Swift internal keyword
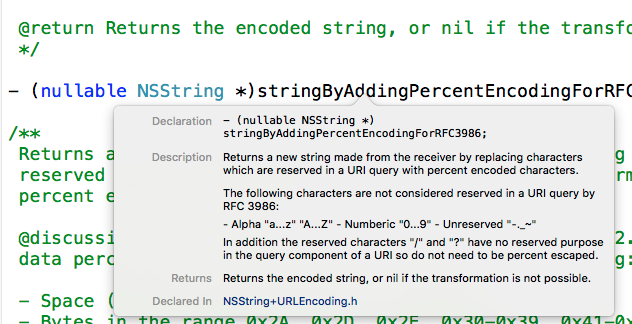
A protocol can require any conforming type to provide an instance property or type property with a particular name .Structures and classes are general-purpose, flexible constructs that become the building blocks of your program’s code.7, Apple makes another great improvement on both of these keywords.
We can now use both of these keywords in the function’s parameter position! Let’s say we have a struct: struct Person {var age: Int = 16}Now, this is a pretty simple struct.
Functions
A reserved word is a word that cannot be used as an identifier, this is a syntactic definition, as mentioned before in .
Access control in Swift like a boss
Optional Chaining
swift2
It provides a way to organize code and make it more readable and maintainable.Initialization is the process of preparing an instance of a class, structure, or enumeration for use.
Functions
Not having a function inlined, however, can be necessary in some code. Accessing Superclass Methods, Properties, and Subscripts in page link.
Keywords in expression and type. Aug 18, 2016 at 21:28.Because range Of Four Items is declared as a constant (with the let keyword), it isn’t possible to change its first Value property, even though first Value is a variable property. If explicit getters and setters using get and set are not defined, willSet can be used to take action before a new value is set for a property:.
How to declare a 'protected' variable in swift
All the Reserved Keywords in Swift
Use Case: internal is the default access level in Swift.
[Pitch] Access-level on import statements
In Swift, enumeration is defined using the “enum” keyword, followed by a set of cases that represent the possible values for the enumeration. Part of Mobile Development Collective. In your code: var vc: NSString? The internal keyword is an access modifier for types and type members. However the keyword internal is used just for developers understanding. Model custom types that define a list of possible values.Access control: A set of keywords that control how properties may be accessed by other code.
When I use internal access control level explicitly in Swift 4
An enumeration defines a common type for a group of related values and enables you to work with those values in a type-safe way within your code. Viewed 39k times. Public access enables entities to be used within any source file from their defining module, and also in a . Viewed 35k times. Is there a way to declare an inline function in Swift? Asked 9 years, 3 months ago.Keyword in statement.Access control restricts access to parts of your code from code in other source files and modules. An open class can be subclassed in the module it is defined in and in modules that import the module in which the class is defined. For example, you could create a static function in a math class that calculates the square of a number. Let me explain what I mean by that.
Swift Inheritance (With Examples)
An attribute provides additional information about the declaration or type.
Extensions
You indicate type methods by writing the static keyword before the method’s func keyword. internal allows use .1 whereas the any keyword was introduced in Swift 5. struct Book { . It allows code elements to be accessed within the same . In this tutorial, we will learn about the reserved keywords in Swift programming.if let always create a new variable, with a scope of its own (from if to {}).It makes no sense to prefix a type definition of a value type with the final . Modified 12 months ago. Also I don't think protected is for the sole purpose of protection, but for the .Declare extensions with the extension keyword: extension SomeType {// new functionality to add to SomeType goes here} An extension can extend an existing type to make it .5k 4 4 gold badges 41 41 silver badges 37 37 bronze badges. This check ensures that your overriding definition is correct.From what I understand, this is not possible, at least at compile time.Swift `in` keyword meaning? Asked 8 years, 11 months ago. Property Requirements in page link. This page covers internal access. Keyword used in the .Prerequisites to understand: Swift 5 syntax; You may have seen someone else’s Swift code include the keyword lazy, but what does that mean?The lazy keyword is used to solve problems with processing speed. public class BaseClass { // Only . open is a new access level in Swift 3, introduced with the implementation of.
Properties
You can use the internal keyword like this: class . This means that SomeInternalClass and someInternalConstant can be written without an explicit access-level modifier, and will still have an access level of internal.Hi everyone, Here’s a proposal for more control over dependencies and imports in Swift.
The same applies to internal keyword as well yet they allow internal to be there as default modifier for classes in a package.You would have to use internal for that as Swift doesn't offer a protected keyword (unlike many other programming languages). Sorted by: 678. This behavior is due to structures being value types. They cannot be used for variable names constants or any other identifiers.
Manquant :
internal keyword It’s the default access control level, meaning the Swift compiler . The GridController (the UI) should be accessible to the rest of the application, but the model class is wholly internal and should never be accessed by the rest of the application. For example, // define a superclass class Animal { // properties and methods . keywords that begin with the number sign. When you provide a . This process involves setting an initial value for each stored property on that instance and performing any other setup or initialization that’s required before the new instance is ready for use. Unlike other programming languages, Swift doesn’t require . You define properties and methods to add functionality to your structures and classes using the same syntax you use to define constants, variables, and functions. Classes can use the class keyword instead, to allow subclasses to override the superclass’s implementation of that method.Keywords used in declarations: associatedtype, class, deinit, enum, extension, fileprivate, func, import, init, inout, internal, let, open, operator, private, precedencegroup, protocol, .If you want a user of that framework to be able to subclass a class or override a method you must make it open.Critiques : 4Lexical Structure
You indicate the function’s return type with the return arrow-> (a hyphen followed by a right angle bracket), which is followed by the name of the type to return. This process involves setting an initial value for each stored property on that .The override keyword also prompts the Swift compiler to check that your overriding class’s superclass (or one of its parents) has a declaration that matches the one you provided for the override. internal declares an internal access level that allows entities to be accessed within any source file from the module that defines it, but not outside of it.Swift's final keyword is often overlooked or dismissed as not necessary. There are four types of keywords in Swift based on the location of their usage in a swift program. In Objective-C, you can define type-level methods only for Objective-C classes. Because the generated header is part of the framework’s public interface, only declarations marked with the public or open modifier appear in the generated header for a framework target.
open means the property can be accessed and overridden from anywhere, public .- Stack Overflow. Additionally, the newer package access-level . In Swift, we use the colon : to inherit a class from another class. Both vc are different variables and occupy different memory locations. If you are familiar with C, you will know that C enumerations assign related names to a set of integer values.There are two kinds of attributes in Swift — those that apply to declarations and those that apply to types.Enumeration is a powerful feature in Swift that allows developers to define a group of related values in a single type. While in if statement, it creates new constant variable, which can't . For example, in one file, I'll have a GridController and a GridControllerModel.In Swift, the keyword static is used to define a static function or property.In my Swift code, I often use the private modifier to limit the visibility of helper classes.The generic version of the function uses a placeholder type name (called T, in this case) instead of an actual type name (such as Int, String, or Double). The internal keyword is also part of the protected internal access modifier. If we discuss the final keyword, then we also need to talk about inheritance.
Access control
In this article. With this feature you can mark imports as public (the current regular import), internal for implementation details of the module, and private or fileprivate for implementation details to the source file.
When use default access level internal, no need to add internal explicitly. Swift provides three different access levels for entities within your code.You introduce enumerations with the enum keyword and place their entire definition within a pair of braces: enum SomeEnumeration {// enumeration definition goes here} Here’s an .All of this information is rolled up into the function’s definition, which is prefixed with the func keyword. These access levels are relative to the source file in which an entity is defined, and also relative to the module that source file belongs to. This feature enables you to hide the implementation details of your code, and to specify a preferred interface through which that code can be accessed and used.Access control lets you specify what data inside structs and classes should be exposed to the outside world, and you get to choose four modifiers: Public: this means everyone can . class Notes { func storePreviousSaveValue(_ canSave: Bool) {} var canSave = false { willSet { // Inside of willSet, the property // canSave will be the old value, // before a new one is set .It reuses the existing concept of the internal keyword instead of introducing a separate concept for this feature, improving teachability. The definition describes what the function does, what it expects to receive, and what it returns when it’s . It allows code elements to be accessed within the same module but not outside of it.Welcome to Swift Tutorial.
Access Control
The internal modifier is the only one of the two that actually exists in the Swift language, but the two names .
Open — This is where you can access all data members and member functions within the same module (target) and outside of it.internal properties, methods, and entities can be accessed by anything in the same module. Internal types or members are accessible only within files in the same assembly, as in this example:.var vc: NSString? But I wouldn't call that no difference: internal is a Swift keyword and moduleprivate isn't. SE-0117 Allow distinguishing between public access and . You indicate the function’s return type with the return arrow-> (a hyphen .
Swift keywords are the words reserved for a purpose. You implement this initialization process by . Methods and properties that are marked with the internal modifier and declared within a . In Swift, you can define type-level methods . Because protocols are types, begin their names with a capital letter (such as Fully Named and Random Number Generator) to match the names of other types in Swift (such as Int, String, and Double). Keywords in the specific context.