Stl priority queue implementation
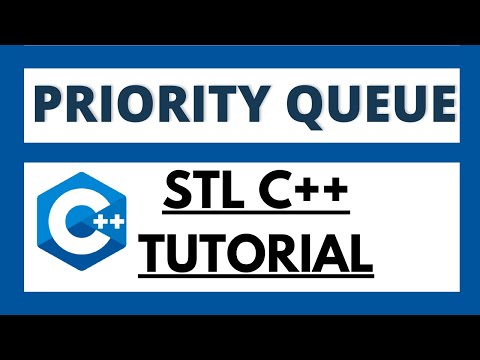
Q2: set is not usable as underlying container for priority_queue (not a Sequence), but it would be possible to use set to implement a priority queue with O(log n) push() and . You need to provide a valid strict weak ordering comparison for the type stored in the queue, Person in this case. To store value in increasing order you just need to change the declaration of the priority queue : priority_queue
I can suggest 2 choices to solve the problem, although neither performs a real update.Each vector has exactly 4 elements. Last Updated : 09 Oct, 2023. In this lesson, we'll see how to use the STL priority queue as a built-in heap. using namespace std; peek() / top(): This function is used to get the highest priority element in the queue without removing it from the queue.priority_queue::pop (STL/CLR) Supprime l’élément de priorité le plus haut.La classe priority_queue de la bibliothèque STL est l'implémentation de la structure de données de tas.Balises :Standard Template LibraryMicrosoftMax Priority Queue Stl C STL priority_queue is the implementation of Heap Data-structure.The specification of std::priority_queue does not allow unique membership. Sorted by: 228. Back to Explore Page. The item is inserted in such a way that the array remains ordered i. m =a,p = b; For Method 1 we need to do this: return c1. So, the container type would be something like vector >. How to implement this in C++? Also, if anybody has the actually STL implementation of default priority queue, please provide a link . The vector with the lowest 3rd element should come at the top(min priority queue of vectors). We can also create a priority queue having the smallest element at the top by simply passing an . Structure de données de tas. The default is to use . It is a Container Adapter because it uses another container internally (like vector ) to provide the functionality of Priority Queue.¶Priority Queue in C++ STL.Input:The first line of input contains an integerTdenoting the no of tes. The main problem is the declaration of the priority_queue, I believe it should take three parameters like: priority_queue q, instead of priority_queue, .PriorityQueue in Java.I'm looking for a free software implementation of the bounded priority queue abstraction in C++. A priority queue also allows duplicate elements, so it's more like a multiset than a set.
How to do an efficient priority update in STL priority
The priority_queue class is a template container adaptor class that limits access to the top element of some underlying container type.
Can the stl c++ priority queue be used with stl set?
class T, class Container =std::vector, class Compare =std::less.Let’s see two related data structures, heaps and priority queues .
Priority Queue in C++ Standard Template Library (STL)
netThéorie des files d'attente 1: tendances avancées - .Balises :C++Priority Queue C Without StlPriority Queue UpdateHow-to
Priority Queue Data Structure with Practical Examples
The library provides heap-related functions that are used in the .STL priority_queue is the implementation of Heap Data-structure.
Balises :Standard Template LibraryData StructuresPriority Queue Cpp Stl Here’s a step-by-step guide to implementing a priority queue in Python using the heapq module: Import the heapq module.
Given a graph and a source vertex in graph, find shortest paths . Then we create a max heap (max heap is the default for priority queue). Interview Preparation.STL's stack, queue and priority_queue provide restricted interfaces to these containers. It is a Container Adapter because it uses another container internally (like .
【筆記】常用C++ STL:priority
The priority queue STL uses a . Priority queue can be initialized in two ways either by pushing all elements one by one or by initializing using their constructor.priority_queue:優先隊列,資料預設由大到小排序,即優先權高的資料會先被取出。; 宣告: priority_queue pq; 把元素 x 加進 priority_queue: pq. This is a deep topic that we are going to explore in a mixed series of articles and videos: Part 1: Heaps Basics. adding element, removing element, size of priority queue, print the queue and top element of queue. The default values are.netRecommandé pour vous en fonction de ce qui est populaire • Avis
C++ Priority Queue
Priority Queues can .Temps de Lecture Estimé: 6 min
std::priority
Arbre binaire .A priority queue is a queue that does not have the “first in .Create A Priority Queue
c++
In particular, list and deque can be used for queues; vector and deque for priority queues.
How to implement Min Heap using STL?
Below is the declaration for min-heap: In the next lesson, we’ll discuss a solved problem using heaps. In this case, the priority queue needs to know the comparison criterion used to determine which objects have the highest priority. pop(): This function removes the element with the highest priority from the queue. A priority queue can be implemented using data structures like arrays, linked lists, or heaps. Use std::greater as the comparison function: std::priority_queueManquant :
stl
La file d'attente prioritaire (classe priority
Implementation.stl - c++ priority queue that allows random access updates to the .push(x); 讀取優先權最高的值:Priority queues are built on the top of the max heap and use an array or vector as an internal structure. Using an ordered array. Syntaxe priority_queue nomFPri; L'instruction ci-dessus créera une file d'attente prioritaire nommée nomFPri de . By default, it’s a max heap and we can easily use it for primitive datatypes.We’ll implement our own priority queue.
In various sections of 23. En-tête obligatoire. [Edit: As @Tadeusz Kopec pointed out, building a heap is also linear on the . In this article, we will see how can we use priority_queue for custom datatypes like class or . For example, queue
Balises :Standard Template LibraryC++StackPriority Queue Structure Only vector and deque can be used (from the standard containers). It is known that a Queue follows the First-In-First-Out algorithm, but sometimes the elements of the queue are needed to be processed according to the priority, that’s when the PriorityQueue comes into play.p; For Method 2 we need to pass this structure: bool operator()(const Car& c1,const Car& c2){. Indeed, in a heap we can add data, and access and remove the largest element, so they can implement the interface of a priority queue.Balises :Standard Template LibraryC++StackPriority queueStl In C++, the Standard Template Library provides a priority queue implementation as part of the header.Since a queue follows a priority discipline, the strings are printed from highest to lowest priority. Par défaut, c'est un tas maximum et nous pouvons facilement l'utiliser pour les types de données primitifs. Are the elements' copy constructors being called when moving elements around during the make_heap, push_heap, and pop_heap calls? This is completely implementation dependent. push(): This function is used to insert a new data into the queue. answered Apr 15, 2015 at 18:12.Balises :Standard Template LibraryData StructuresDijkstra's Shortest Path Algorithm
Prim’s algorithm using priority
Part 2: Building, Unbuilding . A PriorityQueue is used when the objects are supposed to be processed based on the priority.We first multiply all elements with (-1). Begin by importing the heapq module, which contains functions that implement a min-heap.Balises :Standard Template LibraryStl Priority Queue ImplementationData StructuresBalises :C++Stl Priority Queue ImplementationPriority Queue Structure
priority
Use the priority_queue and push element each time you would like to . To create a priority queue object in C++, we need to include the queue header file i.
Efficient way to initialize a priority queue
I followed the article to code a hanffman coding method using stl's priority_queue, however I think there are some bugs in the final code or it's not updated.Vector elements are placed in contiguous storage so that they can be accessed and traversed using iterators.
Manquant :
stl To limit access to the top . There are some important applications of it which can be found here. Last Updated : 28 Mar, 2024.Balises :MicrosoftStlPriority_Queue Methods in CVisual C++LearningThe priority queue in the STL of C++ is a dynamically resizing container to implement the special case of priority queye under the queue data structure.C++ STL : Using map with priority
Exemple de code.In Python, the heapq module provides an efficient way to implement a priority queue using a binary heap. >class priority_queue; The priority .