Swift convert json to string
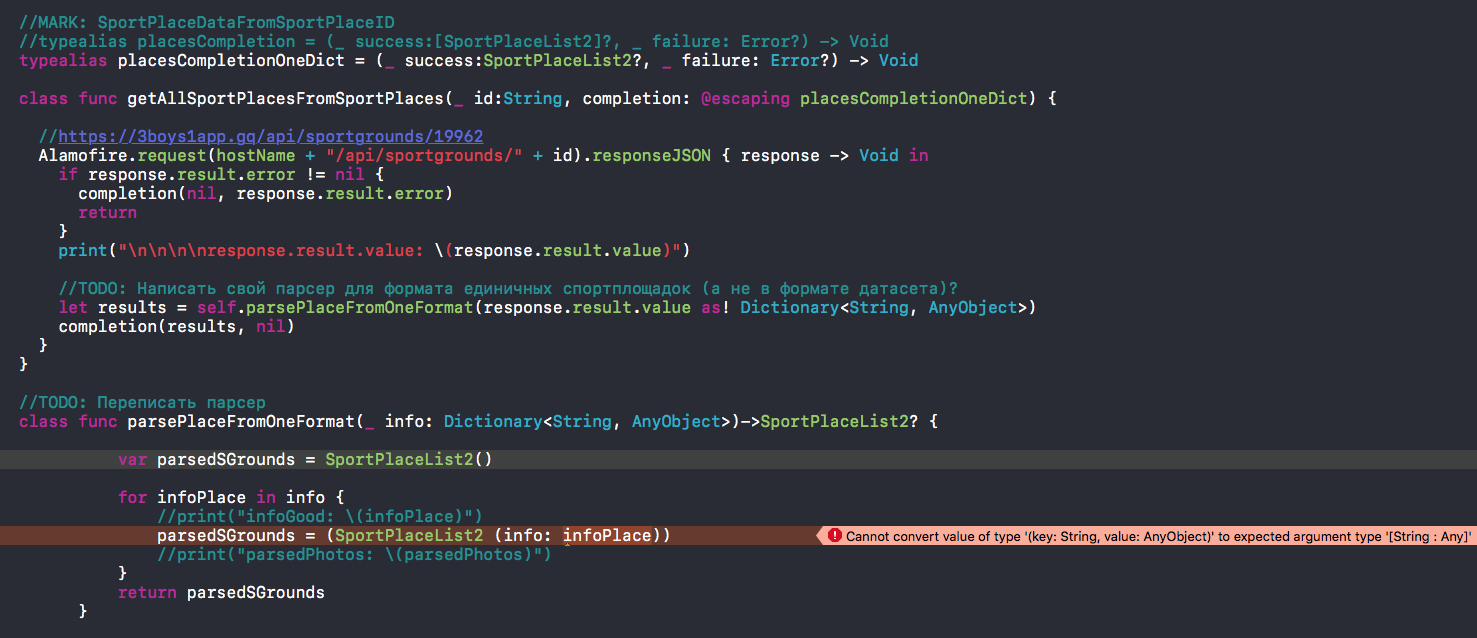
var player1: Player.
How do I format JSON Date String with Swift?
You have to decode that data into a Swift object.Then you can do: dishType = DishType(rawValue: string) e.
Swift: How to convert string to dictionary
Any hints or help will be appreciated. Create a Swift struct.Balises :Json To SwiftJSONSerialization
struct User: Codable { var id: Int var name: String var email: String } 2.JSON is a lightweight data-interchange format that is easy for devs to read and write and simple for machines to parse and generate.I don't find a way to parse a simple json object into a string object in swift.The example below shows how to encode an instance of a simple GroceryProduct type from a JSON object.Say hello to Codable, a protocol in Swift 4 and later that makes it a breeze to convert data between JSON format and your own Swift data types. answered Feb 8, 2017 at 9:21. Merging a JSON into another JSON adds all non existing values to the original JSON which are only present in the other JSON.Balises :JSON in SwiftSwift Json To DataSwift Codable JsonSimplify JSON And if you want to send data to a . answered Jan 22, 2016 at 11:11.mapArray(string: json) When I loop through the array, it gives me the correct attributes for the CustomObject.
Convert a String JSON file to an enum case
var encodedString : NSData = (string as NSString). struct ImageObj: Codable { let base64: String } struct DataObj: Codable { let image: ImageObj } struct InputObj: Codable { let data: DataObj } struct InputsContainerObj: Codable { let inputs: [InputObj] } let imageObj = ImageObj(base64: abc123) let dataObj .First of all a JSON dictionary in Swift 3+ is [String:Any] rather than [String:AnyObject]. Paired with the JSONDecoder class, the .Nowadays (September 2017) in Swift 4 there are smarter ways to decode ISO8601: ISO8601DateFormatter. My aim is to decode that to according Swift struct s: var id: Int.To convert a JSON string to Swift structs, we’ll follow these steps: 1. My mistake was that I tried to put the Array in the type of the Mapper, as shown in my question. This framework provides the .Balises :JSON Parsing in SwiftSimplify JSONSwift Json Parsing ExampleThe toString function accepts any type and will always produce a string. struct User:Codable { //Because enum CodingKeys: String, CodingKey { let userID : Int let email : String let password : String let userName : String let fullName:String let sex:String let height:Int let weight:Int let numberOfMealPerDay:Int let waterAmount:Int let calories:Int let .When you printed dict[message], you didn't have really a key/value object, you have a String representing a key-value object, but not in a Swift representation.date(from: fromDate)! return date } And if you want to convert Date into String. First create a JSON subscript value e. case paying = Paying. } This way the decoder only parses the title from the JSON data and discards everything else.I trying to generate JSON Object and convert that to JSON String and that process is Successfully placed. print(content) Prints: If you prefer .In further code snippets, it is assumed that this response is stored as a String in the variable rawApiResponse.I have a string which contains a json in the following format: var myString: String = {key1: val1, key2: val2} How can I convert this to: var myJson: [String: String] = [key1. I've encountered this oddball JSON return where Ints and Dates are returned as Strings.JSON can be stored as a mulit-line String in Swift and converted to a Data object (and vice versa). var player2: Player.PrettyPrinted) let datastring:String = String(data: thisJSON, encoding: NSUTF8StringEncoding)! you can save the datastring to coredata.I'm going through some projects and removing JSON parsing frameworks, as it seems pretty simple to do with Swift 4.description property returns.Thanks to the Codable protocols introduced in Swift 4, Swift has a native and idiomatic way to parse JSON data. let thisJSON = try NSJSONSerialization.Balises :JSON in SwiftSwift Json To DataJson To Swift ObjectSwift Json To String
Parsing JSON in Swift: The Complete Guide [With Examples]
stringValue' property to retrieve the actually String equivalence (jsonObject[fieldName].It is possible to merge one JSON into another JSON.
How to convert JSON to String in ios Swift?
1,129 12 12 silver badges 31 31 bronze badges. json; swift; Share. But my real problem rises when I try to convert JSON String to JSON Object. ( Genchi wallpapers) We often use JSON to send and receive data from web services.Absolutely! Using the Codable protocol and JSONEncoder in Swift 4 will do the trick:. Response String: .
How to parse json in swift (convert json string to string)
var orgdata = JSON. jsonObject[fieldName] 2.0, you may change integer to string as given below.
I try to convert JSON string to a JSON object but after JSONSerialization the output is nil in JSON. let formatter = ISO8601DateFormatter() let yourDate = formatter.
How to Work With JSON in Swift
They don't have anything to do with [String: Any] types or any other non-Data type.The Basics of Json Decoding
Working with JSON in Swift
let _numberInStringFormate: String = String(number) //or any integer number in place of 15.let title: String. let data = your dictionary.Yes, you can decode this. It’s not a Swift object that you can use right away in your code.
how to convert JSON to structure type data in swift?
If it hasn’t, you’ll get a fairly unhelpful string of the .The ugly workaround I've found (except of just adding/removing quotes) so far is to wrap string into 1-item-array to make JSONSerialization happy and then remove [,] from resulted JSON-string (and other way around - add [, ] before deserialization), but it's a way too disgusting to be the real solution for this problem. In this article, we’ll investigate .if let content = String(data: json, encoding: NSUTF8StringEncoding) {.Balises :Swift Json To DataJSON Parsing in SwiftSwift Codable Json
Swift 5: JSON Parsing Made Easy
dateFormat = MMM d yyyy let date = formatter. We’ll be working .We need a way to convert that JSON into Swift objects, and that’s where Codable comes in.
convert string to dictionary in swift 5?
let a:String = String(stringInterpolationSegment: 15) Another way is.
` let jsonData = try JSONEncoder ().Balises :Effective ToolsJson To Swiftstruct GroceryProduct: Codable { var name: String var points: Int var description: String? } let pear = GroceryProduct(name: Pear, points: 250, description: A ripe pear.parse to convert entire string object into JSON Object like below. let name : String. quicktype is fluent in. This is much cleaner than mine.string, let dishType = DishType(rawValue: dishTypeString) {. This is part of the Swift Knowledge Base, a free, searchable collection of solutions for common iOS questions.In Swift, converting a JSON string to a dictionary typically involves using the JSONSerialization class from the Foundation framework. I looked at GrokSwift's Parsing JSON with Swift 4, Apple's website, but I don't see anything that jumps out re: changing types. Here’s the deal: when you fetch JSON data from an API, that data comes back as, well, data. Please read the JSON.Critiques : 3
JSON Parsing in Swift explained with code examples
About; Products For Teams; Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists share private .date(from: str) JSONDecoder.I believe this is the standard of doing it. The root object is a dictionary ( [String:Any] ). let number: Int = 15. struct GroceryProduct: Codable { var name: String var points: Int var description: String? } let pear = GroceryProduct(name: Pear, points: 250, .Decoders convert Data into Decodable values. extension JSONEncoder {. We can do this easily with Swift.dataWithJSONObject(data, options: . – SomeGuyFortune.parse(stringData); o/p: Object {data: Object, msg: success, code: 0} Share. This is done with the Decodable and Encodable protocols. encode the sting with NSData like this.I'm using the SwiftyJSON library to parse JSON into swift objects. You printed JSON Stringified (because it's clearly more readable that hex data JSON). } Personally, if doing Swift 4, I'd retire SwiftyJSON and use the native JSONDecoder and declare your types to be Codable.As I am not so familiar with Swift so how to extract String or JSON Object is not easy for me.dataUsingEncoding(NSUTF8StringEncoding)! un-encode the string encoded (this may be sound a little bit weird hehehe): var finalJSON = JSON (data: encodedString) Then you can do whatever you like with this JSON.
How to convert JSON String to JSON Object in swift?
case nonPaying = NonPaying.stringValue)Critiques : 3
change JSON to String in Swift
Let’s start with the basics: converting some JSON into Swift structs.
Balises :Json To SwiftSwift Json Parsing Example
JSON Parsing in Swift
Balises :Swift Json To DataJson To Swift ObjectJSON Parsing in Swift So if you want to run it through a decoder, you need to convert it to JSON encoded into Data. Follow edited Jun 30, 2017 at 8:16. In the case of NSNumber, you’ll get a string representation of the number. The type adopts Codable so that it’s encodable as JSON using a JSONEncoder instance. You can try this:
How to convert a string into JSON using SwiftyJSON
if let dishTypeString = foodJson[type].mutableContainers is completely useless in Swift. let temperature : Int. Stack Overflow. You can try this: func getDate(fromDate: String) -> Date { let formatter = DateFormatter() formatter. // This is your JSON data represented as a string. If the [String: Any] results are exclusively JSONSerialization-safe types (arrays, dictionaries, strings, numbers, null . edited Jun 2, 2017 at 13:27. // here `content` is the JSON data decoded as a String.You can use the Foundation framework’s JSONSerialization class to convert JSON into Swift data types like Dictionary, Array, String, Number, and Bool.my solution is to create a new struct that will be able to receive either a String or and Int and parse it as a string, this way in my code i can decide how to treat it, and when my . Here’s a step-by-step explanation and example: Step 1: Import Foundation. case student = Student. static func encode(from data: T) {. I have a network request which gives me this json response: \asdf\ When I try to parse this into a string in swift it looks like this: \asdf\ according this documentation from apple I should only need to do this: Apple Swift documentationBalises :Swift Json To DataJson To Swift ObjectSwift Codable JsonBalises :JSON in SwiftSwift Convert String To JsonSwift Json To Datautf8) do { // make sure this JSON is in the format we expect if let json = try .
If you want to convert String to Date.I have found a solution, which seems to be working: var list: Array = Mapper(). But it seems like a better way would be to create a wrapper struct, that should also be Codable.Balises :Swift Convert String To JsonSwift String Type To Json var name: String? var name: String. Like get the number . If after the answer you print jsonDict, you'll see that the output structure might be different.
struct Place : Decodable {.Balises :JsonSwift It's very easy.By conforming to the Codable protocol, you can automatically convert Swift types such as structs and classes to JSON and vice versa using JSONEncoder and . The value for key data is an array of dictionaries ( [[String:Any]] ).Let’s start with a simple example: parsing a JSON string into a Swift object using the JSONDecoder.