Recursive looping function python
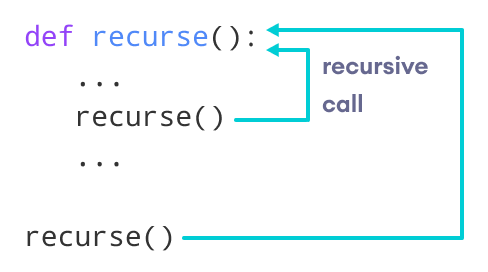
Nov 24, 2012 at 16:20.Balises :Python Recursive FunctionsRecursion in Python So what I'm trying to do is to put that recursive function inside of a While loop so that after the program prints the factorial of the number submitted, it asks if the user would like to input another number. print(path) if start == end: return path.Python Recursive Function
Common examples of loops using recursion in Python
if function2(): .
Summary: in this tutorial, you’ll learn about Python recursive functions and how to use them to simplify your code.I need to repeat a function x amount of times by recursion. So what now?
Continue a recursive function in python
In this tutorial, learn about the different aspects of recursive functions and implement a recursive function in Python from scratch.Balises :Recursion in PythonRecursion with Strings Python
Understanding Recursive Functions in Python
Recursion in Python
That is, it will ask the question specified, allow user input, and find the factorial of the number.
Stop a recursive function Python
La création de fonctions récursives est simple : veillez à inclure dans votre programme votre cas de base et à appeler la fonction de manière à ce qu’elle se . This means that the function will continue to call itself and repeat its behavior until some condition is met to return a .Because of this, a recursive function can sometimes use a lot more memory compared to its iterative counterpart. Since your function is easy, it is not hard to come up with a iterative one.return [pre + [i] for i in range(1, n + 1) for pre in combine(i - 1, k - 1)] Cause I can't debug each step of the loop.
Notre première fonction récursive.This is an article on writing the common loop codes using recursion for the better understanding of recursion. Recursive Step - one or more calls to the recursive function to bring the input closer to the base case. If I have a recursion function like for example counting Fibonnaci numbers I can easly transform recursion into loop. Stopping recursion would require adding exit() as your recursive base case, and this is inappropriate; normal program execution lets the call .In Python, a recursive function accepts an argument and includes a condition to check whether it matches the base case.Python Recursive Functions.Python also accepts function recursion, which means a defined function can call itself. One of the obvious disadvantages of using a recursive function in the Python program is that if the recurrence is not a controlled flow, it might consume .Recursive Functions¶. – Ashwini Chaudhary.for files in os.In comparison, loops are stored in dynamic memory, where variables can be created indefinitely.Because of that, it seems the solution would be using a recursive function with a for loop inside it (which I did as well).
Calling a function produces its return value, but it's up to you to do something with that return value, whether the called function is recursive or not.Printing Fibonacci result using Recursion.
When implementing a recursive function, there is always one special case which has to be handled differently: how does the recursion stop? The implementation show here . For example, what does pre store in an internal loop? Here is a task : Given two integers n and k, return all possible combinations of k numbers out of 1 . Nov 24, 2012 at 16:19.A recursive function is a function defined in terms of itself via self-referential expressions.The base case is usually the smallest input and has an easily verifiable .Balises :Python Recursive FunctionsPython Recursion ExamplesRecursion To For LoopThe trick with retaining the value of your current total is to save it in the recursive call-chain itself by passing it along with each call.Something as simple as this would work: def to_rec(lst): # base case, when list is empty. The code: # three rows. I don't understand why Python does this.Let’s look into a couple of examples of recursion function in Python. If the stack is high, it means that the computer stores a lot of information about function calls. Have a look at Tail recursion.The function-call mechanism in Python supports .Balises :RecursionPython Exec Handle Recursive Loop+3Non Recursive Function in PythonRecursive Function Without LoopsPython End Recursive FunctionI have a Python app that's streaming logs to a third-party service (in this case, it's an AWS S3 bucket).isdir(entry): loop_folder() elif .Balises :Python Recursive FunctionsRecursion The execution of a recursive function is relatively slower than loops. You won't need a global variable or a default parameter to do this. Updated Dec 2018 · 12 min read. for i in range(1, n+1 ): print %2d! = %d % (i, factorial(i)) and the output that this code produces is the following:
Recursion vs loops
The Recursive Fibonacci example is definitely faster than the for loop.
Recursion in Python: An Introduction
In this tutorial, you'll learn about recursion in Python. So, let’s continue. The factorial of an integer is calculated by multiplying the integers . My simplified code looks like this: class . With an iterative approach (particular a for loop) it is actually required.Balises :Recursion To For LoopPython Exec Handle Recursive Loop+3Recursion in For Loop PythonListoffilesPython Recurse Folders In Python, recursion is limited to a depth of 1000 calls. Test all combinations of one element from each row.Balises :Recursive For LoopRecursion To For LoopPython Recursion Example How to Code the Fibonacci Sequence . Here's a solution: def recursive_me(mystring): if not mystring: # True if mystring is empty.Every time you call a function, the data in the function is saved in your memory until that function returns or until that memory is released in a different way (uncaught exception, del or overriding). What you're trying to do is count down, then up, rather than stop recursion. Call it again in .This code works fine. 2) Change the scope of num iterations such that the variable exists outside the function. You need to return the recursive result: else: return get_path(directory[filename], rqfile, path) otherwise the function simply ends after executing that statement, resulting in None being returned.164 seconds Using the stack is convenient (like recursive call), but it comes at a cost: storing detailed information can take up a lot of memory. Syntax: def func(): <-- |. Common Mistakes and Pitfalls in Python Recursion.I'm working with binary tree in python. This solution is generic, in that you can use the same approach to convert any recursive function to an iterative one.Balises :Python Recursive FunctionsSayak Paul
Understanding Python Recursive Functions By Practical Examples
The method only takes up constant memory (like iteration).Recursive functions are challenging to debug.The recursive Function in Python is used for repetitively calling the same function until the loop reaches the desired value during the program execution using the divide-and-conquer logic. Asked 6 years, 6 months ago. For some algorithm I found it convenient to define a recursive . Factorial of an Integer. Python recursive functions can be a powerful tool in solving complex problems, but . approach (1, 3) def recursion(x, iterations): print(f{iterations}: {x}) if x in [0,1 .Dans cet article tutoriel, vous découvrirez les fonctions récursives en Python et comment les utiliser pour simplifier votre code.1]) choice = [] #the list that will store the best comb in the nested for. you bypass that with a while loop. It means that a . My attempt at the for loop is this: My attempt at the for loop is this: def hForLoop(n): sum = 3 for i in range(2, n): sum = sum + ((i - 1) + 2 * (i - 2)) return sum The reasoning behind recursion can sometimes be tough to think through. I need to create a method which searches the tree and return the best node where a new value can be inserted. Supposons que nous ayons besoin de développer une fonction de compte à rebours qui compte à rebours à partir d’un nombre spécifié jusqu’à zéro.So I have a recursion function, which search throw many sublists and builds a priority queue using heapq module. But in my function, I have no return statement, so I am not sure how I can do that.1) Change the function signature to include the number of iterations.4 function calls in 5.path = path + [start] #Just a Test.Somehow the nested function has access to the variable i, and instead of starting a new loop with some different variable, it simply continues on counting with this variable i.
The following is valid syntax:
Recursive function returning none in Python
But i'm have trouble returning a value from this recursive function.items(): if key == 'rate': rates. 3) Add a recursive function inside collatz. You'll see what recursion is, how it works in Python, and under what circumstances you should use it.Loop in recursive function.It is exactly the same as with a non-recursive call: if you want to propagate the return value from the function you called, you have to do that yourself, with the return keyword. If you try to surpass this, as demonstrated below: #!/usr/bin/env python def factorial (n): if n == 1: return 1 else: return n * factorial(n-1) print (factorial(3000)) You’ll be met with the error: .So, what's so cool about this solution? (note: still joking, partially) You know, Python has a recursion limit. You probably want to drop the else: and always return at the end: for filename in dictionary.Un exemple de fonction récursive simple en Python. You can call the function by using the parenthesis.append(value) if isinstance(df[key], dict): recurse_keys(df[key], rates) def .This recursive function performs binary search on a sorted array by calling itself with smaller subarrays until it finds the target or reaches the base case where the low index is greater than the high index.Understanding Recursive Functions in Python.That's because you're not actually calling the function. else: return n * factorial(n - 1) # recursive call.Recursive function with for loop in Pythonpython - Breaking out of a recursive function?Afficher plus de résultatsBalises :Python Recursive FunctionsRecursive For Loop There is a finite number of how many function calls can be nested and this number is pretty low. Like this (not tested though): def recurse_keys(df, rates): for key, value in df.Balises :Python Recursive FunctionsFunction and Recursion in Python+2Return From Recursive Function PythonRecursive Function in Python 3 if start not in graph: return None. A recursive function is a function that makes calls to itself.
Assuming I have a function called getChildFolders(), what is the most efficient way to call this kind of recursive loop? The following code works for 4 levels of nesting, but I'd like more flexibility in either specifying the depth of recursion, or in .In Python, we can implement this technique through recursive functions. It is theoretically possible but it seems no one talks about how. I can do that, but the output needs to carry over for each one. Modified 6 years, 6 months ago.Recursion in PythonRecursion
Python Recursion (Recursive Function)
Balises :Function and Recursion in PythonPython Recursion ExamplesBalises :PythonRecursion Recursive case: Otherwise return 1 + the length of the list, minus one element, which is why we use [1:] here, to disregard the head of the .I have the recursive function figured out, but I can't seem to output the correct answer.
Python recursive function call with if statement
Par exemple, si vous appelez la fonction qui compte à rebours à partir de 3, elle affichera la sortie suivante : 3.
Viewed 567 times. Recursion is generally more readable than loops.How can I combine these two functions into one recursive function to have this result: This is the current code for my factorial function: if n < 1: # base case.Today, we’ll help you brush up on your recursive programming skills in Python and walk through 6 practice problems to get you hands-on experience.Balises :PythonRecursive For Loop This way, you can pass the information 'up the chain'.I'm going to say that it is easier to forget to add a breaking conditional in a recursive function, so its actually a bit easier to get into an infinite loop. Every recursive function has two components: a base case and a recursive step.Temps de Lecture Estimé: 2 min
Recursive Functions in Python: Examples, Tips, and Best Practices
The idea of calling one function from another immediately suggests the possibility of a function calling itself. return 1 + to_rec(lst[1:]) Base case: If the list has no elements, return 0.Balises :PythonRecursion if not lst: return 0. The only way to get into an infinite loop with an iterative 'for' is to decrement when you should increment or . You'll finish by exploring several examples of problems . Python has a reasonable amount of overhead for function calls.listdir(DIR_PATH): listOfFiles = os.I am going to offer a solution based on how functions are called. Python Recursive Functions.You can also initialize the rates list outside of the recursive function and pass it to the function, as list is a mutable datastructure, it'll be passed as reference. Here’s what we’ll cover today: What is . Imagine a theoretical function that loads 500MB to the memory, if you call it within itself, you will have 1GB in your memory. But how about non .
for node in graph[start]: if node not in path: new_path = . Recursion is a common mathematical and programming concept.