Golang enum example
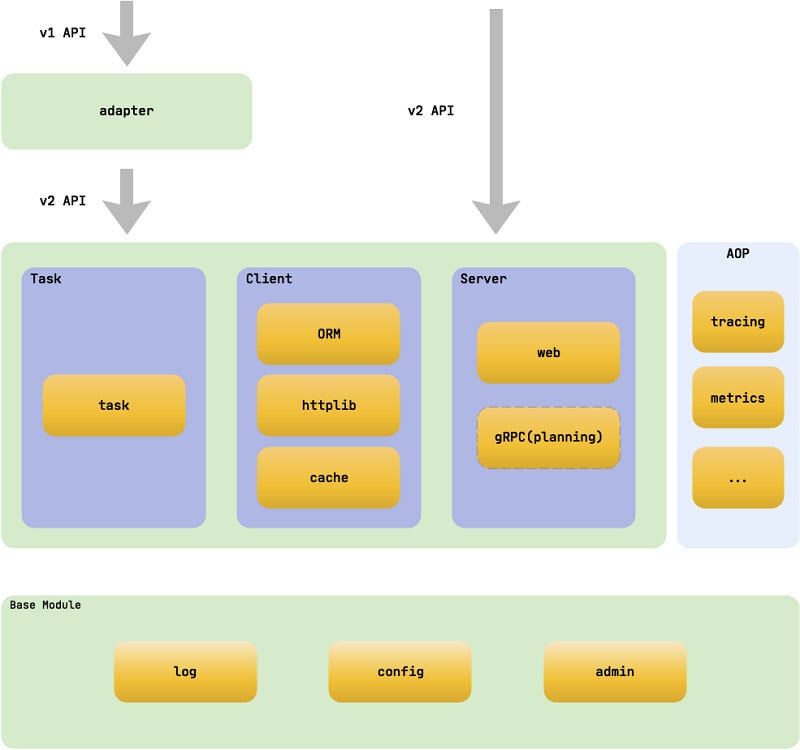
Convert an IOTA or Enum to a string in Go (Golang)
type USState int.In Go, enums can be created using constant blocks ( const) combined with the iota keyword.
4 iota enum examples · YourBasic Go
This article shows how to build enum like logic in Go using the iota keyword.
enums package
proto file syntax and how to generate data access classes from your . type DayOfWeek int. import fmt const (. I figured out something that works in a similar way (at least works for my current situation): Use string for enum -like constants: type MyEnum string. It is mainly used to assign names to integral constants, the names make a program easy to read and maintain.使用Go实现枚举? 枚举基本实现. 如果这个枚举有 自定义方法 ,可以 . Enum Operations.
How To Work With Golang Enum
An enum, or enumerator, is a data type consisting of a set of named constant values.A basic Go programmers introduction to working with protocol buffers.In this example, we've created a new type Weekday as an integer.
Working with enums in Go
IOTA in Go (Golang) In this post, we will see how to convert an IOTA or enum to a string value.
Ultimate Visual Guide to Go Enums and iota
T-shirt Sizes: Small, Medium, Large. For example, the days of the week: >>>. and generate a .When a repeated Enum field is parsed all unknown values will be placed in the unknown field set.This guide describes how to use the protocol buffer language to structure your protocol buffer data, including . Working with Golang Enums. The first step in creating enums is to declare them using the const keyword, followed by the enumeration values. Having a wide range of usages, ENUMs are a powerful feature .
Comparison Operations.An Enum (short for enumerated type) is an excellent choice to define suits, and in fact – the Wikipedia article about enumerated types uses card suits as an . Server Statuses: Unknown, Running, Stopped, Resumed. You can define a set of named constants with iota, which is a pre-declared identifier that represents successive integer constants.3 Enum definition .Critiques : 1
Master Golang Enum Techniques: Don't be a Rookie
Go is an open source programming language designed for building simple, fast, and reliable software.
They are most useful when you have a variable that can take one of a limited selection of values.proto file: enum Enum {A = 0; B = 1;} message Msg {repeated Enum r = 1;} A wire format containing the values [0, 2, 1, 2] for . Also, the enum values you list in the protobuf files will be generated to be constants in Go. Male Gender = 男性.An Enum is a set of symbolic names bound to unique values. Unfortunately, enums in Go aren’t as useful due to Go’s implementation. 可以使用 类型别名 ,让常量看起来 更直观 :根据类型就能明确知道该常量是啥枚举。. And you cannot take the address of constant values, for . Example of an . ) Now, use the decoding json to custom types as mentioned here. Enums are user-defined data types that allow us to restrict the set of values to a predefined list of named constants.
There are seven days of the week and not more. AFAIK, no you can't do that without explicitly typing the name as a string.Enum extracted from open source projects.For example, Python has Enum type: from enum import Enum.AFAIK, no you can't do that without explicitly typing the name as a string.
iota enums in Go
You can't assign a non-pointer Foo_Bar value to this field, only a pointer value. Enums are a powerful feature with a wide range of uses. the output of the stringer tool. An “enum” (or enumeration data type) is a data type that consists of a set of “values that are explicitly defined by the programmer”[@institute1990ieee]. data := MyJsonStruct{} Check out the first example or browse the full list below. Taking the example of days of the week, my code would have loops like for i := Monday; i < LastDay; i++ {. Acetaminophen = Paracetamol. However, in Golang, they’re implemented quite differently than most other programming languages. Hereby mistake, the state of wed is 2, it should be 3.这种方法和方法二中的方式具有相同的优点,同时该方法还能让通过gorm创建数据表时,让数据表的字段也是enum类型的优点。 结论.Protobuf with syntax=proto2 generates enum fields with pointer type, so Foo. Although it borrows ideas from existing languages, it has unusual properties that make effective Go programs different in character from programs written in its relatives.Try it out! This tutorial provides a basic Go programmer’s introduction to working with gRPC. You can even define message types nested inside other messages – as you can see, the PhoneNumber type is defined inside Person.
I will show you how to implement enumerators, or enums, in Golang using a predeclared identified iota.
Emulating Enumerations in Go: Unlock the Power of Enums
Example enums: Timezones: EST, CST.
Declare enum in Go (Golang)
In the above example, the Person message contains PhoneNumber messages, while the AddressBook message contains Person messages.Enums provides utilities for creating and using enumerated types in Go. 先来实现基本的枚举功能,我们要实现以下几点: 通过 type 关键字给 int 类型起别名,达到自定义枚举类型的效果;; 通过 iota 关键字 + 自定义的类型赋值给常量,作为枚举项;; 实现 Index 方法返回枚举项的索引值,借助类型转换,将自定义类型转回 int 类型;
Protocol Buffer Basics: Go
One simple way to create enumerations in Go is by using constants. Then, we've defined a constant block with the days of the week as enum-like values, using iota to increment their integer values automatically. Alabama USState = iota. // Enum of week days.Enums (short of enumerators), identifiers that behave like constants, are useful parts of many languages. Why do we need enums? Grouping and expecting only some .
Go: How to use enum as a type?
An enum is a data type consisting of a set of named constant . take a look at this example. const( Monday DayOfWeek = iota. For example, days of the week are an enum.
Implementing Enums in Golang
Then you may use it like Color. Arbitrary Expressions. Please refer to the same example below for a better understanding.Using Constants. They let you define strict values of data you expect. const ( statusPending = PENDING statusActive = ACTIVE ) Or, application of the example at Ultimate Visual Guide to Go Enums. 通过类型别名可以很直观、很明确地知道 Male 和 Female 两个常量是性别枚举。. They are similar to global variables, but they offer a more useful repr() , grouping, type-safety, and a few other features. import fmt type Size uint8 const ( . Conversion and Parsing.Go is a new language. It covers the proto3 version of the protocol buffers language: for information on the proto2 syntax, see the Proto2 Language Guide. There are two main parts of enums: command enumgen and package enums.
How to use enums in go?
This is better than the top answer since if you have a ton of enums, you don't have to write out the list twice (unlike top answer): package usstates.This is a common pattern in . It's a good practice to name your enums in a way that clearly represents their purpose.Golang Enum - 3 examples found. For example, given the .Enumeration (or enum) is a user defined data type in C. Enforces compile-time type-safety resulting in more robust code. Skipping Values. Use the Go gRPC API to write a simple client and server for your service. // and here will be an alias of unit8.To create enum in Golang you need to declare custom type, constant (const) values using iota keyword and some helper methods: String() and IsValid()
How and Why to Write Enums in Go
//go:generate stringer -type=USState. 在本文中,我们探讨了如何在Go中给GORM模型添加枚举类型。枚举是一个有用的功能,它允许我们定义变量可以取的一 .comGitHub - eddieowens/go-enum: Enums for Golanggithub. This is a reference guide – for a . Generate server and client code using the protocol buffer compiler. A straightforward translation of a C++ or Java program into Go is unlikely to produce a satisfactory result—Java programs are written in Java, not Go. enum State {Working = 1, Failed = 0};All you have to do is to implement String method on the type that defines the ENUM. iota is a predeclared identifier representing successive untyped integer constants. Here's a basic syntax and example: But you can use the stringer tool from the standard tools . Please read the official documentation to learn a bit about Go code, tools packages, and modules.
学习gorm系列九:gorm中如何使用枚举值
A common practice in go is to use the suggestion posted by @ttrasn : define a custom type, and typed constants with your enum values : NEW_USER EventName = NEW_USER. When it is serialized those unknown values will be written again, but not in their original place in the list. In Golang, we use a predeclared identifier, iota, and the enums are not strictly typed. running this command.Get Free Course.What are common practice in golang to iterate over constant values used as enum.Having a wide range of usages, ENUMs are a powerful feature of many languages.For enums that I need to be iterable, I usually add a dummy end marker. See this example.Package enum simplifies the creation of enumerated types (which Go does not natively support). When dealing with enums .Enum Declaration; Utilizing Enums In Functions; Enum Declaration. Let’s see an example. go-enum will take a commented type declaration like this: // ENUM(jpeg, jpg, png, tiff, gif) type ImageType int.That way, if hell freezes over and I need to add an 8th day to the week, I just have to shove it before LastDay, the dummy end marker. The biggest drawback is that they aren’t strictly typed, thus you have to manually validate them.baz will be of type *Foo_Bar. As an example, having . type Day uint8. IOTA provides an automated way to create an enum in Golang.
There is no enum type at the moment in go, and there currently isn't a direct way to enforce the same rules as what typescript does.
Later we will see an example of we can define a custom toString method to print the string value of IOTA or enum. By walking through this example you’ll learn how to: Define a service in a .
golang protobuf enum example
It resets to 0 whenever a new const block is encountered and increments by 1 for each constant within the block.Iota basic example; Start from one; Skip value; Complete enum type with strings; Naming convention; Iota basic example. Now that we've defined our Golang enum, let's discuss how to work with it effectively. These are the top rated real world Golang examples of github.
The Complete Guide to Enumerations in Go
Unlike many other programming languages, Go has no build-in enum type. class Color(Enum): RED = 1.