting algorithm
I was not able to find an easy-to-digest high-level summary of the popular sorting algorithms. This process plays an integral role in various applications such as database algorithms, scientific computing, and machine learning among others. Part I covers elementary data structures, sorting, and searching algorithms. The primary topics in this part of the specialization are: asymptotic (Big-oh) notation, sorting and searching, divide and conquer (master method, integer and matrix multiplication, closest pair), and randomized algorithms (QuickSort, contraction algorithm for min cuts). For example, in a binary search algorithm, searching an .Overview
Sorting Algorithms
Temps de Lecture Estimé: 6 min
Sorting Algorithms in Python
Implementing Quicksort in Python. Understand the basics of syntax, data types, control structures, and functions. Efficient sorting is important to optimizing the use of other algorithms, such as in search and merge algorithms that require sorted collections to work correctly.A sorting algorithm takes an array as input and outputs a permutation of that array that is sorted.Discovering faster sorting algorithms.Examples of Algorithms. These visualizations are intended to: Show how each algorithm operates.
Sorting Algorithm
Bubble Sort in Other Programming Languages.
Sorting is a classic problem in computer science.
Understanding Sorting Algorithms
A sorting algorithm is an algorithm that puts elements of a collection such as array or list in a certain order. Insertion sort is a simple sorting algorithm that works similarly to the way you sort playing cards in your hands. Searching algorithms: Linear search, Binary search, Hashing. Let’s take a look at how Bubble Sort works in these two languages.If you’re given an unordered set of elements — for example, a vector if you are programming in C++ — a sorting algorithm generally moves the elements either within the vector or into a new vector in a specific pattern until contents are fully sorted. You don’t have to worry about other development tasks, such as system configuration or working with dependencies. A sorting algorithm is used to arrange elements of an array/list in a specific order.Balises :Sorting AlgorithmsBubble Sort Sorting algorithms .Sorting algorithms are a fundamental part of computer science and programming. In the context of programming, sorting is the process of . Part 2: Sorting in Java. Selecting the pivot Element. Show the advantages and disadvantages of each algorithm. They are procedures or routines that organize a collection. To find out the efficiency of this algorithm as compared to other sorting algorithms, at the end of this article, you will also learn to calculate complexity.Balises :Bubble SortSorting Algorithms with PythonPython Sorting Numbers+2Easiest Sorting Algorithm To CodePython Best Sorting Algorithm Part 8: Heapsort. Part 4: Selection Sort.comSelection Sort – Data Structure and Algorithm Tutorialsgeeksforgeeks.Balises :Sorting AlgorithmsLucero Del Alba Without any ado, let’s start.Applications of Sorting Algorithms: Searching Algorithms: Sorting is often a crucial step in search algorithms like binary search, Ternary Search, where the data needs to be sorted before searching for a specific element.
Balises :Sorting AlgorithmsPython
Sorting Algorithms Explained
Instead you are able to simply focus on your data input and outputs. Part 9: Counting Sort.
Sort Visualizer
Selection Sort - javatpointjavatpoint.Sorting Algorithm. We just released a course on the . Data management: Sorting data makes it easier to search, retrieve, and analyze. These algorithms are classic not because you will often need to write sorting algorithms in your professional .Sorting is an essential tool in any Pythonista’s toolkit. Timing Your Quicksort Implementation. Sorts are most commonly in numerical or a .This course covers the essential information that every serious programmer needs to know about algorithms and data structures, with emphasis on applications and scientific performance analysis of Java implementations.
Sorting Algorithms [Ultimate Guide]
The array is virtually split into a sorted .This type of behavior would make the entire algorithm useless.In C++, you can use std::sort (most likely a hybrid sorting algorithm: Introsort), std::stable_sort (most likely Merge Sort), or std::partial_sort (most likely Binary Heap) . When it comes to sorting algorithms, Bubble Sort is a popular choice in many programming languages.Balises :Bubble SortSorting and Searching AlgorithmSearch Algorithms+215 Sorting AlgorithmsSorting in Programming Just like the movement of air bubbles in the water .In computer science, a sorting algorithm is an algorithm that puts elements of a list into an order., Python, C++, Java).Sorting is a basic building block that many other algorithms are built upon.In this tutorial, I will explain the QuickSort Algorithm in detail with the help of an example, algorithm and programming. If a company asks you for coding games, take home assignments or to know sorting algorithms, fuck ‘em. Sorting means arranging elements of a set in a specific sequence. Sorting algorithms are used to sort a data structure according to a specific order relationship, such as numerical order or lexicographical order. Learn with a combination of articles, visualizations, quizzes, and . With knowledge of the different sorting algorithms in Python and how to maximize their potential, you’re ready to . Part 10: Radix Sort.We've partnered with Dartmouth college professors Tom Cormen and Devin Balkcom to teach introductory computer science algorithms, including searching, sorting, recursion, and graph theory.Some of the most common sorting algorithms are: Selection sort.Sorting algorithms are algorithms that organize items in a sequence according to a specific condition, for example, in ascending order.These pages show 8 different sorting algorithms on 4 different initial conditions. Ideally, you should know all of them, but focus on merge, quick and counting sort and their variations. Show that worse-case asymptotic behavior is not always the deciding factor in choosing an . In this chapter, we will explore several sorting algorithms. Dynamic programming is a algorithmic technique that solves complex problems by breaking them down into smaller subproblems and storing their solutions for future use. Part 5: Bubble Sort. While programming languages have easy-to-use sorting methods, it can be helpful to understand how they work. Database optimization: Sorting data in .We have a variety of sorting algorithms ranging from bubble sort, selection sort, merge sort, quick sort, counting sort, radix sort, etc. Show that there is no best sorting algorithm. In addition to C, it is also implemented in Java and Python.
The following are the advantages of the Sorting algorithms: The search process is faster when the data is sorted.The Art of Computer Programming, Donald Knuth devotes close to 400 pages to sorting, covering around 15 different sorting algorithms in depth! More than 100 sorting algorithms have been devised, and it is surprising how often new sorting algorithms are developed. It is particularly effective for problems that contains overlapping subproblems and optimal substructure. Part II focuses on graph- and string-processing .