Trees in python
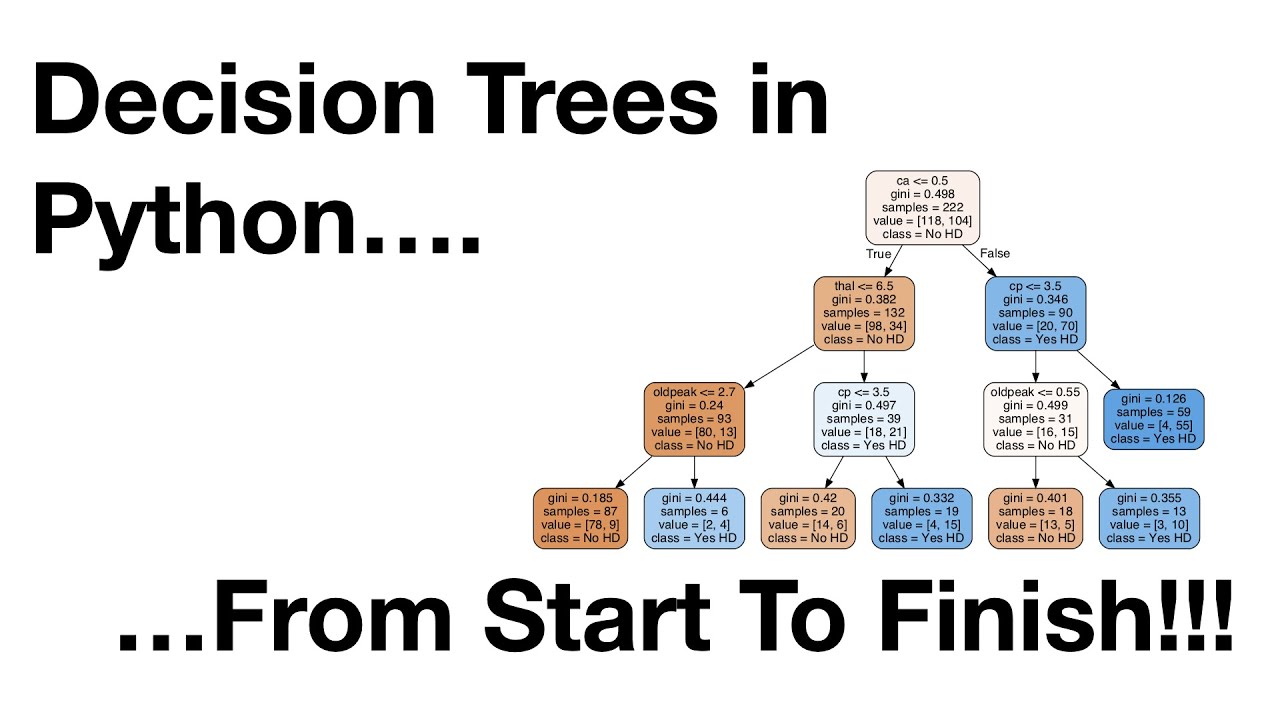
A state diagram for a tree looks like this:Learn how to create and manage trees in Python using a class named 'Tree'. Then passed the positive and negative integer and float point values to them using the Python .
Binary Tree implementation in Python
In a binary tree, a parent node can have at most two children nodes. A subtree branching off from the left child of a given node, is referred as its left subtree , and similarly, a right subtree is the subtree that branches off from the right child .netUn Arbre Binaire de Recherche en Python - MarcAreamarcarea. In computer science, a tree is a non-linear data structure consisting of nodes .A tree as a data structure can quickly become a complex mathematical subject ( check the wiki 👀 ), we are surrounded by real and virtual things ( data really) that .Learn about the basics of tree data structure, its terminologies, types, and applications in Python programming language. Diese Node -Klasse enthält 3 Variablen. Time = Distance / Speed. A common kind of tree is a binary tree, in which each node contains a reference to two other nodes (possibly None). Parses an XML section from a string constant. Each node of the binary tree has two pointers associated with it, one points to the left child, and the other points to the right child of the node. A tree is composed of nodes that store values and references to .
5 Best Ways to Implement a Binary Tree Data Structure in Python
Tree data structure is used to represent hierarchical data such as organization hierachy, .
A TreeNode is a data structure that represents one entry of a tree, which is composed of multiple of such nodes. Output: As you can see, In the above code, we have first created objects of the BinaryTreeNode class defined in the example.In this article, let’s first see how to implement a tree from scratch without using any library, and later you will see how to implement a tree with the help of a Python . Binary Tree is a form of a tree whose nodes cannot have more than two children.
Introduction to Abstract Syntax Trees in Python
Python Tree Data Structure Explained [Practical Examples]
How to traverse the tree in different ways. A path of a tree T is a a . A Binary Tree Data Structure is a hierarchical data structure in which each node has at most two children, referred to as the left child and the right child.
Learn Advanced Data Structures with Python: Trees
See examples of tree representation and traversal in C++, Java, and Python. We declared 3 functions to calculate speed, distance, and time.
Implémenter une structure de données arborescente en Python
Inorder Tree Traversal in Python. Parameters: criterion{“gini”, “entropy”, “log_loss”}, default=”gini” The function to measure the quality of a split.In Python, various types of trees exist, with each type having its own characteristics and use cases. The figure below shows what a Binary Tree looks like.Now, to answer the OP's question, I am including a full implementation of a Binary Tree in Python. Install the Graphviz Package. An edge of a tree T is a pair of nodes X and Y where X is the parent of Y or vice versa. They’re called the left and right children of the node. Likewise, each node can have an arbitrary number of child nodes.ensemble import RandomForestRegressor # Instantiate model with 1000 decision trees rf = RandomForestRegressor(n_estimators = 1000, random_state = 42) # Train the model on . A tree can be seen as a piecewise constant approximation. It generates a tree representation of a program’s code, which can help identify potential issues and improve code quality and security. Then, we will edit the “__str__” function to print the node values as if they were a tree. If not given, the standard XMLParser parser is used. Returns an Element instance. # Import the model we are using from sklearn. The goal is to create a model that predicts the value of a .The following Python code loads in the csv data and displays the structure of the data: . Read more in the User Guide. Visit the root node (usually an action is performed such as printing the node . To create a basic tree in python, we first initialize the “TreeNode” class with the data items and a list called “children”. Inorder tree traversal is basically traversing the left, root, and right node of each sub-tree. 236K views 3 years ago Data Structures And Algorithms In Python. A tree is a unique data structure that draws inspiration from natural trees, with nodes, edges, and leaf nodes.Binary trees starts with a root node, which is the topmost node, and the other nodes branch of from this node in a hierarchical manner. Parameters: criterion{“gini”, “entropy”, “log_loss”}, default=”gini”.Tree nomenclature. Mark as Completed. Application of depth-first tree . How to find the maximum value in a tree. This means there’s a direct link between the the two nodes.There are three types of tree traversal techniques: Inorder Traversal in Tree.Trees — How to Think Like a Computer Scientist: Learning with Python 3. Speed = Distance / Time.Arbre en Python - Python - Developpezdeveloppez.Decision Trees (DTs) are a non-parametric supervised learning method used for classification and regression.In this tree traversal method, we first visit the left sub-tree, then the root, and finally the right sub-tree. How to insert into a binary tree. The underlying data structure storing each BinaryTreeNode is a dictionary, given it offers optimal O(1) lookups.
Trees are non-linear data structures where there is a single root node and one or more sub-trees attached to it. Supported criteria are “gini” for the Gini impurity and “log_loss” and “entropy” both for the Shannon information gain, see Mathematical .
Tree Data Structure
This process continues until a base case is reached, which is .PyCF_ONLY_AST as a flag to .
00:00 In the previous lesson, I showed you .
The abstract syntax itself might change with each Python release; this module helps to find out programmatically what the current grammar looks like. Decision Trees ¶.
text is a string containing XML data. Python doesn't include a BST class by default—but allows developers to implement a custom one with ease! When considering data structures and algorithms one inevitably comes across binary search trees.The ast module helps Python applications to process trees of the Python abstract syntax grammar. Convert a Tree to a Dot File.Tree Traversal.Binary search trees are powerful data structures that can make searching, sorting, and maintaining data a breeze. It isn't the same as a binary tree, they're different . Recursion in Python Christopher Trudeau 07:06.A decision tree classifier. The topmost node of a tree is called the “root”, and each node (with the exception of the root node) is associated with one parent node. Let’s look at in-order traversal in action. Here is an illustration of a binary tree: Here node 1 is known as . Each node contains a data field that stores the object or value being stored.A binary tree is a special variant of a tree data structure. How to find the depth of a tree.Trees in Python. The advantages of decision trees include that we can use them for both classification and regression, that they don’t require feature . Here’s a basic implementation of a tree node:pythonCopy code.In Python, recursion is implemented by defining a function that makes a call to itself within its definition. An introduction to binary trees and different types of binary trees.
Tree Traversal Techniques in Python
You will learn how to construct, traverse, and . Supporting Material.Edges and Paths.Creating binary trees in Python.Continue your Python 3 learning journey with Learn Advanced Data Structures with Python: Trees.
Tree/Binary Tree in Python
Learn how to implement a TreeNode class in Python, which represents one entry of a tree data structure. Note: These traversal in .Last Updated : 22 Feb, 2024. Each child is an instance of the same class.
Tree Traversal
Now we will try to implement the binary tree given in the above example in the following code: def __init__(self, data): self. Convert a Dot File to an Image.Introduction to Tree Data Structure in Python
How to Implement a Tree Data Structure in Python
In-order Traversal. Post-order Traversal.Creation of Basic Tree in Python. Cette classe Node contiendra 3 variables; la première est la .Pour créer un arbre en Python, il faut d’abord créer une classe Node qui représentera un seul nœud. Preorder Traversal in Tree. This tutorial covers the concepts of binary tree, binary . Like list nodes, tree nodes also contain cargo.leftChild = None.Learn the basics of tree data structure, its terminologies, types, and operations. This is a general n-nary tree. In the Python example below, we do the following: Line 5-9: . Additional: Using To-Do List with . A binary tree is a tree in which each node has between 0-2 children.Hence we will use the abs () method to calculate the exact time, distance, and speed.Visualize Trees in Python. What binary trees are and what they are good for. Like linked lists, trees are made up of nodes. Earthly Cloud: Consistent, Fast Builds, Any CI. Pre-order Traversal.Python TreeNode class. Trees¶ Like linked lists, trees are made up of nodes. Tree Modification Methods. These are very common operations performed on trees.A generic tree is a node with zero or more children, each one a proper (tree) node. For many different reasons, decision trees are a common supervised learning technique.Einen Baum von Grund auf in Python implementieren. It is an acyclic connected graph, without any circuits. Let's explore some commonly used trees: Binary Trees. Formula used: Distance = Speed * Time. Trees do not contain self-loops, . Tree Search Methods. Constructing Trees. Each node in a binary tree has at most two children: the left child and the right child. In this article, we explore five methods for implementing binary trees in Python.rightChild = None. Exporting Trees. How to build them from scratch in Python. An abstract syntax tree can be generated by passing ast.Understanding Trees: Before delving into Python code, let’s establish a clear understanding of what trees are.💡 Problem Formulation: Binary trees are fundamental data structures in computer science used to represent hierarchical data.
An Introduction to Binary Trees in Python
In Python, trees can be implemented using classes and objects.comRecommandé pour vous en fonction de ce qui est populaire • Avis
Getting Started with Trees in Python: A Beginner’s Guide
What a balanced tree is, and how to balance one that is unbalanced.XML(text, parser=None) ¶.
How can I implement a tree in Python?
The BinaryTreeNode class would have a constructor function that takes in a .The first step in building a binary tree is to create a node object, which represents a single item in the tree. class TreeNode: .
Understanding Trees in Python
Decision Trees (DTs) are a non-parametric supervised learning method used for classification and regression. Depth-first traversal. Tree Traversal Algorithms.