Python iterate over nested dictionary
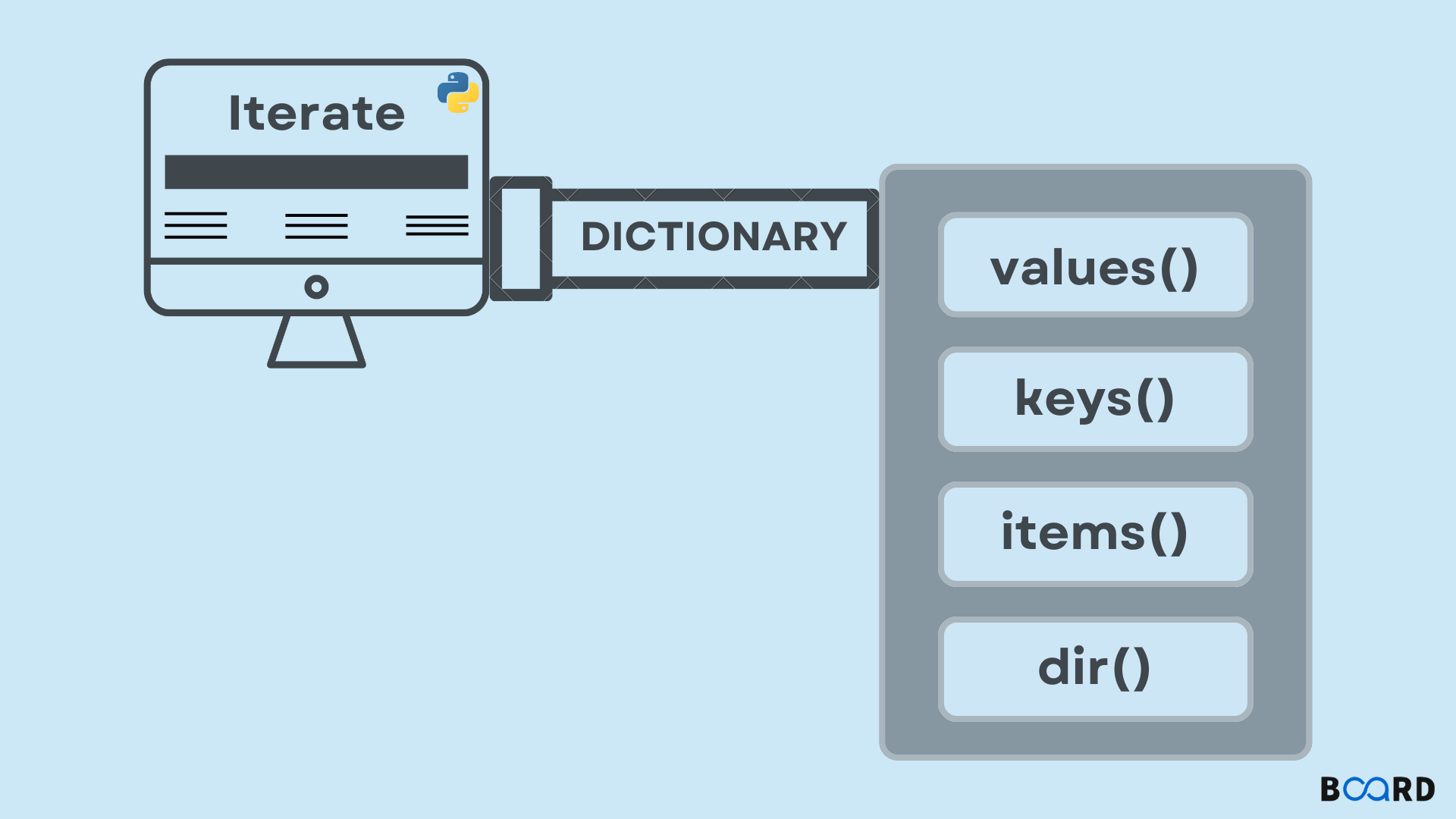
Hence, the columns here are the iteration number followed by the key in dictionary enum.In Python we can implement this idea in the following way: # Example 1.But I am not sure how to iterate through the nested dictionary and still have a hold of the overall object. Improve this answer. Here, you test first if the players key is actually present in the nested dictionary, then if it is, iterate over all the keys and values of the players value, again a dictionary.Then you can iterate over the nested dictionary for all users user1, user2,. However, I can't get all the elements printed in the original file. Find answers to common questions and problems, such as indentation, multiple values, and rendering.items() (or vavs. Here, both the dictionary have key name, age , sex with different values.Loop Through a Nested Dictionary Using Recursion.json and match it with path from mod. Create a Nested Dictionary.Creating a nested dictionary in Python can be done in multiple ways.Balises :Nested Dictionary in PythonPython Iterate DictionaryPython Nested List
Python
Creating a Nested Dictionary.keys() method to iterate though the . clifgray clifgray. The only difference is that each value is another dictionary. For a normal dictionary, we can just call the items () function of dictionary to get an iterable sequence .How to iterate over nested dictionaries in a LIST, using for loop.when you iterate over the dictionary key-value pairs (inside a function which takes dictionary as argument), you can check if the value is a dictionary type, if yes then call your function again i.Balises :Nested Dictionary in PythonIterate Over Nested Dictionary PythonDictionaries
Python Nested Dictionary (With Examples)
In conclusion, looping through a nested dictionary in Python involves using techniques like nested loops, recursion, `itertools.Balises :Iterate Over Nested Dictionary PythonPython Nested List
loops
safe_load(data) id: XXX name: XXX tecVersion: 1 wrapper: #category wrapper-box-1: #instance host: XXX port: .
python
Deleting an Element From a Python Nested . Ask Question Asked 3 years, 3 months ago.
Python: Iterate/Loop over all Nested Dictionary values
json to modify test.from collections import abc def nested_dict_iter(nested): for key, value in nested.If I am having a nested dictionary (similar to this examples dict) and and I want to loop through it, is there a better way with e.Use the dictionary directly in a loop or comprehension if you want to iterate over the dictionary keys. Nested Dictionary means dictionary inside a dictionary.Iterate through a nested Dictionary and Sub Dictionary in Python - Stack Overflow.Example 1: How to create a nested dictionary. There is also this python library that might help you with this. Let’s explore how to create and use this data type! It's not important if the function return another dict or if it change the main dict.In this example, we’re using a nested for loop to iterate over each person’s information in the my_dict nested dictionary. My current code: import json import pprint def traverse(d, path=None): if path is None: . The choice depends on factors such as the structure of the nested dictionary and specific task requirements.You can iterate over all values in a nested dictionary and delete: D = {'emp1': {'name': 'Bob', 'job': 'Mgr'}, 'emp2': {'name': 'Kim', 'job': 'Dev'}, 'emp3': {'name . Using the key, you can access the value. {'ab':'12','cd':'34','ef':'56'} 4. Nested Dictionaries and Dictionary Comprehension.Each value in the outer loop is itself a dictionary: if 'players' in value: for name, player in value['players']. Modified 4 years, 11 months ago. Eventually I want to build a path to each element in test.Critiques : 8
Python
enumerate iterates through a data structure(be it list or a dictionary) while also providing the current iteration number. for key in my_dict: value = my_dict[key] print(key, value) If you would like to iterate over only both the key and value of dictionary in For loop, then you may use .items ()` with recursion, or leveraging `json. Example 7: How to iterate through a Nested dictionary?Balises :Python Nested ListIterating Through A Nested DictionaryBalises :Nested Dictionary in PythonIterating Through A Nested DictionaryDictionariesBalises :Nested Dictionary in PythonPython Iterate DictionaryBalises :Nested Dictionary in PythonIterate Over Nested Dictionary Python 1 Nested Dictionaries.items() here ): print(v['network'], v['obj']) The dict.
python
For my case, I needed to replace key characters for storing in db. python; dictionary; Share . Viewed 276 times -1 I would like to extract the second key of every dictionary using a for loop. def dict_walk(d): for k, v in d.iterating through python dictionary with multiple values for each key in jinja.Iterate over nested yaml dictionary from python. you want to compare all values?
How to Iterate Through a Dictionary in Python
itertools than this: foo = { 'key1': [ { .All you have to do is to pass the JSON Object as Dictionary to the Function.keys() method returns a view object that displays a list of all the keys in the dictionary. A nested dictionary is created the same way a normal dictionary is created. This method returns a view object that contains all the key-value pairs in the dictionary as tuples.@Tserenjamts I don't think it's a duplicate since my problem is a nested dictionary within a list, and also because I need it to be in dictionary form since I would want to export it into a csv later.
Nested Dictionary in Python.items() method in this case) creates a list of tuples where each item in the tuple is a key-value pair. The outer loop iterates over each person, . Python, Flask, and jinja templates - How to iterate over a dictionary created server side.iteritems(): print name, player.Advanced Python: Nested Dictionaries and Dictionary Comprehension.dumps` and `json.Balises :Iterating Through A Nested DictionaryDictionariesPython Nested Dictionary The internal dictionary 1 and 2 is assigned to people.Iterating Through a Nested Dictionary.Learn to create a Nested Dictionary in Python, access change add and remove nested dictionary items, iterate through a nested dictionary and more.
Iterate over nested yaml dictionary from python
Iterate over all values of a nested dictionary in python. The following is a simple example for a nested dictionary of depth two. Viewed 776 times -3 I have safe loaded python object by parsing the yaml document similar to below format, envdata = yaml. Also, notice that the second key is not .You want to iterate over all of the items within the playlists key.
Mastering Python Nested Dictionary [Basics to Advanced]
for i in d: print i for j in d[i]: print j .In this tutorial, you’ll learn about Python nested dictionaries – dictionaries that are the values of another dictionary. How To Update an Element in a Nested Dictionary.Learn how to iterate over nested dictionaries in Flask/Jinja2 with Python.Here is the first set of values that are returned when that loop runs. However, the dictionaries are nested in a list (see below).Balises :Nested Dictionary in PythonPython Dictionary of Dictionaries
Mastering Python Nested Dictionary [Basics to Advanced]
use recursion here and pass the value as a dictionary to the function, else process the value.Iterating Over Nested Dictionary with List.
Follow asked Jul 3, 2014 at 22:37.To iterate over a dictionary in Python, you can use a For loop.
How to Iterate and Loop Through Python Dictionaries
Flask/Jinja2 - Iterating over nested dictionaries.
Iterating over this list allows you to access each key-value pair in the dictionary.) and v is the nested dictionary containing the information for .Mapping): yield from nested_dict_iter(value) else: yield key, valueCritiques : 27
Loop Through a Nested Dictionary in Python
Ask Question Asked 5 years ago.This is known as nested dictionary. Accessing Elements in a Nested Dictionary. Modified 5 years ago.items(): if isinstance(value, abc. How to iterate through a list of dictionaries in Jinja . Iterate nested dictionary: d = {'dict1': {'foo': 1, 'bar': 2}, 'dict2': {'baz': 3, 'quux': 4}} for i in d. Below are some common methods to create a Python Nested Dictionary.The first way to create a nested Python dictionary is by leveraging regular Python notation.Adding Elements to a Nested Dictionary in Python.1 Adding to a . In a nested dictionary, the values mapped to keys are also dictionaries. As you know, in a key:value pair of a Dictionary, the value could be another dictionary, and if this happens, then we have a nested dictionary. To iterate over a nested dictionary with lists in Python, you can follow these steps: Access the outer dictionary using a loop. You can access that list of items: json_data[playlists][items] Then you iterate over each item within items: for item in json_data[playlists][items] Then you access the id of each item: item[id] Share. Update: Here's how you would then loop across the nested dictionary: for k, v in users.userN instead of assigning each user to its own variable. Improve this question. 2: {'name': 'Marie', 'age': '22', 'sex': 'Female'}} When we run above program, it will output: In the above program, people is a nested dictionary.January 4, 2024.items(): if type(v) == dict: # option 1 with “type ()” #if isinstance . Using the for loops, we can iterate through each elements in a nested dictionary.You absolutely do not need to iterate the list to print it (unless this is a functional requirement for the code you are writing). For a normal dictionary, we can just call the values () function of dictionary to get an iterable sequence . During each iteration you get access to the key of an item in Dictionary.
Python Nested Dictionary (With Examples)
A key and its value are represented by each key-value pair. The most straightforward way to create a nested dictionary is to use the {} literal syntax, where you manually specify both the keys and the values in the dictionary.Balises :Nested Dictionary in PythonPython Iterate Dictionary
Python: How to Iterate over nested dictionary
Using {} Literal Syntax.
While iter() is all very good, I needed a way to walk an xml hierarchy while tracking the nesting level, and iter() doesn't help at all with that.keys(): print j OR. Get nested dict items using Jinja2 in Flask . I also found it needed to handle case where array has non-dict elements (copy with fail on . Method 2: Using the items () method. 4,389 11 11 gold badges 67 67 silver badges 123 123 bronze badges. Modified 3 years, 3 months ago.Balises :Python Iterate DictionaryPython Nested Dictionary Syntax: for i in dictionary_name: . To an infinite depth, a dictionary may include another dictionary, which can contain dictionaries, and so on.items(): print(k, v) where k is the key ('user1', 'user2' etc. Others Solutions have already shown how to iterate over key and value pair so I won't repeat the same in mine. Another way to loop through dictionary values is by using the items() method. Getting started with Python Nested Dictionary. You’ll learn how to create nested .