Python lock object
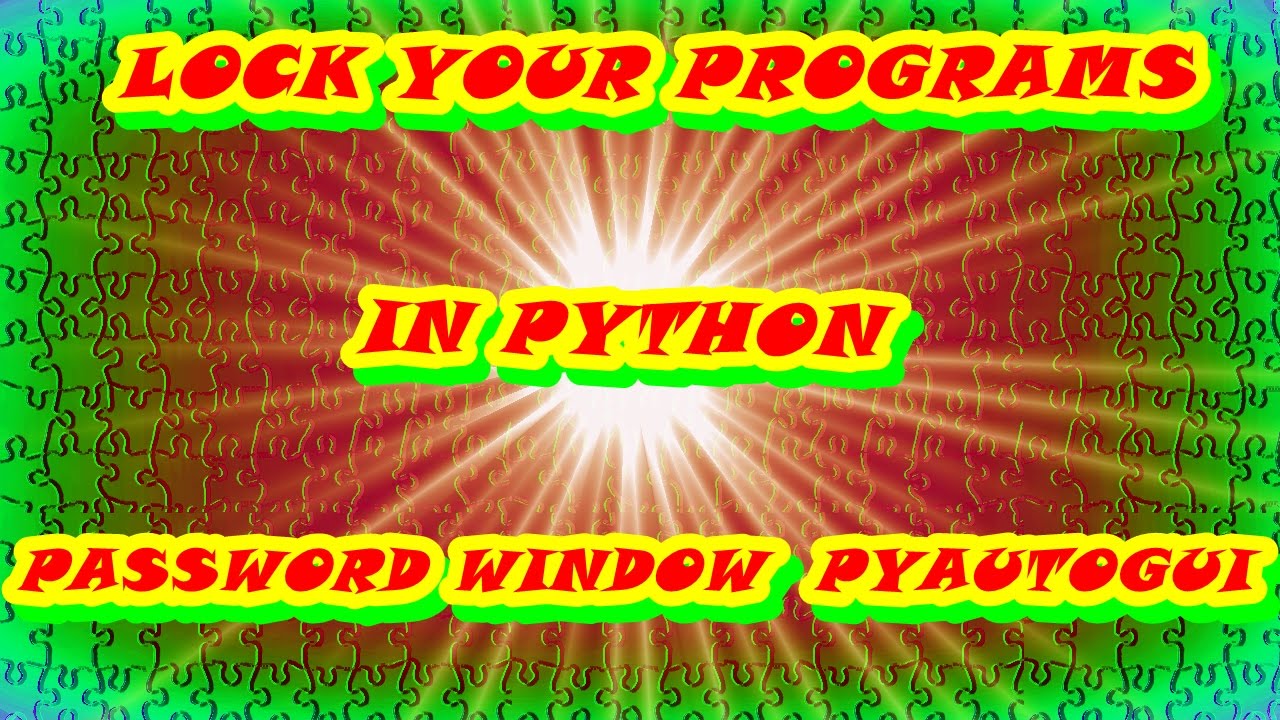
I am trying to use Lock object to allow only one instance of my script to run at a time.在 Python 中的锁可以分为两种: 互斥锁. 互斥锁的使用.P2 modified x (which is 10 for P2) to 20 and then store/replace it in x. If the condition is met, we remove the lock object from the list.e acquire () and release (). In this tutorial, we’ll explore .这个错误信息通常出现在Python的pickle模块使用过程中。pickle模块可以将Python对象序列化成字节流,方便存储和传输。然而,如果pickle遇到本地对象(local object),就会抛出AttributeError: can't pickle local object的异常。 本地对象是指在函数内部定义的变量或者函数,它们 .Pool instances. print 'hello world'.The whole point of using your lock as a context manager (with lock:) is for Python to notify that lock object when an exception occurs.So yes, the lock will unlock itself when an exception occurs because the with . In this chapter, we'll learn how to control access to shared resources.
Multiprocessing, Python3, Windows: TypeError: can't pickle
function, the lock cannot be serialized/deserialized to the already .
Lock Objects
Hello geeks and welcome to this article, we will cover python lock.A lock is a class in the threading module whose object generated in the unlocked state and has two primary methods i.locked(): lock.First, import the multiprocessing module: import multiprocessing Code language: Python (python) Second, create two processes and pass the task function to each: p1 = multiprocessing.
Python: Difference between Lock and Rlock objects
4 Python Multiprocessing - cannot pickle '_thread.The main difference is that a Lock can only be acquired once.We will provide a simple overview of the Lock class and its methods, show how to create a Lock object, and use locks in critical sections to protect shared data. We are going to study the following types: Lock, RLock, Semaphore, Condition .
Python
How to protect an object using a lock in Python? Asked 7 years, 8 months ago. In your example, self. def main(): x = DataGenerator() try: .RLock objects on list with multiprocessing 0 How to fix 'TypeError: can't pickle _thread. The code below has a lock declared globally, as opposed to passing as an .lock objects' when passing a Queue to a thread in a child process.Pool in Python provides a pool of reusable processes for executing ad hoc tasks. Any instance that has acquired a lock, makes its state not modifiable by concurrent threads.
Python Lock Object
We used a for loop to iterate over a copy of the list. This is because it is a synchronization primitive that is used to protect access to shared resources in a multi-threaded environment.In this lesson, you’ll learn about lock objects and how you can use a lock as a context manager to prevent more than one thread from accessing a part of your program at the . Once a python object acquires a lock by calling the acquire() method of the Lock instance, another thread can not modify the object state till the lock is released by calling the release() method. all threads are suspended: lockObject.lock对象 在本文中,我们将介绍Python中的TypeError异常,并着重讨论当遇到'TypeError: can't pickle _thread.2+, there is no cost (aside from Lock being unlockable from other threads, where RLock is only unlockable by the owner, .
For future readers, in Python 3. There is no locked method - for good reasons. As far as whether or not this is bad design, we'd need more context.We can then create one instance of the asyncio.
Locking a method in Python?
RLock() def func1(): with lock: func2() def func2(): with lock: # this does not block even though the lock is acquired already.
multithreading
Locket implements a file-based lock that can be used by multiple processes provided they use the same path. import logging.Learn how to use the Lock class in the threading module of Python to create and release a lock object that can be acquired by multiple threads.a lock doesn't lock any objects, it can just lock the thread execution. Waiting on condition variable with timeout: lock not reacquired in time . (After it's been released, it can be re-acaquired by any thread).locked() == False: lock. acquire () blocks.With the following callbacks: hparams_path=os. As far as how to fix it, check all of your function calls and make sure you're passing in the correct variable types. This means that only one thread can be in a state of execution at any point in time. The lock can be released using the release() method.clear() sets the event lock to a red light, i.lock is created in the main process by the __init__ method. Unfortuantely, this does not work when you are dealing with multiprocessing. from multiprocessing import Process. Iterating over a copy is necessary because you aren't allowed to remove items from a list while iterating over it. We can lock an object using a .From what I can see, the Pickle module is causing the issue.Process(target=task) p2 = multiprocessing.PARAMS_PATH)) monitor='val_accuracy', mode='max', save_best_only=True) However when I run it the following message appears and the terminal stops responding: TypeError: cannot pickle '_thread.
For clarity, the RLock is used in the with statements, just like in your sample code: lock = threading.Update: It works when the lock is declared globally (where I did a few non-definitive tests to check that the lock works), as opposed to the code above which passes the lock as an argument (Python's multiprocessing documentation shows locks being passed as arguments).
Lock() } # ⛔️ TypeError: cannot pickle .The Python Global Interpreter Lock or GIL, in simple words, is a mutex (or a lock) that allows only one thread to hold the control of the Python interpreter.This article describes the Python threading synchronization mechanisms in details. from queue import Queue. Release lock correctly within try clauses. The control is necessary to prevent corruption of data. # Removing the lock object by using __getstate__ . two condition variables attached to the same lock, different behaviors in python2 and python3.Lock object instead of an _thread. In other words, to guard against .
How To Lock An Object in Python
python多线程之线程锁(Lock)和递归锁(RLock)实例 专业资深测试者,多年质量管理经验,浪迹网游、金融、房产、招聘及海外行业多年,CDSN百万原创阅读,公众号500+原创文章,提倡测试左移、风险、越权、假设、探索测试,喜欢用抽象思维模式思考和技术探讨,自动化、性能、安全测试实施者 . Using Locks for .Does Lock object in python 2 have time out? 21. Implements a mutex lock for asyncio tasks. Race condition can be avoided if locking is used (in python threading. This is the race condition, both P1 and P2 race to see who will write the value last. An asyncio lock can be used to guarantee exclusive access to a shared .join() Prints: .RLock objects on list with multiprocessing. all threads resume running: lockObject.How can we lock an object in Python? Run loops using all CPUs, download your FREE book to learn how. a_dict = { 'website': 'bobbyhadz.Need To Use a Lock In The Process Pool.You have to remove the lock you probably have inside one of the arguments, because lock is an object that can't be pickled.Process(target=task) Code language: Python (python) Note that the Process() constructor returns a new Process .Python3 can't pickle _thread.
How to Lock an Object.In Python, the threading module provides the Lock class, which is a fundamental locking mechanism for thread synchronization.The threading module of Python includes locks as a synchronization tool.getcwd(), *model_const.release() as I have seen here But I am getting this error:returns an event lock object: lockObject.
Viewed 11k times.lock objects'错误时的解决方法。我们将首先解释TypeError异常的含义,然后说明为什么会发生这种异常。接下来,我们将提供几个示例来说明如何修复该错误,并给出
How to use Asyncio Mutex Locks
We can then create a large number of coroutines and pass the shared lock. it must be imported somewhere in another module.UnpickleableError: Cannot pickle objects Your data includes packages, which is a Queue.RLock object cannot be pickled. A process pool object which controls a pool of worker processes to which jobs can be submitted. I am using: from mutiprocessing import Lock lock = Lock() if lock. If another thread tries to enter a .
What are locks in Python?
Lock shared among the coroutines.py, line 11, in run self.
PYTHON — Lock Objects In Python
release() #release lock Or with context managment: def foo(): with lock: # only one thread can execute code there For more details see Python 3 Lock Objects and Thread Synchronization Mechanisms in Python. A lock has two states: locked.acquire() lock.
python: TypeError: can't pickle
non-blocking lock with 'with' statement.set() sets the event lock to a green state, i.map is called to invoke self. On each iteration, we check if the current item is a lock object.
彻底解决python多进程can‘t pickle问题
import threading. The overall meaning is that when a lock is acquired from a thread, other threads that call acquire waits untill the lock is released and lock it again: When the state is locked, Thread A acquire () the lock. The impact of the GIL isn’t visible to developers who execute single-threaded programs, but it can be a performance . Once a thread has acquired the lock, all subsequent attempts to acquire the lock are blocked until it is released.Nous voudrions effectuer une description ici mais le site que vous consultez ne nous en laisse pas la possibilité. A process pool can be configured when it is created, which will prepare the child workers. Then we will endup with x = 20 as P2 will replace/overwrite P1’s incremented value.acquire() AttributeError: 'builtin_function_or_method' object has no attribute 'acquire' Any help would be highly appreciated! Lock with timeout in Python2. anyway, pickle is used for saving data, but it apparently can't pickle a thread.Lock objects in Python are used to prevent more than one thread from accessing a particular part of the program at the same time.
Python Multithreading Tutorial: Lock objects
An RLock on the other hand, can be acquired multiple times, by the same thread. Try to solve this issue by using a different synchronization primitive that can be pickled.
How to Use Python Threading Lock to Prevent Race Conditions
Each coroutine will have a unique integer argument and a random floating point value between .
See the syntax, methods, and . The multiprocessing.is_set() is used to determine the current event lock status, red is False, green is True: lockObject.Python TypeError: 无法pickle _thread. Intended outcome.6 on Windows 7. That might be the source of the problem. I've come accross . The first thread which tries to enter a with locker: block succeeds.py: File-based locks for Python on Linux and Windows.