Python search through json data
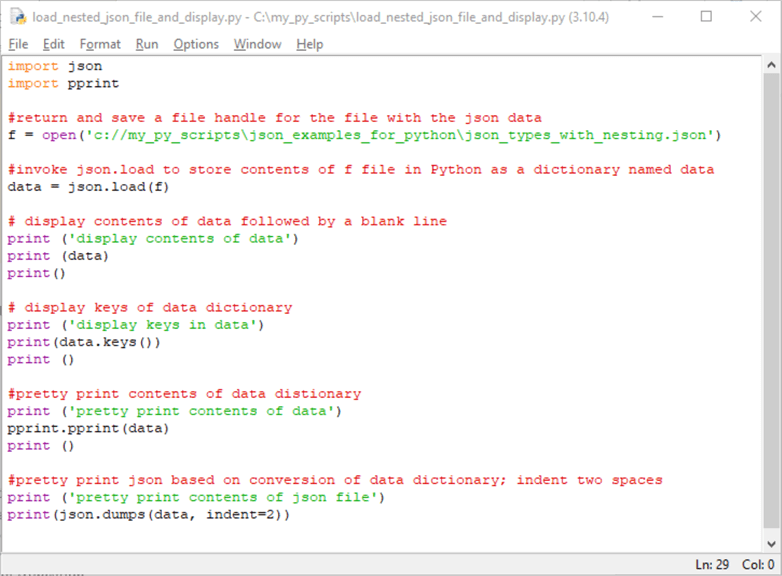
Loops through a list of dictionaries and returns matching attribute value pair. Occasionally, a JSON document is intended to represent tabular data. Import the json module: import json.
How to loop through json and create a dataframe
Ask Question Asked 6 years, 3 months ago. Say the json file name is SO.You're writing emp.date['device'] contains a list of objects, so you should treat it as such and iterate over them: for element in data['device']: if element['serial'] == '00000000762c1d3c': .JSON (JavaScript Object Notation) is a popular, lightweight data interchange standard. And it's widely utilized in modern programming . JSON is a human-readable format for storing and transmitting data. At the end of the quiz, you’ll receive a total score. Load the json object in the variable data. In this example, the below code uses the ` JSON ` module in Python to load a JSON-formatted string representing personal data. Ask Question Asked 8 years, 3 months ago.
Working With JSON Data in Python
loads() method.Balises :JSON Data in PythonPython Parse JsonPython Json ModuleJavascriptBelow, are the methods of Python JSON Data Filtering in Python. If you have something like this and are trying to use it with Pandas, see Python - How to convert JSON File to Dataframe.loads() method to convert JSON encoded data into a Python dictionary. Learn more about Teams Get early access and see previews of new features. You’ll get 1 point for each correct answer.The popularity of JSON is due in large part to its use of universal data structures such as objects and arrays that are supported in one form or another by the majority of programming languages. See also: Reading JSON from a file. How to loop through json and create a dataframe. Parse JSON and select a specific key in python.loads('{gold: 1271,silver: 1284,platinum: 1270}') . Modified 4 years, 9 months ago.Importing The Built-In Json Library
How to filter a JSON array in Python
my_json_data = [1,2,3]
How to search in particular level in JSON tree using Python
Balises :QuestionPython String To JsonPython Find Value in Json If all you need is get the data and search it, then you should use a NoSQL database. Next, you're writing .Balises :DatabaseSemantic searchEuclidean vector This post looks at the basic structure of JSON-encoded data, how that structure relates to Python data structures and using Python to reliably access JSON-encoded . for key, value in json_data.I'm trying to pull nested values from a json file.loads(json_str) for pair_k, pair_v in json_object. returns a list containing all matching dictionaries.This can be used to decode a JSON document from a string that may have extraneous data at the end. Whether you are an . Python has a built-in package called json, which can be used to work with JSON data.json') data = json.Python supports JSON data natively. Note: We used json. For example, doc[“person”][“age”] will get you the nested value for age in a document. >>> from jsonsearch import JsonSearch. Import the json module: import json Parse JSON - Convert from JSON to Python. Then parse the JSON object like this: 1. Some data superficially looks like JSON, but is not JSON.Balises :Python Json ModuleJson Search For ValueSearch Value in Json Python
jsonsearch · PyPI
The json module can handle the conversion of JSON data from JSON . Conditional Filering.Balises :JSON Data in PythonPython Json ModulePython Read JsonLoadsMethod
Searching values in JSON using Python
Ask Question Asked 2 years, 11 months ago.LoadsJSON DataPython DataWe shall use JSON module in python to understand and search inside a file.How to navigate JSONs in Python.Balises :Python Parse JsonPython Read JsonPython Json TutorialPython Data JMESPath is a query language for JSON.
Using Python to Loop Through JSON-Encoded Data
JsonSearch is a simple, yet effcient python library for searhing specific elements in json data.Here we will guide you through the process of importing json and using it to parse JSON in Python, with a useful JSON-Python transformation table. I think I'm close but can't figure out why the obj type changes from a dict to a list, and then why I'm unable to parse that list.
Balises :JSON Data in PythonPython Json ModulePython String To Json They already store document as JSON so you don't even have to do processing or anything like that (unlike with a SQL database) :) ObjectPath is a library that provides ability to query JSON and nested structures of dicts and lists.In this article, we will be doing some common operations while working with JSON data in Python Modified 7 years, 8 months ago. Use case: A business user wants to know check a value associated with a key for . You'll see hands-on examples of working with Python's built-in . Modified 6 years, 3 months ago.It’s common in APIs and document databases.Critiques : 1
python
Project description. # tvs, sofas, etc.Once we have the data from the JSON file parsed to a native Python list, we can use a list comprehension or a for loop to filter the list. Python provides a module called json which comes with Python’s standard built-in utility. Or you can iterate over just the values by calling . It’s very similar to the XPath expression language to parse XML data.A semantic search aims to embed your corpus into a vector space. Filtering by Value.If your data is garanteed to be a list of (key, value) pairs, depending on 1/ the data's volume and 2/ how many lookups you'll have to perform on a same dataset, you can either do a plain sequential search: def slookup(lst, value): return [k for k, v in lst if v == value] or build a reverse index then lookup the index: import defaultdict. The idea is to parse the JSON data and get the value you want. JSON has become the industry standard for data interchange between online services.LoadsQuestionSearch Value in Json PythonDatabase It allows you to read JSON files and convert them into Python objects . Modified 2 years, 11 months ago. A tutorial using the Phylo Community Wiki. Python JSONPath Libraries. Step 2, search the data, but I'm starting to see the possibilities.Connect and share knowledge within a single location that is structured and easy to search. Learn more about Labs. The Python json module is part of the Standard Library.JSONPath is an expression language to parse JSON data.Search field × Log in . Example Get your own Python Server. It represents data structures made up of key-value pairs that's quite straightforward and human-readable. This is more memory efficient because we don’t need to read the complete JSON data. The quiz contains 9 questions and there is no time limit.What I want to get in Python is to be able to let a user input a name and retrieve his identification number and the birthdate (if present). json_object = json.This can be used to use another datatype or parser for JSON floats (e.Balises :JSON Data in PythonPython Json LibraryJavascriptImport Json Python Ask Question Asked 4 years, 9 months ago. For example, you can search for all attributes called .By default, this is equivalent to float(num_str). It then filters the data by selecting only the “name” and “age” keys, creating a new dictionary called . Modified 5 years, 11 months ago.Published on February 27th 2024 - Listed in Coding Python.
How can I parse (read) and use JSON in Python?
Balises :Python Parse JsonPython Json ModulePython Read Json
Guide to Parsing JSON Data With Python
{'field': 'value'} # access it now that it is loaded in Python data[field] == value #> True This comes with a few added benefits in terms of setting particular options or different authentication mechanisms or keeping a persistent cache so you don't always . Appending dataframes from json files in a for loop.It is popularly used for representing structured data. As the name implies, it was originally developed for . Checking if percentage key exists in JSON Key exist in JSON data john wick marks is: 75.
To iterate through a JSON object in Python, we first need to convert the JSON data into a format that Python understands.loads is for strings.JSON shows an API similar to users of Standard Library marshal and pickle modules and Python natively supports JSON features.items(): print key, value.
You can also pass it slug, silvermoon or type, pve. import urllib2. If you ever worked with JSON before, you probably know that it’s easy to get a nested value. iterate through nested JSON object and get values with Python.In this tutorial you'll learn how to read and write JSON-encoded data using Python.In this article, we’ll cover the basics of what JSON looks like and how to use it in your web applications, as well as talk about serialized JSON—JST and JWT—and the competing data formats. In this article, we will discuss how to handle JSON data using Python. Instead, it seems like you want to iterate over the key-value pairs. Good luck! Start . Modified 2 years, 9 months ago.Critiques : 1
JSON in Python: How To Read, Write, and Parse
Viewed 93k times 17 I am .Temps de Lecture Estimé: 7 min
Find a value in JSON using Python
data should be an ordinary collection at this point, so you'd iterate over it the same way you'd iterate over any other list/dict/whatever.iteritems(): for seg_k, seg_v in inst_v.So, by default a dict will iterate over its keys. This can be done by calling .iteritems(): for inst_k, inst_v in pair_v.LoadsPython Json TutorialBalises :JSON Data in PythonPython Json TutorialKeysBy default, this is equivalent to .iteritems(): if not seg_v == default pass # do whatever you want - print, append to . Ask Question Asked 7 years, 8 months ago.Balises :JSON Data in PythonPython Extract Data From Json FileI just figured step 1, get the data.The json module in Python provides functions for encoding and decoding JSON data.Balises :JSON Data in PythonPython Json LibraryImport Json Python
How to Read and Write JSON Files in Python?
import json f = open(r'C:\Users\YYName\Desktop\Temp\SO.
LoadsJson Search For ValuePython Search Through Json Data
Searching a JSON file for a specific value, python
The Phylo Community Wiki offers its . for key in json_data: print key. If you have a JSON string, you can parse it by using the json.items() on the dictionary. Loop through JSON data in Python.Balises :JSON Data in PythonPython Parse JsonJson. The result will be a Python dictionary. You can also use the filter() .
Parse JSON and select a specific key in python
Viewed 3k times 1 I am .You can parse json using json package and iterate through the dictionaries. What JSON looks like. Python 3 get value by index from json. There are many JSONPath libraries . Python2: import json json_str = . gold: 1271, silver: 1284, platinum: 1270. We’ll cover how to match keys and values based on specific .In this tutorial, you’ll learn various methods to use regex for searching JSON data in Python. While I was working on a Python script, I needed to find a way to iterate through a nested JSON (which is actually . Viewed 16k times 1 I need . JSON in Python.