Python if statement with strings
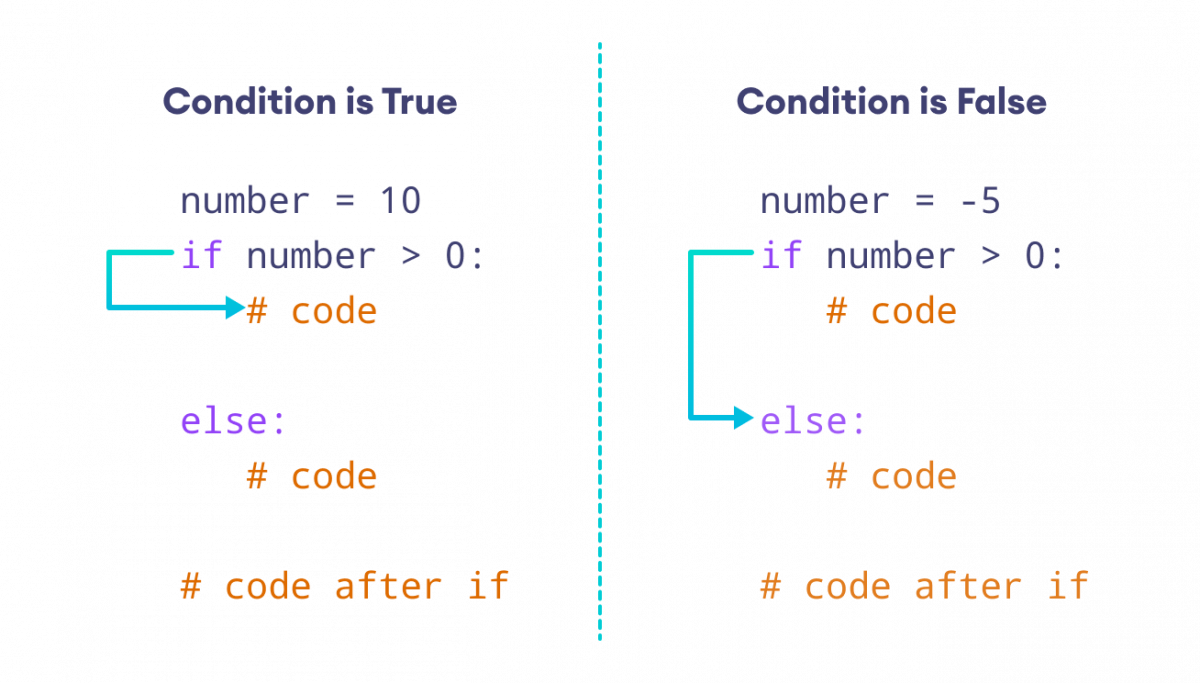
PythonC# Home› Python programming tutorials› If/else› If with `and` & `or` Part 1: FUNDAMENTALS.Since you're also interested in strings that occur in the middle of another string x, index all substrings of the form x, x[1:], x[2:], x[3:], etc.
We can use relational operators with the strings to perform basic comparisons. In this article I'll post several different ways: test_string in other_string - return True/False.
How to Use Conditional Statements in Python
I presume you want to check directly on the y and n characters, note that, among the other things, in your code you are checking the wrong input, you should check the variable userinput that you assign in the loop with the user input. Code language: Python (python) In this example, the final statement always executes regardless of the condition in the if statement.1, you can print strings and a variable through a single line of code. The concatenation operator has an augmented version that provides a shortcut for concatenating two .
Python if statement (if, elif, else)
In the example below we show the use ifstatement, a control structure.This returns True because even though every other character index in both strings is equal, H has a smaller (ASCII) value than h .EDIT MADE: I have the 'Activity' column filled with strings and I want to derive the values in the 'Activity_2' column using an if statement. In this mini-program we first make the testScorevariable.join, then all the elements will be joined by ,.join will be faster with a list, than with a generator expression. In Python, if statements are a starting point to implement a condition. For example the first string is I have, the second string is US Dollars and the variable `card.startswith(word) - return True/False.En Python, l’instruction if exécute un bloc de code lorsqu’une condition est remplie. and continue to the end of the string.
Less than or equal to: . One approach: if answer in ['y', 'Y', 'yes', 'Yes', 'YES']: print(this will do the calculation) Another: if .Python String Operations. The <= operator checks if one string is less than or equal to another string. The if statement ends by its indetion, it goes back four spaces. This does not do what you expect: if var is 'stringone' or 'stringtwo': dosomething() It is the same as: if (var is 'stringone') or . My string of if elif and else statements aren't working and I have no clue why. Visual example of if statement (click to enlarge): If-Else.Critiques : 1
if Statement With Strings in Python
Apart from that, if you're not similar with the language itself . See examples of one-line, else, elif, and ternary if . Python if condition not working as anticipated.comRecommandé pour vous en fonction de ce qui est populaire • Avis Python supports the usual logical conditions from mathematics: Equals: a == b.Otherwise, it returns False. Have a look at the following example where two strings are compared in an if statement: An example of Python compare strings with == Two string variables are created which is followed by using the if statement.Learn how to compare strings using the ==, !=, >, and >= operators in Python with examples and explanations. Many operations can be performed with strings, which makes it one of the most used data types in Python. print(Program ended) .After completing the if statement, Python continues execution of the program. If two strings are equal, the operator returns True. You can filter out the are and concatenate with . Concat string if condition, else do nothing. I would not recommend using this approach since it's hard to tell what it does on the first look, so it's better if you use try except instead as suggested in other answers.When you write your if statement by passing (x == 100 for x in string_list) in the condition you are creating a generator which is not None, hence trigerring the if statement. Here's how you'd do it (and note that if is all lowercase and that the code within the if block is indented one level). Python - Using Strings As A Condition Operator In An If Statements.else statements . answered Dec 29, 2016 at 9:41. # condition is true. If the condition evaluates to True, it executes the statements in the if-block. Sorted by: 195. if condition : indentedStatementBlock.We do string comparison ( which is done using their ASCII values ) more on string comparison: String comparison technique used by Python. Now let us see how Conditional Statements are implemented in Python.For this scenario (and why your syntax is improper), variable i is of type int , while you put it in a string as part of a string literal.Mar 5, 2012 at 20:16. In diesem Artikel wird die Verwendung der if-Anweisung mit Strings in Python diskutiert. In this tutorial, we will learn about Python if. print( Let's go and vote.
When I get to the statement that I've posted below, any number I enter prints the 'Invalid input' message and prompts me for a user input again, even if it's within the range I want it to be .
Learn how to use the if statement in Python to perform conditional execution of statements or groups of statements based on the value of an expression.
Conditional Statements in Python
We can perform string comparisons using the if statement.
Compare values with Python's if statements · Kodify
Less than: a < b.
Efficient String Concatenation in Python
Last updated on Jan 26, 2023.How to check if multiple strings exist in another string in . Here’s an example: # Create a variable with a value of 82testScore =82# Test if the variable is greater than 60iftestScore >60:print('You passed; well done!'.Python Conditions and If statements. Let’s look at them one by one. See how case sensitivity can alter the equality .In the above example, age_group() prints a final message constructed with a common prefix and the string resulting from the conditional statement.In computer programming, we use the if statement to run a block of code only when a specific condition is met. Also the code you posted here is not indented correctly, albeit it could be caused by copy-pasting.Es wird normalerweise mit dem Schlüsselwort else verwendet, das einen Block ausführt, wenn die Bedingung in der Anweisung if nicht erfüllt ist.
if-Anweisung mit Zeichenketten in Python
elif condition not working properly for string matching in python.
String Equals Check in Python: Using 4 Different Methods
Couple things here.join, like this.Part 1: FUNDAMENTALS. Using strings in an if statement.The general Python syntax for a simple if statement is. About; Products For Teams; Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists share private .If the Python version you installed is 3.Python simpliest If statement with strings not working. The general Python syntax for a simple if statement is.A basic way to use an if statement is to see how some variable compare against a value. If statement using string function. It means that the control flow of the Python program will be decided based on the outcome of the condition.If strings are the same, it evaluates as True, otherwise False. For it to iterate over each element, write the if statement inside the for loop. You can use if .
However, You must be careful here, condition could also be 0 or 1, but any higher number or any literal will break the code. An if statement evaluates data (a condition) and makes a choice.
String Check with if Statement in Python
So Activity_2 shows the desired result. If the condition is true, then do the indented statements.
Python's if statement explained (with example programs) · Kodify
In Python, string comparison is basically a process through which we compare the strings character-by-character to check for equality.tutorialspoint.
Déclaration if avec des chaînes de caractères en Python
I'm trying to find a letter in a giving string.How to use f-strings in Python. If statementNested if statementIf/else statementNested if/else statementCascaded if statement. In the if statement, both variables are compared by using equal . We can compare strings using several ways like using ‘==’ operator, ‘!=’ operator, ‘is’ operator and __eq__ () function. In the case of if only, the specified process is executed if the condition evaluates to true, and nothing is done if it evaluates to false. Ein String ist eine . This will work because condition * False will return '', while condition * True will return original condition. OP wants to know if the variable is an empty string, but you would also enter the if not myString: block if myString were None, 0, False etc. Il est généralement utilisé avec le mot-clé else, qui exécute un bloc si la . That is, you index substrings that start in position 0, 1, 2, etc.Python string-based if-statement.The if statement allows you to execute a block of code if a certain condition is true. If you enter an integer value, still it will convert it into a string. In this case that is the statement printing “Thank you”. This isn't necessarily wrong (the code will still work) but there's no reason to convert input to a str, it already is a string.In Python führt die if-Anweisung einen Codeblock aus, wenn eine Bedingung erfüllt ist. Stack Overflow. We use the == operator to compare two strings.New to Python (2. We can also use conditional statements here like we did in previous sections.In Python, Whatever you enter as input, the input() function converts it into a string. print(10 greater than 5) .
Conditional Statements in Python
Lets have al look at a basic . Here is a working example, note that i convert to lowercase in order to accept both y\n and Y\N with a .join means that, join the strings returned by the generator expression with no filling character. The reason is that it doesn’t belong to the if block:For example: age = input( 'Enter your age:' ) if int(age) >= 18 : print( You're eligible to vote. If statement types. Compare Two Strings. String Concatenation Issue with the If Else Logic in Python . How to Compare Strings Using the <= Operator. Python: How can I use a String for a If-Statement? Hot Network Questions ID a .In any event, when you have finished with the if statement (whether it actually does anything or not), go on to the next statement that is not indented under the if.In this type of use case, the plus operator is your best option for quick string concatenation in Python.
If statement for strings in python?
find(test_word) - return index.To check a string against a set of strings, use in. Here's what I got: if i in steps == 'u': y -= pixels_per_move elif i in [steps] == 'r': x -= pixels_per_move steps is a randomly generated . It is possible, but it's not very Pythonic: something = a + b + c * condition.
Python list of strings and conditional statement
Is there a quick way for an if statement to accept a string regardless of whether it's lower-case, upper-case or both in python?