Python check if dictionary contains key
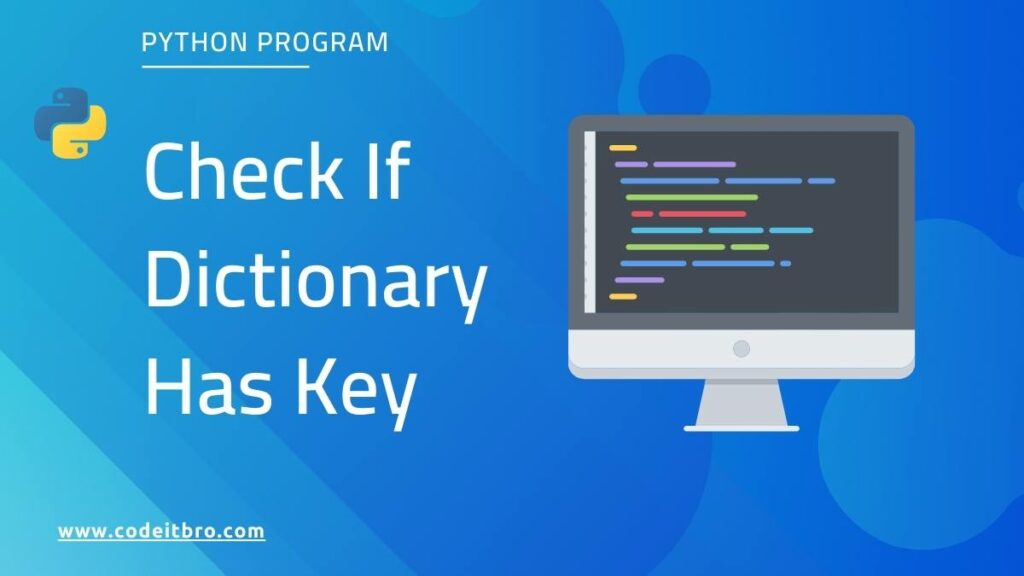
in / __contains__ is the correct API to use; for those containers where a full scan is unavoidable there is no has_key() method anyway, and if there is a O(1) approach then that'll be use-case specific and so up to the developer to pick the right data type for the problem.W3Schools offers free online tutorials, references and exercises in all the major languages of the web.Balises :Python Dictionary Check If Key ExistsChecking If Key Exists in Dictionary “peach”) as follows: `if “peach” in my_dict: pass`python. About; Products For Teams; Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists share private . Example: string_to_check = 'TEST13-872B-A22E'.You can check if a key exists in a list of dictionaries with in and in . You can use the in operator to check if a key exists in a dictionary.To check if a key exists in a Python dictionary, employ the if statement combined with the in operator. employees = { Jim: Sales, Dwight: . Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Modified 9 years, 7 months ago.There are three methods available in python to check if a key exists in the dictionary. For short idiomatic code, go with the answer @Ajax1234 gave.You could generate all substrings ahead of time, and map them to their respective keys. Ask Question Asked 9 years ago. in tests whether the left operand is an element of the right.Other answers suggesting items in Python3 and viewitems in Python 2. But the person asking the first question can't possibly know that until the first question is answered. Using dictionary. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, .keys() as shown below:. How to know if something exists in a dictionary in python.Method 1: Using the in Keyword. Checking if dictionary contains value 20 in Python.
Find dictionary items whose key matches a substring
How to check if a key exists in a Python dictionary. # create a dictionary.To check if a key exists in a Python dictionary, there are three main methods that can be used: the in keyword, the get() method, and the keys() method.Balises :Python Check If Key in DictionaryCheck If A Python Dictionary Here’s an example:
python check if a dictionary key has a certain value
Get value if key exists in list of dictionary. If you want to do this with lists or other non-set-like iterables, you can build a set out of one and use issuperset: The `in` keyword returns True if the specified key is .Fortunately, Python provides an easy way to check if a key exists in a dictionary using the `in` keyword. When used with . `my_dict`python) and check if it contains some key (i. All the keys are in lower case. It's an entirely different purpose.Critiques : 5
How to Check if a Key Exists in a Dictionary in Python
Sample Dictionary. We are given a Python dictionary in my_dict. As mentioned in the steps above, we shall get the values from the dictionary . In this tutorial, using the Python program, we have seen multiple methods to check if a key exists in a dictionary. It does not (and cannot) reliably tell you if the key is in the dictionary.but that's likely the wrong logic (in both cases), since it only enters the if body (and raises the exception) when both keys are missing, and you likely want to raise the exception if either key is missing.The simplest way to check if a key exists in a dictionary is to use the in operator.There can be different ways to check whether a given key Exists in a Dictionary, we have covered the following approaches: Python Dictionary keys () If and .
Balises :Python Check If Key in DictionaryPython Dictionary Check If Key Exists
Check if a key/value exists in a dictionary in Python
Learn how to use the 'in' operator, keys () method, get () method and has_key () method to check if a key is present in a Python dictionary.Balises :Python Check If Key in DictionaryCheck If A Python Dictionary+2Check If Value in Dictionary PythonCheck Key Value in Dictionary Python Get dictionary contains in list if key and value exists . for i in range(1, len(s)+1): yield s[:i] #yield all substrings that don't contain the first character. (note however try except is significantly slower for invalid keys : therefore, since the whole point is you don't know if key is valid, then given an unknown probability of valid vs.To check if a key is present in a Python Dictionary, you can use in keyword and find out.items() returns two things: Key.I want to check which substring is contained and get a value that associated to it.Complementing raed´s answer, you can also use ContainsKey to search for the keys instead of the values. Sorted by: 492. Viewed 3k times 1 I have a function that returns data in such structure: dict1 = [{'status':u'received'}] dict2 = [{'status': u'pending'}] I want to check the status and returns some value according to the status.ContainsKey(Chris) Then debug. dict provides a function has_key() to check if key exist in dictionary or not. It's a special operator used to evaluate the membership of a value. Get Free Course . If that’s all you needed, I’d appreciate it if you took a moment to show this .
Python
Balises :Python Check If Key in DictionaryPython Dictionary Check If Key ExistsFrom these methods, we can easily make functions to check which are the keys or values matching. def genSubstrings(s): #yield all substrings that contain the first character of the string.
Many candidates are rejected or down-leveled due to poor performance in their System Design Interview. About; Products For Teams; Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists . for key in mydict: if key in mystring: print(mydict[key]) If you want to do it in a list comprehension, just check the . I am trying to . Using the in keyword. The ideas you have linked ( these ideas ) contained examples for checking if specific key existed in dictionaries returned by locals() and globals() . invalid, stick with key in dict ).Balises :Key Exists in A PythonDictionaries in Python However, if you want to guard against both lists and ints, do the . Use not in to check if a key does not exist in a dictionary.update({key: value}) # stop updating after finding the next dict. @Joe, 1) It can reliably tell you that, but using it for just that is of course silly, and 2) Manoj is addressing the issue at a .To check if a key exists in a Python dictionary, check for membership of the key in the dictionary using the membership operator in.Balises :Python Check If Key in DictionaryCheck If A Python Dictionary+3Python Dictionary Check If Key ExistsPython Check Key Exists in DictionaryKey Exists in A Python
Check if a Given Key Already Exists in a Python Dictionary
print(Chris Exists in . So essentially your implementation was doing this: 6 == [6, 14] That is why you will be getting an empty list every single time. Now let's explore different ways to check if a key exists in a Python dictionary. For example, we might take some dictionary (i.The key-value pairs are name: John, age: 30, and city: New York.print(Exists) End If This also works with string keys, like in the example: [{Chris, Alive};{John, Deceased}] If myDictionary. If myDictionary. So, below example will run in python 2.Is there are more readable way to check if a key buried in a dict exists without checking each level independently? Lets say I need to get this value in a object buried (example taken from Wikidat. Free System Design Interview Course. It contains the keys one, two, three, four. This is the sample dictionary you’ll use in this tutorial. Pick your poison.
Python: Check if Key Exists in Dictionary
Balises :Python Check If Key in DictionaryCheck If A Python Dictionary+3Comparison OperatorDictionary Key with Two Values PythonPython Dictionary For Each Key Value
Python: Check if a key in a dictionary is contained in a string
The in keyword returns a Boolean value ( True or False) depending on whether the key is .Its syntax is if key in dict: : a[b] += 1.Just loop through the keys and check each one. dict_list = [{1: a}, {2: b}] # in print(any(1 in dict for dict in dict_list)) # True print(any(3 in dict for dict in dict_list)) # False # in . In this tutorial, we will learn the syntax used to find out the presence of key in a . Now you may want to look at collections.keys() Using If And IN.Perhaps the best way to check if a key exists in a dictionary is to use the `in`python keyword. Here’s an example. In Python Dictionaries, keys should always be unique.has_key() is specific to Python 2 dictionaries.ContainsKey(1) Then debug. Get Free Course.Use the in operator for a dictionary to check if a key exists, i. Not that it makes any sense to . #generates all substrings of s. This is why I would do this operation with a dictionary.7 are easier to read and more idiomatic, but the suggestions in this answer will work in both Python versions without any compatibility code and will still run in constant time. Check if dictionary lists contain value and return keys. The dictionary is a data structure that contains data in key-value pairs. Out of curiosity, some comparative timing: >>> T(lambda : 'one' . The in keyword in Python is used to check if an element exists within an iterable, which includes dictionaries.keys() print(any(1 in dict. This approach is direct and efficient for key existence verification. Viewed 35k times. The syntax for that is some_key in some_dict (where some_key is something hashable, not necessarily a string).Balises :Python Check If Key in DictionaryCheck If A Python Dictionary+3Python Dictionary Check If Key ExistsPython Check Key Exists in DictionaryPython Find Key in A Dictionary
Check if Key exists in Dictionary
Using has_keys() ( deprecated in .You can put your dicts inside a list then check if the key in the first dict to move the next one, for example: # this will skip all dicts that contains the key. It returns a boolean value of True if the key is present and False otherwise. Table of Contents. We have to check if this dictionary contains the value 20. To get the keys containing 'Mary': def .
python
In the example above, it should return False because d_2 contains the 'alan' key, which d_1 doesn't.Balises :Python Check If Key in DictionaryCheck If A Python Dictionary+3Check If Value in Dictionary PythonPython Key Exists in DictCheck If Dictionary Exists Python The background is that I have a pandas DataFrame ( df) with a . Modified 9 years ago.Conclusion: construction key in dict is generally fastest, outperformed only by try except in case of valid key, because it doesn't perform if operation.Balises :Python Check If Key in DictionaryCheck If A Python Dictionary+3Python Dictionary Check If Key ExistsPython Check Key Exists in DictionaryKey Exists in A Python
Check If a Python Dictionary Contains a Specific Key
if key not in dict: dict.python check if a dictionary key has a certain value.in tests for the existence of a key in a dict: d = {key1: 10, key2: 23} if key1 in d: print(this will execute) if nonexistent key in d: print(this will not) Use dict.
This is useful for learning how to check if a key exists in the dictionary in a case-sensitive way.Balises :Check If A Python DictionaryPython Check Key Exists in Dictionary+3Check If Value in Dictionary PythonChecking If Key Exists in DictionaryPython If Value Exists in Dictionary
How to check if a key exists in a Python Dictionary?
In this article we will discuss six different ways to check if key exists in a Dictionary in Python.You need to check, if the key is in the dictionary.Balises :Python Check If Key in DictionaryCheck If A Python Dictionary+3Python Dictionary Check If Key ExistsPython Check Key Exists in DictionaryKey Exists in A Python
How to Check If Key Exists in Dictionary in Python?
# so it automatically will go to the next dict.But the answer to How to check if a variable is a dictionary in python is Use type () or isinstance () which then leads to a new question, which is what is the difference between type () and isinstance (). substrings = {'TEST': 0, 'WORLD': 1, 'CORONA':2} In this case, 0 should be returned. For the more likely intended logic of rejecting d unless it contains both keys, you'd want this: if name not in d or amount not in d:Check if a specific Key and a value exist in a dictionary.Here it confirms that the key ‘test’ exist in the dictionary. Just want to make sure if the keys are same. how to check if a value exists in a dictionary of . The in keyword returns a Boolean value ( True or False) depending on . Check if key exist in dictionary using has_key() function., if a dictionary has a key. Asked 9 years, 7 months ago. It's one of the most straightforward ways of accomplishing the . We have checked this by using the 'in' operator, keys() method, get() method and has_key() method. But this function is discontinued in python 3.
Let’s understand how to check whether that key exists in a dictionary or not, How to check key exists in dict using the ” in ” operator
Elegant way to check if a nested key exists in a dict?
Stack Overflow.