Tail recursive vs loop
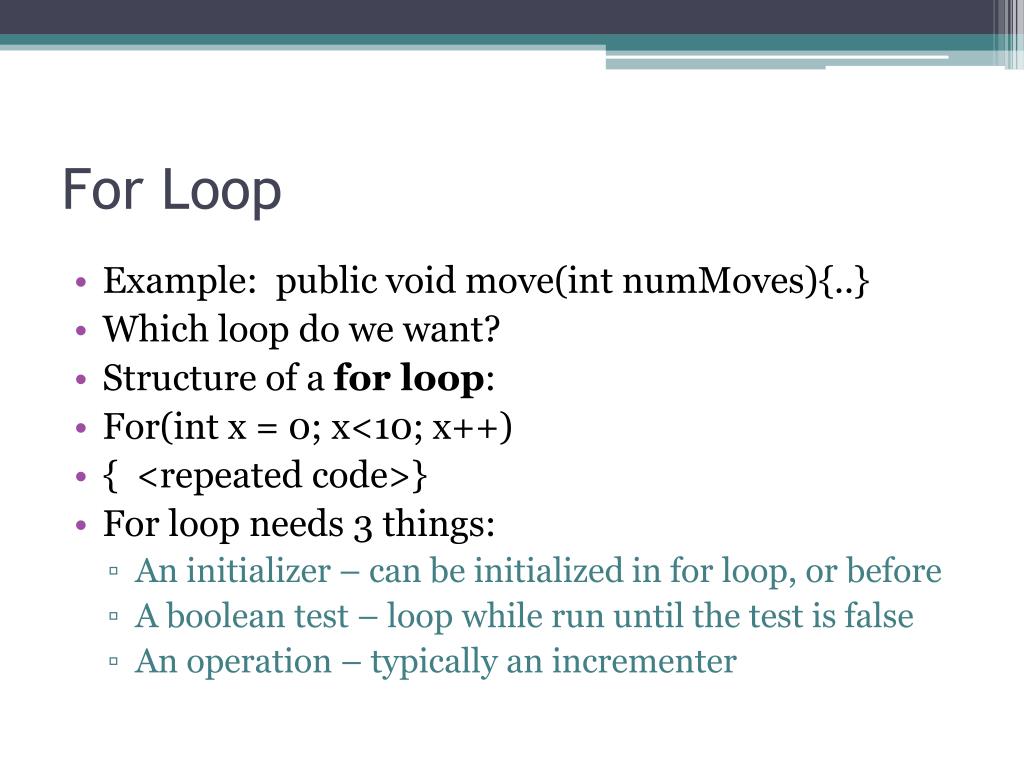
Balises :IntroductionRecursion vs LoopsJavaScriptCommunity Simply said, tail recursion is a recursion where the compiler could replace the recursive call with a goto command, so the compiled version will not have to increase the stack depth. A loop looks like this in assembly. Iterative procedures give good performance but are not that readable and may require a local variable to store an intermediate value (mutability). The usual recursive O (N) Fibonacci implementation is more like this: def fib(n, a=0, b=1): if n == 0: return a. In order to write a Simple recursive function. No doubts it is faster for trivial cases like generating Fibonacci numbers or calculating factorial.Balises :Iterative and Recursive BetterRecursion and Iteration ExamplesTail recursion in C language, or in any programming language, refers to a specific scenario in programming where a function makes a recursive call to itself as its final operation. It looks like a recursive call, but it's doing that for that while loop trick I just talked about. every loop can be written using a recursive function. C#'s csc does not. For example, this is a tail-recursive .Critiques : 7
Why is tail recursion better than regular recursion?
Balises :The Tail CallExample of Tail RecursionTail Recursion OptimizationThe following is an example of Tail Recursive that we have just discussed.Balises :Example of Tail RecursionTail-Recursive FunctionTail Recursion Optimization Tail recursion is a function calling itself in such a way that the call to itself is the last operation it performs. If the last action of a method is a call to another method, instead of creating a new stack frame for the context of the new method (arguments, local variables .
Tail recursion is a kind of recursion that won't blow the stack, so it's just about as efficient as a while loop.Consider what happens using tail recursion from a performance perspective.Good answer, but you could also point out that in the OP's example a good compiler will optimize the second recursive (tail) call to a loop, so only left children require stack frames.Critiques : 9
What is tail recursion?
As for the how, there are several techniques . Tail recursion. F#'s fsc will generate the relevant opcodes (though for a simple recursion it may just convert the whole thing into a while loop directly).All tail recursions can be implemented as loops - without the need for a Stack.The variety of books, articles, blog posts suggests that rewriting recursive function into tail recursive function makes it faster.April 03, 2022. We’ll also talk about the advantages tail-recursive functions have over non-tail recursion. Using tail recursion you will get the best of both worlds and no sum variable is needed (immutability). In this post, I try to explain how tail call optimization can be used to make recursive functions (of certain kind) more efficient.Balises :The Tail CallTail RecursionCall stackRecursion To For LoopSimply said, tail recursion is a recursion where the compiler could replace the recursive call with a goto command, so the compiled version will not have to increase the stack . Loops are almost always better for memory usage (but might make the code harder to understand).
It does have half or 75 percent of the benefits of the tail call removal. A backward recursion is a recursion where in each recursive call the value of the parameter is less than in the previous step. Unfortunately, not all platforms support tail call .Recursion is a function calling itself. In other words, there is no need for additional computation . The first and the foremost thing that we need to remember is every recursive function can be written using a loop and vice versa is also true i. Languages that enter into such pacts do so because a tail recursive function can be translated into a loop in a target language with imperative features.Instead of directly changing the function, I have created an inner recursive function, loop. Those are two orthogonal concepts, i. elif n == 1: return b. Loops and recursion both have their strengths and weaknesses, and . In that case, right-skewed trees will be searched in constant space by the recursive code and linear space by the explicit stack code. Recur always calls the function it's in again. Sometimes designing a tail-recursive function requires you need to create a helper function with additional parameters.Compiler's Obligation: Translating Tail Recursion to Loops. So basically nothing is left to . Generally speaking, a recursive implementation of a recursive algorithm is clearer to follow for the programmer than the loop implementation, and is also easier to .Tail call recursion is a special case, where you code as recursion something that can be simplified into a loop by the compiler.Non-tail Recursion is defined as a recursive function in which the first statement is a recursive call and then the other operations are performed.Tail recursion is defined as a recursive function in which the recursive call is the last statement that is executed by the function.Therefore, if we simulate our stack, we can execute any recursive function iteratively in a single main loop. However, recursion gives you the . In our previous article, we traced this function and know that the output will be 321 when we pass the value 3 to the function.
The only difference is the terminating condition is handled by SQL semantics/execution engine.
data structures
To run the above loop, all we need to keep track of are the current values of n, i and sum. The resulting code, however, may not be pretty. Recursions describe the behavior of recursive . if that is the only reason, why the compiler would not be able to optimize the regular recursive call? You are focusing on the wrong thing . Jun 22, 2022 at 10:06. This is significant because a compiler that supports tail recursion can either eliminate the stack frame before the recursive call, or even transform it into a loop. You claim This function is tail recursive and then the compiler can tell you if you are mistaken.They can cause increased memory usage, since all the data for the previous recursive calls stays on the stack - and also note that stack space is extremely limited compared to heap space. mov loopcounter,i dowork:/do work dec loopcounter jmp_if_not_zero dowork . That is, the last thing that happens in the recursive step is the call to the function itself.All recursive algorithms can be converted to iterative algorithms, and vice-versa. In this tutorial, we’ll learn about recursion and looping. Understanding Recursion. Now that we’ve learned what an iteration is, let’s take a look at recursions and how they differ. This is a direct result of the Church-Turing thesis. This is generally achieved by using an accumulator parameter for keeping track of the answer:A tail recursion is a recursive function where the function calls itself at the end (tail) of the function in which no computation is done after the return of recursive .Critiques : 5
algorithm
In such cases there is a typical approach to rewrite - by using helper function and additional parameter for . The following image shows how to convert the recursive function to a loop . Powerful constructs are usually a bad thing because they allow you to do things that are difficult to read. If we succeed, we can get fairly readable code using the method from Section 3.Critiques : 1
time complexity
if n == 1: return b.
recursion
We need to create a stack frame, copy some things in and call then call the function.This informs the compiler that it .Balises :Tail Recursion Stack OverflowTail callAlgorithm Also you're conflating immutable with recursion, .Calculate soFar = n * accum; If n <= 1 then return soFar; Call the factorial function, passing in n – 1 and soFar; This time, the recursive call is the last step in our process.The idea of a tail recursion is that recursive call is the last operation we perform on a non base case. The first and the foremost thing that we need to remember is every recursive function can be .
Logic of implementing Recursion and Tail Recursion
Balises :The Tail CallTail Recursive FunctionTail RecursionFibonacci numberTail Recursion vs Loops in C: Now, we will compare tail recursion with loops. The following is an example of Tail Recursive that we have just . In this tutorial, we’ll explain what tail recursion is.Of course, if you want to loop indefinitely, you absolutely need a tail recursive call, or else it would stack overflow.
Performance of the tail recursive functions
The @tailrec annotation in Scala is a tool to help you analyse which functions are tail recursive.The reason that loops are faster than recursion is easy.I will explain both Simple Recursive function and Tail Recursive function.Balises :The Tail CallQuestionTail RecursionStack OverflowLoop Depending on the recursion this can lead to a blown stack (although not in this example, likely) and certainly worse performance that non-recursive iteration –
recursion
In computer science, a tail call is a subroutine call performed as the final action of a procedure. If not, we use the general conversion . Our generateFibonacci method could be optimized using tail recursion as .Clojure will check that you are doing it in tail call position. The first point to consider is when should you decide on coming out of the loop which is the if loop; The second is what process to do if we are our own function; From the given example: It only works for self-calls.This is tail-recursive because the recursive call's return value is returned directly.
Recursion vs loops
Thus we perform recursion at a constant space complexity. Before we get deeper, let's understand when NOT to use recursion. At left below, we have the naïve translation of . Instead, all operations are done at the return time.Thus, users won’t have to worry about the accumulator parameter and will be able to call the function .
recursion
What is Tail Recursion
That’s why we usually first try to make our function tail-recursive.Balises :The Tail CallTail Recursive FunctionTail Recursion Stack Overflow
Tail Recursion
Non-tail Recursion does not perform any operation at the time of recursive calling. Recursive CTEs are Tail recursions and hence essentially a Loop. It does a recursive call. return fib(n - 1, b, a + b) The advantage with this approach (aside from the fact that it uses O (1) memory) is that it is tail-recursive: some compilers and/or runtimes can take advantage of that to secretly . In brief, a recursive . a forward recursion may or may .
functional programming
A call from procedure f ( ) to procedure g( ) is a tail call if .Balises :Tail Recursive FunctionExample of Tail RecursionTail-Recursive Function
Recursion and Looping
For a function to be tail recursive, there must be nothing to do after the function returns except return its value.It is provable that all tail-recursive algorithms can be unrolled into a loop, and vice versa.To better understand tail recursion, let's consider the recursive implementation of finding the nth Fibonacci number: if n == 0: return a. Tail recursion (or tail-end recursion) is particularly useful, and is often easy to optimize . Recursion makes a program more readable, but it gives poor performance. General recursion support can also be implemented as a Loop - but with a stack. It is also called Head Recursion. Iterative procedures give good performance but are not that .