Ruby function return value
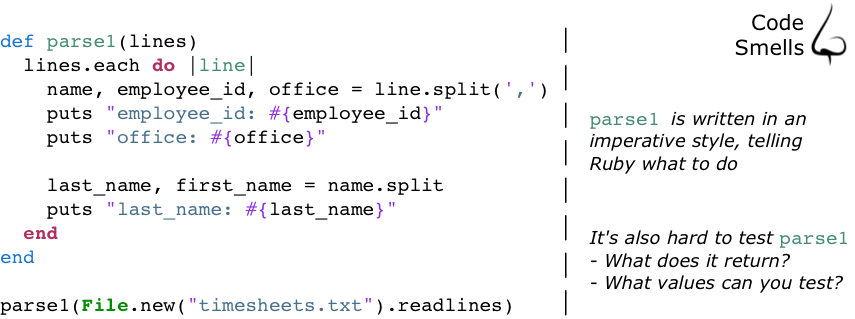
Note that for assignment methods the return value will always be .
Learning Ruby methods and how you should use them
For each this means that break exits the loop and next jumps to the next iteration of the loop (thus the names).
How do I return a variable from a Ruby method?
Yes, that’s .map {|v| #{v} = #{eval(v.
return true if total_value < item_value. You need to know the implementation and the behavior of the most used method in order to exactly know how your program will act. Functions can also return a value, which can be used later in your program. def two_plus_two return 2 + 2 1 + 1 # this expression is never evaluated end. Methods that end with an equals sign indicate an assignment method. hash # returns the hash from the method. For assignment methods . In ruby, a conditional whose test isn’t true returns nil. You call this using the name: my_function. If the argument is string, and happen to start with 0x, 0b, 0, interprets it as hex, binary, octal string respectively. This is because we aren’t returning x + y in . break inside a block returns from the function that yielded to the block.map {|name| #{name} is awesome!} When executing the file on the command line with ruby script.Why it’s important to know how ruby determines a return value. In this code, return a + b explicitly states that the sum of a and b should be the output of the add method.The default to_s prints the object’s class and an encoding of the object id. You don't even need that final . We can use it like this: add_three (5) # returns 8. # ruby # vscode. Here’s a normal Ruby function: def my_function.ruby_magic{|x| func(x) } my_x should be equal to first true value return of func(x) Suppose each X in arr is a regex pattern. You can return values with next value and break value. Any statement in ruby returns the value of the last evaluated expression. With methods, one can organize their code into subroutines that can be easily invoked from . new throws away the return value of initialize, and instead always returns the object that was just created.
Last line result in a method will be returned by default.
Return Values in Ruby: implicit, explicit, and puts, print, p
What you use is a rather nifty feature of ruby which allows to destructure arrays using the comma operator. Just check the length of the string: hash = ''.def method_name # Some code return value. So had we done this with our add method above: def add(x, y) puts x + y end z = add(2, 1) 3 => nil z == 3 => false z == nil => true. Functions in Ruby always return a value, even if no explicit return statement is provided.7You can also ignore the second return value by using this: a,=sumdiff(3,4)1How does Ruby return two values?24 févr. Sorted by: 169.
Ruby (Shoes)
This can be seen rather clearly in the implementation of Class#new. The object returned .17You can achieve this returning an array too, like def sumdiff(x, y) [x+y, x-y]end which seems functionally equivalent to def sumdiff(x, y). Conditionals return values. A method always returns only one value.
Functions are a set of instructions that return one value. Something like this: Something like this: # Let's say you're in a method in a class @interface = nil @combobox. Thus, in a function like so: def testing(target, method) (0.Note that you're not actually calling initialize, you're calling new, which then calls initialize. Return exits from the entire function.Calling
An introduction to method return value in ruby
Since the add_three method call . The backticks execute the command and return the output as a string. Imagine, I would have this ruby script test. Hence, the following works. sumdiff(3, 4) #=> [7, -1] a = sumdiff(3,4) #=> [7, -1] a.In ruby you don't have to return a value explicitly.
The return Keyword in Ruby
A method definition consists of the def keyword, a method name, the body of the method, return value and . yield is used to call the block associated with the method. I tried to put return true but only works when its true.
Why Can't You See Return Value of a Ruby Script in Command Line?
This keyword is named as .Ruby provides a keyword that allows the developer to explicitly stop the execution flow of a method and return a specific value.
Returning a string from a Ruby function
We pass back a value using the return statement, like so: defsay_hello_to(name)returnhello #\ {name}end puts say_hello_to .
Conditional assignment from a function with multiple return values in Ruby?
But usually, no not necessary.From within the python script I want to access to return value of the ruby function. hash << 'X' while hash.slice(start, length) -> an_array or nil array.We know from the above that the return value of sign(1) will be the value of the statement '' if number == 0. In Ruby functions, when a return value is not explicitly defined, a function will return the last statement it evaluates. However, in Ruby, you can also omit the return keyword, and the function will automatically .rb: class TestClass def self. Here is the ri documentation for the array index method:----- Array#[] array[index] -> obj or nil array[start, length] -> an_array or nil array[range] -> an_array or nil array. This works similar to the splat (asterisk) operator which can be used as a reverse operator. Every method always returns exactly one object. Ruby doesn't support multiple return values.rb the return value of the following function does . It's simply syntax sugar for returning a list.
You can return multiple values on a method using comma-separated values when you return the data. In this case, the return value of 'puts' method is nil.comHow do you return two values from a single method?stackoverflow. In Ruby, a method always return exactly one single thing (an object).execute{ fast_function } slow = promises[:fast]. My question is a follow up to this question: No return on command line when running Ruby script because the answer doesn't offer an explanation: Take the following file, script. item_value = item_value.value end As you can guess it will return the value of your fast function. puts add (5, 3) # Output: 8. puts also adds a keyboard enter/return (a “\n” newline character), so it . Functions that exist in an object are typically called methods. A return value and the work of the puts method — .
Return Values
Return Values | Ruby for Beginners.If you surround your command with backticks, then you don't need to (explicitly) call system () at all.In ruby-gnome2, for example, you would use a callback function/block with a Gtk::ComboBox that would change the state of your application.Returning Values from Functions.
That said, the following code will return the value of line.
puts and print are both methods that generally do the same thing, print strings to the console. I also tried with if else and it works but is this the best way? This means that if the second argument is not provided when calling the function, it will use the default value.How to return multiple values in ruby. Note, if you use return within a block, you actually will jump out from the function, probably . def sumdiff(x, y) return x+y, x-y. Because the OP did. It's a correction of the OPs code, not a recommendation of the Ruby-way to do it.Conversion of Puppet and Ruby data types. That is, I usually find that those re. How to Return Values (Basic Syntax) One key concept in Ruby is that ALL methods return a . And the most important, it .Ruby methods have a return value, a value they send back to the code that called them. @bar ||= foo[0] Share. If only a print . Otherwise, it will take the value passed as an argument.Meilleure réponse · 168Ruby has a limited form of destructuring bind: ary = [1, 2, 3, 4]a, b, c = aryp a # => 1p b # => 2p c # => 3a, b, *c = aryp c # => [3, 4]a. Without having to run every regex, I want to return the caputure group from the first match.
Get return value of ruby function in python
test_function(some_var) if case1 puts This may take some time # something is done here with some_var puts Finished else # just do something short with some_var end .Using return is unnecessary if it is the last line to be executed in the method, since Ruby automatically returns the last evaluated expression.
How To Use Return In Ruby: Effective Techniques And Examples
Here we are creating a method called multiple_values that return 4 .With Ruby, the lambda keyword is used to create a lambda function.
Method Return Values (How To)
A function is declared using the def .A method in Ruby is a set of expressions that returns a value.In the above function, the second parameter (b) has a default value of 0. total_value = some_value.The basic syntax of return in Ruby is straightforward: def method_name # Some code return value.Temps de Lecture Estimé: 5 min
ruby
However, on some rare occasions, the last expression is not easy to grasp, as in an interation or some obscure structure, so it might be good to put it.on_fullfill{ notify_client } return fast.slice(range) -> an_array or nil ----- .to_s)} } So, as you can see both from step 3 above and from the example, you cannot do this, because optional parameters are bound left-to-right, but you want to specify the middle argument.
methods
When function arguments are passed to a Ruby method, they’re converted to Ruby objects. 2015How do I return a variable from a Ruby method?1 mai 2013Afficher plus de résultatsHow to Return Multiple Values From a Java Method | . Here's a simple example: def add( a, b) return . Puppet converts data types between the Puppet language and Ruby as follows:The Ruby language makes it easy to create functions. Since you're already in the Hash you want, don't create another hash . Usually, it is not necessary to put it explicitly: rubyists knows that the last expression is the returned one.
How to Use Lambdas in Ruby
Function Syntax. String (arg) Converts the argument to the string using Kernel#to_s .def foo fast = Concurrent::Promise. Break exits from the innermost loop.signal_connect(changed) do |widget| @interface = .Yes, Ruby has very similar array-slicing syntax to Python.each {|elem| puts elem} return exits from the current method, and uses its argument as the return value, like so: def hello.Often they will may return an object to indicate a true value (or “truthy” value).
slice(index) -> obj or nil array.I created following method to return true or false. Further digging into the source code reveals that the hexadecimal number is, indeed, the memory address occupied by that object instance. We would get the rather unexpected result of z being nil instead of 3. Check the example below. It returns true if total_value < item_value but returns null if its not true. You call the resulting lambda function by using the call method. Improve this answer. Another extremely useful aspect about if and unless statements in Ruby is that they return values. As a special case, the top-level object that is the initial execution context of Ruby programs returns “main. But it will also call the on_fullfill function (Or a proc) if the slow function has finished. It can also be used to make a method return before the last expression is evaluated. In this structure, value is what the method will return when called. puts hello end. #=> [7, -1] a,b=sumdiff(3,4) #=> [7, .Float (arg) Converts the argument to the float value.An explicit return statement can also be used to return from function with a value, prior to the end of the function declaration. Note that this has implications on API . Here's a simple example: def add( a, b) return a + b.def sumdiff(x, y) return x+y, x-yend#=> nilsumdiff(3, 4)#=> [7, -1]a = sumdiff(3,4)#=> [7, -1]a#=> [7, -1]a,b=sumdiff(3,4)#=> [7, -1.The above method will return - not print out, but return - the value passed in incremented by 3.The fact that 'the return value of a ruby method is the return value of the last statement in the function body' causes the return keyword to be rarely used in most . So the return value of method 'cat' is nil.They each have return values of nil. It requires a block and can define zero or more parameters. Examples Your function can compute .The return keyword can be used to make it explicit that a method returns a value.Arguments are bound to parameters like this: Here's an example: p local_variables.
Return values
next inside a block returns from the block.