Repository pattern vs entity framework
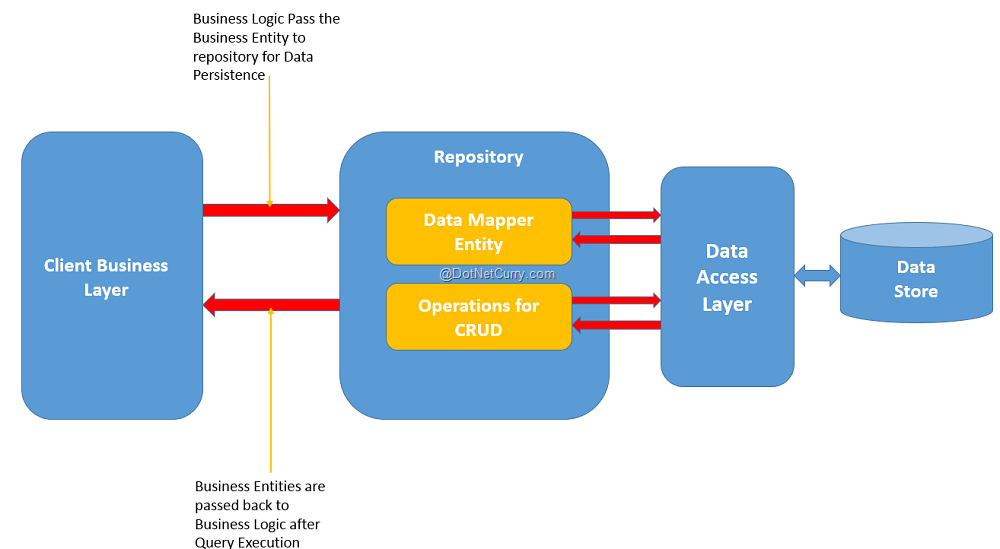
At this point, skip it alltogether and use directly EF.The first step of implementing the repository pattern is to extract out your EF Core LINQ queries to a .Repository Pattern.
entity framework
You can follow this article if you want to get a foundation generated for you. In this article, we will learn Entity Framework Repository Implementation for Relational databases like SQL in the .Update(entityA); repository.
As the name implies, it treats each part of the application (usually methods) as a unit.NET Core applications, exposing an asynchronous interface and leveraging . I find that we really do NOT need repositories with Entity Framework Core. The second one is called the code-first approach.Generic Repository, Unit Of Work et Entity Framework.SaveChanges(); } Should the idea be that we should dispose of the context as soon as possible otherwise the memory might leak or .; DbSet is already a repository provided by Entity Framework Core, so our repository will be just a thin layer to hide all .Tl;Dr – SummaryRepository pattern is used to abstract from particular database and object-relation-mapping technology (like EF) used. For example it makes the code look neater.
In current implementation the code of that methods look like below: Otherwise you’ll just break the abstraction with the entities instead (= the repository pattern isn’t very useful then).
Using the Repository Pattern with the Entity Framework
Design patterns that I often avoid: . client code) repository is same as DAL, except: (1) it's insert/update/delete methods is restricted to have the data container object as the parameter. Ignoring for now the arguments about whether the Repository Pattern should be used with EF, I'd like to ask . You can implement .Modified 4 years, 5 months ago. Repositories are classes that hide the logics required to .Implementing a Data Access Layer with Entity Framework 6. Similarly, when retrieving the list of entities, the repository will have to know how to retrieve each entity subtype and .Where(set => set.One of the important aspects of this strategy is the separation between the physical database, queries and other data access logic from the rest of the application. The six principles and patterns are: Separation of concerns – building the right architecture.It seems easiest, therefore, to examine the type of the entity when passed to your repository type and throw an exception if it is not a supported type since there's nothing else your repository will be able to do with it.I'm using the repository pattern with EF, and I think I'm pretty much sold on it but one question remains; If I want to eager load an entity, what is the best way to do this? For example, I have a repository mapped to an entity: public class EFFooRepository : IFooRepository { public IQueryable GetFoo() { .The Entity Framework DbContext class is based on the Unit of Work and Repository patterns and can be used directly from your code, such as from an .
The Repository Design Pattern acts as a middleman or . The Service Layer – separating data actions from presentation action.Repository pattern. This is the approach that we will for building our Repository. But in that case you can't use expression directly and you'd have to either have multiple . It requires DbContext in its constructor arguments (Which is injected with dependency injection) but just uses DbSet from it. Do note that the repository pattern is only useful if you have POCOs which are mapped using code first. The DbSet is the repository, and DbContext is the Unit of Work. In my opinion, using a generic repository is generalization too far . Implementing the Repository and Unit of Work Patterns.Add(myStudentEntity) repository. public static IQueryable GetAllRooms(this DbSet rooms) return rooms; public static Rooms GetSingleRoom(this DbSet rooms, int Id)However, it's just another anti pattern and is used frequently with Entity Framework to abstract calls to the data access layer. We already discussed basic details on getting started with EFCore in ASP.NET 6 application. I know most folks say I don't need to, but I'd like to have functions like. However, that was corrected in EF 6, so since that time there's been absolutely no benefit, even for testing purposes, in using the repository/unit of work .NET Core using GraphQL for . By using it, we are promoting a more loosely coupled approach to access our data from the database.
What is Generic Repository Pattern in Entity Framework
And do your tests! It is well documented in MSDN. EF's DbContext used to not implement an interface, so it made mocking it difficult for testing purposes.I want to create a repository style pattern for EF Core 6.Repository Pattern C# ultimate guide: Entity Framework Core, Clean Architecture, DTOs, Dependency Injection, Microservices | Medium. The Repository pattern allows you to manage the data from a data source (like a database) in a more object-oriented way. Better way - have one shared DbContext for one operation (for one call, for one unit of work ). Why implementing repository pattern when ORM already do that for us! For testing, just change the provider to InMemory. Software Development.NET Programming. In this article, I will discuss the Repository Design Pattern in C# with an Example from the context of Entity .Repository Design Pattern in C# with Examples.Repository/Unit Of Work pattern just duplicates what Entity Framework Core gives us with a DbContext — an abstraction from the database; If you consider a .EFCore Repository Implementation in ASP. using (var repository= new Repository(new MyContext)) {.With the Repository pattern, we create an abstraction layer between the data access and the business logic layer of an application.
Design Patterns
Dans cet article nous allons aborder plusieurs sujets qui permettront d’avoir une solution clé en main permettant de gérer nos données avec Entity Framework.Understanding the Repository Pattern. Instead of depending on an .
The Repository pattern is a popular way to achieve such an isolation. You can use repository classes with or without a unit of work class.A Repository pattern is a design pattern that mediates data from and to the Domain and Data Access Layers ( like Entity Framework Core / Dapper).Generic Repository Pattern in Entity Framework. Repositories – picking the right sort of database access pattern.About this code snippet: It extends from the specialized repository. Entity Framework provides us with the actual . It can be accessed using Entity Framework DbContext, or maybe the repository is an . var result = await _dbContext. A Repository mediates between the domain and data mapping layers, acting like an in-memory domain object collection.Entity framework. Having implemented it, we don’t have to call the database directly from our core logic.
Implementing & Testing Repository Pattern using Entity Framework
First, we will create a new basic-framework solution where we will store all the interfaces and base classes we need to implement the pattern repository.There's many reasons and a long history for why there's so much confusion here.This is a bad implementation of the Repository pattern i.Overall comparison.
Is the repository pattern useful with Entity Framework Core?
The Idea is, to define a generic interface IRepository and a class Repository that hides how the data is actually accessed.
e is not the Repo pattern. Client objects construct query specifications declaratively and submit them to Repository for satisfaction. Ignoring for now the arguments about whether the Repository Pattern should be used with EF, I'd like to ask if EF should return tracked entities.NET, Entity Framework Core, .In this tutorial, you review and customize the create, read, update, delete (CRUD) code that the MVC scaffolding automatically creates for you in controllers and views. Repository Pattern serves as an abstraction layer between the application’s business logic and the data access layer. The Repository Pattern, as well as the Unit of Work Pattern, allows to create an abstraction layer between the data access layer and the . Viewed 2k times.SingleOrDefaultAsync(); return .Since I wrote my first GraphQL post in 2019, much has changed with GraphQL in the .
Repository Design Pattern in C#
This article will walk you through the steps to create a basic GraphQL API on ASP.comEF Core Repository with Specification Pattern in .This article shows how I applied these principles and patterns in real-world applications that use EF. It provides a layer of abstraction by .
A Repository is defined as. This article discusses the basics of Repository pattern in the context of Entity Framework and ASP.Using the Repository pattern I have noticed that nobody actually uses the Using Pattern eg. In order for a .
Repository Pattern C# ultimate guide: Entity Framework Core
If you really want to use the Repository , do it properly and don't expose ANYTHING related to EF (entities, linq).There are many ways to implement the repository and unit of work patterns. (2) for read operation it may take simple specification like a DAL (for instance GetByPK) or advanced specification. Having a repository . So I can easily replace (for example) my . It's a common practice to implement the repository pattern in order to create an abstraction layer between your controller and the data access layer.var repositoryB = GetRepository(); repository.
Choosing a testing strategy
NET Core in our last article. As discussed in the Overview, a basic decision you need to make is whether your tests will involve your production database . Objects can be added to and removed from the Repository, as they can from a simple .Implementing the Repository Pattern Correctly with EF Corestackoverflow.Is there a chance your project will have different repositories it will talk to?
NOT using repository pattern, use the ORM as is (EF)
Add the Bsframework. Also, the code is cleaner and easier to maintain and reuse. In the external world (i. In this article we will be exploring Unit of work and Repository pattern with EF Core and .The Repository Pattern has been very popular in the last decade. Take, for example, the following code whose Get() method returns a tracked entity.