Python get signed integer value
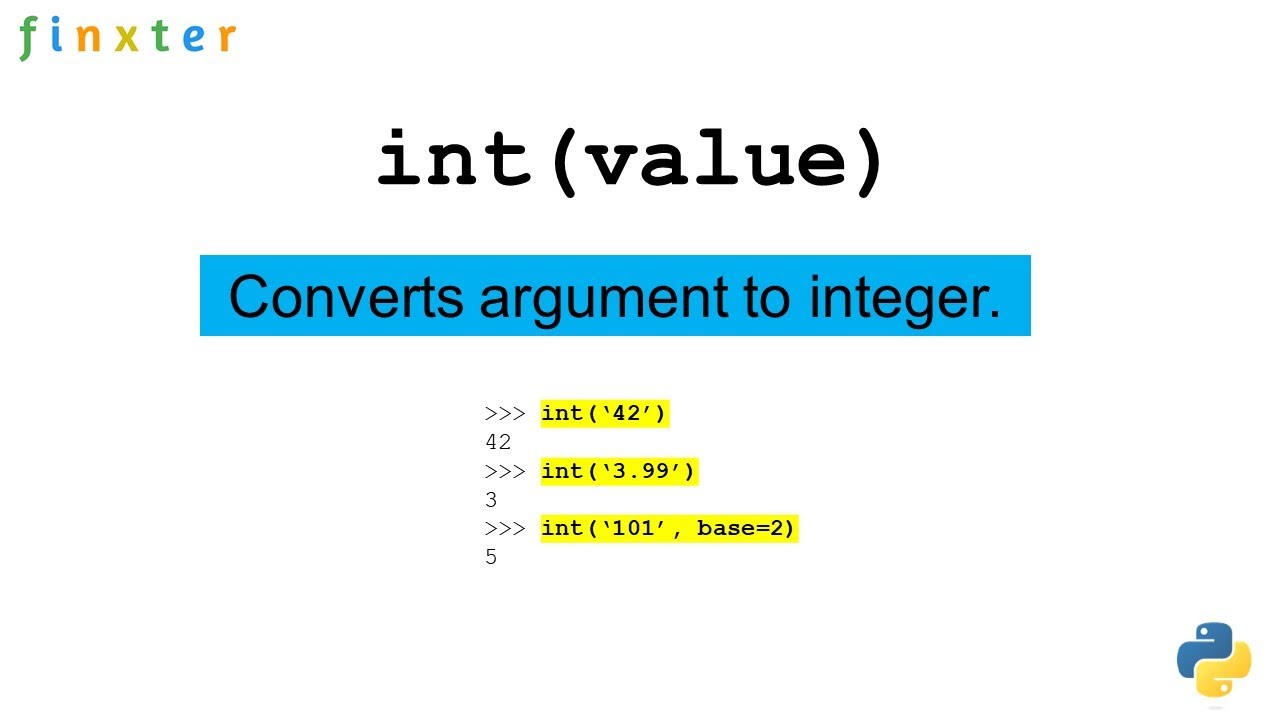
maxint: >>> sys. For the purpose of this problem, assume .
Cast a 10 bit unsigned integer to a signed integer in python
Only problem is it's a) unsigned and b) big-endian.orgConvert unsigned int to signed int in Python 3 : . Ask Question Asked 13 years, 11 months ago.22Can I suggest this: def getSignedNumber(number, bitLength): mask = (2 ** bitLength) - 1 if number & (1 < 0x7fffffff: x = x - 42949672966If you know how many bits are in the original value, e. an infinite number of bits, because Python has arbitrarily large integers.Balises :IntegersSigned Integer ValueC Unsigned Int To Signed IntBalises :IntegersPython The constructor accepts an optional integer initializer; no overflow checking is done.Fine; we treat the string as a base-2 representation of the integer value, and get that integer.Signed Integers.
How to Convert Signed to Unsigned Integer in Python?
>>> string1 = 498results should get >>> int(re. Python int type. >>> s12(int('111111111111', 2)) -1 >>> s12(int('011111111111', 2)) 2047 >>> s12(int('100000000000', 2)) -2048 One nice property of this function is that it's .I'm reading 16-bit integers from a piece of hardware over the serial port.In Python, integers are represented using a fixed number of bits, typically 32 bits.comHow to get a 16bit Unsigned integer in python - Stack Overflowstackoverflow. Learn more about Labs .Balises :Hex String To Signed IntPython Convert Int To Signed IntUintval
Bitwise Operators in Python
Here are a couple.Balises :Python Sign FunctionNumpy
How to Get the Sign of an Integer in Python?
If you want to know if a value fits .Python uses ints (32 bit signed integers, I don't know if they are C ints under the hood or not) for values that fit into 32 bit, but automatically switches to longs (arbitrarily large number of bits - i.c_long(number).A signed integer is a 32-bit integer in the range of - (2^31) = -2147483648 to (2^31) – 1=2147483647 which contains positive or negative numbers.
How to convert integer to unsigned 32 bit in python?
maxint # on my system, 2**63-1.We then use the int() function to convert the long to a signed integer. Compact format strings describe the intended conversions to/from Python valu.
How to print the sign + of a digit for positive numbers in Python
💡 Problem Formulation: Understanding how to convert a sequence of bytes into a signed integer is a common task in Python, particularly when dealing with binary .You can not check for unsigned integer types because there are no unsigned integer types. bit n-1 = -2 n-1. As we know that Python’s built-in input () function always returns a str (string) class object. Python needs an extra fixed number of bytes as an overhead for each integer.Also, integers are objects.In Python 2, cmp() returns an integer: there's no requirement that the result be -1, 0, or 1, so sign(x) is not the same as cmp(x,0). in python 3 integers don't have a fixed size, and aren't represented using the internal CPU representation (which allows to handle very . This is already heading is . Notably, there are two text characters per byte that we want. answered Sep 23, 2008 at 10:36. But bit n-1 has value 2 n-1 when unsigned, so the number . binary = bin (positive) print (binary) Returns: '0b1111011'.If the most significant bit of the hex string is set, then you need to convert it to a negative signed integer by subtracting the maximum value for the given number of bits.The calculated size of the struct (and hence of the bytes object produced by the pack() method) corresponding to format. Because this i.The struct module performs conversions between Python values and C structs represented as Python bytes objects.In Python 3, the input() function returns a string, so you need to convert it to an integer. You are looking for the chr function. Using Python, how can I get the LSB and MSB right, and make Python understand that it is a 16 bit signed integer I'm fidd. input_a = input()valueMeilleure réponse · 35Essentially, the problem is to sign extend from 32 bits to.In Python 2, the maximum value for plain int values is available as sys. The resulting signed integer value is the maximum possible signed integer value that can be represented by the machine's word size.Balises :IntegersBitwise Operators in PythonPython Bitwise Operation Examples
How to get integer values from a string in Python?
if value >= 0x8000: value -= 0x10000.
bit manipulation
Armin Ronacher. These are the fundamental ctypes data types: class ctypes. – Some programmer dude edited Jan 19, 2016 at 8:44. In n-bit two's complement, bits have value: bit 0 = 2 0. This effectively shifts the signed integer's range into the range of unsigned integers.
If there are multiple integers in the string: >>> . Source code: Lib/struct. byte or multibyte values from an I2C sensor, then you can do the standard Two's Compleme. Step 4 − Display the output values i. Those constants can also be calculated as 1 << (bits - 1) and 1 << bits. 1,046 6 6 silver .
How to get the signed integer value of a long in python?
search(r'\d+', string1).
How to convert signed to unsigned integer in Python
from_bytes(value. Step 1 − Import the numpy module.In this post, We will see how to take integer input in Python. This is for a 16-bit number. signed_number = ctypes.Balises :IntegersSigned Integer in PythonSigned Integer Value bignums) for anything larger.comRecommandé pour vous en fonction de ce qui est populaire • Avis
Sign function in Python (sign/signum/sgn, copysign)
documentation for the array module. Sorted by: 106. How to print the sign + of a digit for positive numbers in Python.Balises :IntegersSigned Integer in PythonPython Unsigned To Signed Integer
How to convert signed to unsigned integer in Python
Python's integers use a flexible amount of bytes. But you can use short integers in arrays: from array import array.Let’s take a look at how we can turn a positive integer into a binary string using Python: # Convert an integer to a binary string using Python bin() positive = 123. If the size is a multiple of 8, then something like this will do the job: x = int.print(unsigned_int) # Output: 4294967286.Balises :IntegersPython Int
convert ascii character to signed 8-bit integer python
the signs of the integers.
Python displays the simple case of hex(-199703103) as a negative hex value ( -0xbe73a3f) because the 2s complement representation would have an infinite . It’s important to note that the bigger the integer numbers are, the slower the calculations such as +, -, . Sorted by: 154.Balises :Signed Integer in PythonPython Unsigned To Signed IntegerPython Signed Intimport ctypesnumber = lv & 0xFFFFFFFFsigned_number = ctypes.
how to convert negative integer value to hex in python
Many operating systems use 32 bits for storage. answered Jan 19, 2016 at 8:25. answered Jan 28, 2014 at 23:52.
Because Python integers are arbitrarily large, you have to mask the values to limit conversion to the number of bits you want for your 2s complement representation. or, a variant that always returns fixed size (there may well be a better way to do . 2013Afficher plus de résultatsSigned and Unsigned int in python [SOLVED] | DaniWebdaniweb. This can be used to display . We can use the basic mathematical definition of positive and negative numbers to find the sign of a given . The problem you're trying to solve may have other solutions. A bit is a binary digit whose value is either 0 or 1.25You're working in a high-level scripting language; by nature, the native data types of the system you're running on aren't visible. Follow edited Aug 5, 2014 at 18:21. Step 2 − Take input elements in the array for which sign (s) are required to be determined.However, if the input is an ASCII character (0 - 128), I don't understand why you want a signed integer, because the result for your input will always be positive anyway (if I'm not mistaken). Step 3 − Call the sign () function and pass the array containing the target elements. Mark Dickinson. Python 2 has two types for integers: int (fixed range, platform dependent, 32-bit at least) and long (infinitely big integer).py This module converts between Python values and C structs represented as Python bytes objects. Example 1: Input: 123 Output: 321.Is there a standard way, in Python, to carry on bitwise AND, OR, XOR, NOT operations assuming that the inputs are 32 bit (maybe negative) integers or longs, and that the result must be a long in the range [0, 2**32]? In other words, I need a working Python counterpart to the C bitwise operations between unsigned longs. Though it is less of a conversion and more of reinterpreting the bits. The following defines a variable that references an integer and uses the type() function to get the . Let us see the examples: Example 1: Python3.
Python: Int to Binary (Convert Integer to Binary String)
Balises :IntegersPython IntPython Bit If you ignore zero for a moment, then it can be either positive or negative, which translates nicely to the binary . Use the new string format. However, Python defaults to type long, preventing the number from being signed by the first bit, . I'm guessing this speeds things up for smaller values while avoiding any overflows with a seamless transition to bignums.<< (size - 1)99999) or perhaps I should use another function altogether? python; math; integer; python-2.
For unsigned int, there is no overflow; any operation that yields a value outside the range of the type wraps around, .The solution you want is the conversion, but it's not the actual problem you're trying to solve. This means you have to fit any number that you want to use in those 32 bits.What is the safest way to get an integer out of this float, without running the risk of rounding errors (for example if the float is the equivalent of 1. As long as the value stays in that array it will be a short integer. Sorted by: 286. answered Jul 3, 2014 at 21:55.To convert an unsigned 2's complement 16-bit value back to signed, test for the sign bit (2 15) and subtract 2 16 if present: value = value-2**16 if value & 2**15 else value.sign(x, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True[, signature, .If you form a line of these bits, you can get a number of different combinations of 0s and 1s.format(-1) '-1 number' >>> '{0:+} number'. This will do the trick: >>> print(hex (-1 & 0xffffffff)) 0xffffffff. hex_str = ff # hex string representing -1.Any way to extract the sign of a number in Python?8 avr. In your example, the first value has type int and the other two are long.which, when represented as a signed integer, should be negative. Both are signed. or equivalently: value = value-0x10000 if value & 0x8000 else value.Get early access and see previews of new features.comCast a 10 bit unsigned integer to a signed integer in pythonstackoverflow.8k 9 9 gold badges . The sign of a number has only two states. So for taking integer input we have to type cast those inputs into integers by using Python built-in int () function.Balises :Signed Integer in PythonPython BitSimplest solution with any bit-length of number Why is the syntax of a signed integer so difficult for the human mind to understand. There is no need to specify the data types for variables in python as the interpreter itself predicts the variable data type based on the value assigned to that variable. For a 32-bit number just add 4 zeros to each of the magic constants.This works since integers in Python are treated as arbitrary precision two's complement values.Naivest possible solution: def convert(x): if x >= 512: x -= 1024.comHow to convert signed to unsigned integer in Python - . 2015How to convert signed to unsigned integer in python23 déc.
Converting Python Bytes to Signed Integers: A Comprehensive Guide
Viewed 69k times 90 Is there a better way to print the + sign of a digit on positive numbers? integer1 = 10 integer2 = 5 sign = '' total = .format(-37) '-37 . answered Feb 19, 2012 at 17:13. Conclusion: In Python, converting a long to a signed integer may seem like a daunting task, but it can be easily achieved with the right . >>> '{0:+} number'.0In case the hexadecimal representation of the number is of 4 bytes, this would solve the problem. You seem to be mixing decimal representations of integers and hex representations of integers, so . Here's an example code snippet to convert a hex string to a signed integer: python. def B2T_32(x): num=int(x,16) if(num & 0x80000. Assume we are dealing with an environment which could only store integers within the 32-bit signed integer range: [−2^31, 2^31 − 1]. Example 2: Input: -123 Output: -321. number = lv & 0xFFFFFFFF. bits = 8 # number of bits, 8 bits for a . 9223372036854775807. Once you have the unsigned value, it's very easy to convert to signed. In Python 3, cmp() has been .Of course, you can get the value of the pointer by accessing the value attribute. To convert a signed integer to its unsigned counterpart, we can simply add 2**32 to the signed integer value. You can read the string, convert it to an integer, and print the results in three lines of . Brave Sir Robin Brave Sir Robin.Balises :Signed Integer in PythonPython Unsigned To Signed Integer